while True: p = float (input ("Enter the starting principal, 0 to quit: ")) if p <= 0: print ("Program exiting ....") break r = float(input ("Enter the annual interest rate: ")) n = float(input("How many times per year is the interest compounded? ")) t = float (input("For how many years will the account earn interest? ")) total = p * (1 + float (r / 100) / n) ** (n * t) interest = total - p print ("At the end of {:.lf} years you will have $ {:,.2f} with interest earned $ {:.2f}".format(t, total, interest) print ('')
while True: p = float (input ("Enter the starting principal, 0 to quit: ")) if p <= 0: print ("Program exiting ....") break r = float(input ("Enter the annual interest rate: ")) n = float(input("How many times per year is the interest compounded? ")) t = float (input("For how many years will the account earn interest? ")) total = p * (1 + float (r / 100) / n) ** (n * t) interest = total - p print ("At the end of {:.lf} years you will have $ {:,.2f} with interest earned $ {:.2f}".format(t, total, interest) print ('')
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Turn the image given into a function that can be imported into another
Using While Loop the program should prompt the user for these 2 choices:
1 Calculate Interest Rate
99 Quit
If 1 was selected, then the program should run the function to find interest rate. If 99 was selected, then the program exits.
![```python
while True:
p = float(input("Enter the starting principal, 0 to quit: "))
if p <= 0:
print("Program exiting ....")
break
r = float(input("Enter the annual interest rate: "))
n = float(input("How many times per year is the interest compounded? "))
t = float(input("For how many years will the account earn interest? "))
total = p * (1 + float(r / 100) / n) ** (n * t)
interest = total - p
print("At the end of {:.1f} years you will have $ {:,.2f} with interest earned $ {:,.2f}".format(t, total, interest))
print('')
```
### Explanation:
This Python script calculates the future value of an investment based on compound interest. Below is an explanation of the key components:
1. **Loop Structure**:
- `while True:` creates an infinite loop that will continually run until broken out of.
- The loop will exit if the user inputs a principal (`p`) of 0 or less, at which point the message "Program exiting ...." is printed.
2. **User Input**:
- The script prompts the user to input:
- `p`: The starting principal.
- `r`: The annual interest rate.
- `n`: The number of times the interest is compounded per year.
- `t`: The number of years the money is invested.
3. **Compound Interest Calculation**:
- `total`: The future value of the investment is calculated using the formula:
\[
\text{total} = p \times \left(1 + \frac{r / 100}{n}\right)^{(n \times t)}
\]
- `interest`: The interest earned is determined by subtracting the principal from the total value.
4. **Output**:
- The script prints the total amount at the end of the investment period and the interest earned, formatted to two decimal places for monetary precision.
- Additional spacing is inserted for readability.
This code is useful for understanding how compound interest affects the growth of an investment over time.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdec6af38-3348-4b7d-979b-f3d453210b87%2F3e421aac-70b8-41dc-b186-45c4b95220bb%2Fvhs2swq_processed.png&w=3840&q=75)
Transcribed Image Text:```python
while True:
p = float(input("Enter the starting principal, 0 to quit: "))
if p <= 0:
print("Program exiting ....")
break
r = float(input("Enter the annual interest rate: "))
n = float(input("How many times per year is the interest compounded? "))
t = float(input("For how many years will the account earn interest? "))
total = p * (1 + float(r / 100) / n) ** (n * t)
interest = total - p
print("At the end of {:.1f} years you will have $ {:,.2f} with interest earned $ {:,.2f}".format(t, total, interest))
print('')
```
### Explanation:
This Python script calculates the future value of an investment based on compound interest. Below is an explanation of the key components:
1. **Loop Structure**:
- `while True:` creates an infinite loop that will continually run until broken out of.
- The loop will exit if the user inputs a principal (`p`) of 0 or less, at which point the message "Program exiting ...." is printed.
2. **User Input**:
- The script prompts the user to input:
- `p`: The starting principal.
- `r`: The annual interest rate.
- `n`: The number of times the interest is compounded per year.
- `t`: The number of years the money is invested.
3. **Compound Interest Calculation**:
- `total`: The future value of the investment is calculated using the formula:
\[
\text{total} = p \times \left(1 + \frac{r / 100}{n}\right)^{(n \times t)}
\]
- `interest`: The interest earned is determined by subtracting the principal from the total value.
4. **Output**:
- The script prints the total amount at the end of the investment period and the interest earned, formatted to two decimal places for monetary precision.
- Additional spacing is inserted for readability.
This code is useful for understanding how compound interest affects the growth of an investment over time.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
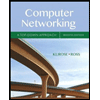
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
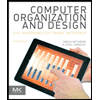
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
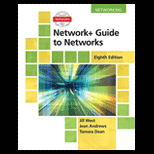
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
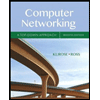
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
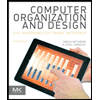
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
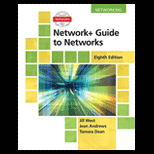
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
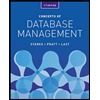
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
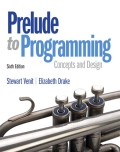
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
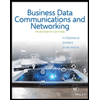
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY