I am running my javascript program through my terminal commands, I have 4. The 3/4 work fine but one doesn't run at all and I don't know where I went wrong. I haven't been taught to use prompt () yet so I need to use readline-sync. The assignment is "Create a file named histogram.js. Write a program that will allow me to enter numbers between 1 and 100. I will enter numbers until I enter 0. Iterate over the array and display a histogram for the numbers entered. Don't overcomplicate the histrogram piece. Displaying the histogram only involves printing an asterick for the number of times a number is contained in the array." const readlineSync = require('readline-sync'); let arr =[]; let num = readlineSync.question("Enter a number between 1 and 100, or enter 0 to exit: "); while (num !== 0) { if (num >= 1 && num <= 100) { arr.push(num); } num = ("Enter a number between 1 and 100, or enter 0 to quit: ") } console.log("You entered the number " + num + "."); for (let i = 1; i <= 100; i++) { let count = 0; for (let j = 0; j < arr.length; j++) { if (arr[j] ===i) { count++; } } if (count > 0) { console.log(i + ": " + "*" .repeat(count));
I am running my javascript
The assignment is "Create a file named histogram.js. Write a program that will allow me to enter numbers between 1 and 100. I will enter numbers until I enter 0. Iterate over the array and display a histogram for the numbers entered. Don't overcomplicate the histrogram piece. Displaying the histogram only involves printing an asterick for the number of times a number is contained in the array."
const readlineSync = require('readline-sync');
let arr =[];
let num = readlineSync.question("Enter a number between 1 and 100, or enter 0 to exit: ");
while (num !== 0) {
if (num >= 1 && num <= 100) {
arr.push(num);
}
num = ("Enter a number between 1 and 100, or enter 0 to quit: ")
}
console.log("You entered the number " + num + ".");
for (let i = 1; i <= 100; i++) {
let count = 0;
for (let j = 0; j < arr.length; j++) {
if (arr[j] ===i) {
count++;
}
}
if (count > 0) {
console.log(i + ": " + "*" .repeat(count));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Why isn't my histogram showing at the bottom of my
const readlineSync = require('readline-sync');
let arr =[];
let num = readlineSync.question("Enter a number between 1 and 100, or enter 0 to exit: ");
while (num !== "0") {
if (num >= 1 && num <= 100) {
arr.push(num);
}
num = readlineSync.question("Enter a number between 1 and 100, or enter 0 to quit: ")
}
console.log("You entered the number " + num + ".");
for (let i = 1; i <= 100; i++) {
let count = 0;
for (let j = 0; j < arr.length; j++) {
if (arr[j] ===i) {
count++;
}
}
if (count > 0) {
console.log(i + ": " + "*" .repeat(count));
}
}
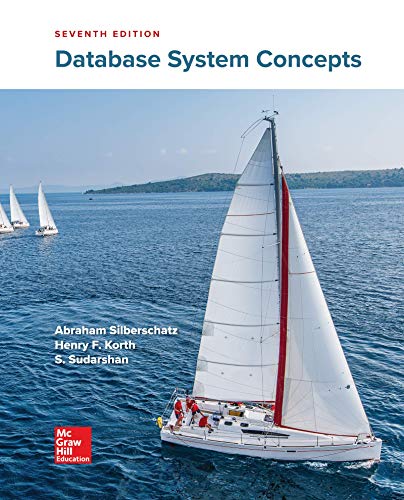
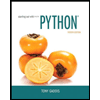
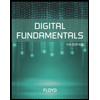
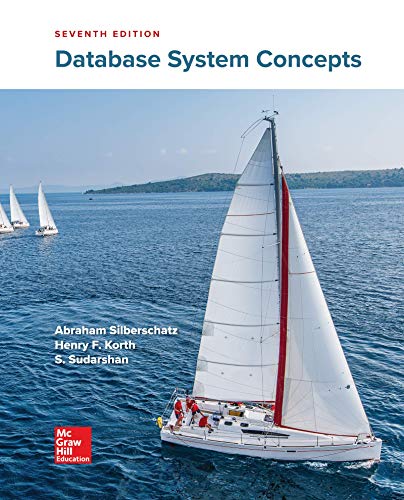
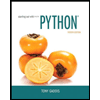
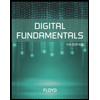
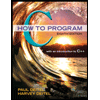
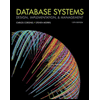
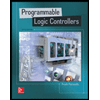