Which one is the key thing in backtracking? Insertion Recursion Submission In what manner is a state-space tree for a backtracking algorithm constructed? Depth-first search Breadth-first search Twice around the tree Nearest neighbour first What does the following function do? int fun(int x, int y) { if (y == 0) return 0; return (x + fun(x, y-1)); } x + y x + x*y x*y xy
Which one is the key thing in backtracking?
Insertion
Recursion
Submission
In what manner is a state-space tree for a backtracking
Depth-first search
Breadth-first search
Twice around the tree
Nearest neighbour first
What does the following function do?
int fun(int x, int y)
{
if (y == 0) return 0;
return (x + fun(x, y-1));
}
x + y
x + x*y
x*y
xy
Consider the following recursive function fun(x, y). What is the value of fun(4, 3)
int fun(int x, int y)
{
if (x == 0)
return y;
return fun(x - 1, x + y);
}
10
9
13
12
Predict output of following program
16
4
Runtime Error
8
Assume you have the following backtracking based sorting algorithm:
bool Backtracking_Sort(sorted_list, unsorted_list, index, length) {
if (index == length) {
return true;
}
Potential_values = [];
If (index != 0) {
Potential_values = get_larger_unused_values(sorted_list, unsorted_list, index);
} else {
Potential_values = copy(unsorted_list);
}
While(NotEmpty(potential_values) {
Next_value = Choose_Random_Element(potential_values);
Sorted_list[index] = next_value;
If(Backtracking_Sort(sorted_list, unsorted_list, index + 1, length) == true){
Return true;
}
Potential_Values.Remove(next_value);
}
return false;
}
Assume that you have an array of length 20, what is the base case for this recursive algorithm?
When Index = 19
When Index == 20
When Index = 21
When Index == 0
When Index = 10
What is the “dead end” condition in this algorithm which forces the backtrack?
When index == length
When NotEmpty(potential_values) is true
When Index != 0
When Index == 0
When NotEmpty(potential_values) is false
Assume you have the unsorted list [10, 3, 9, 5, 8] and have a partially created sorted list equal to [3, 8] index==2, what are the potential values for the next value in the sorted list?
9
10
9, 10
5, 3, 8
10, 9, 5, 8
Assume you have a list of 100 elements, in the first call of the algorithm how many potential candidates are there for the first element of the sorted list?
100
50
99
101
98
Which of the following lines of code prevents the algorithm from choosing already explored candidate values twice?
Next_value = Choose_Random_Element(potential_values);
Potential_values = get_larger_unused_values(sorted_list, unsorted_list, index);
Sorted_list[index] = next_value;
Potential_values = copy(unsorted_list)
Potential_Values.Remove(next_value);

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

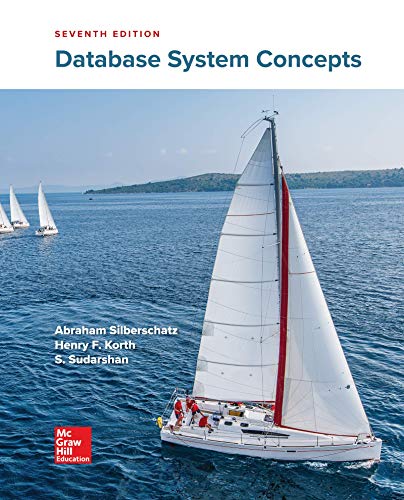
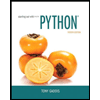
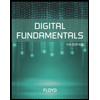
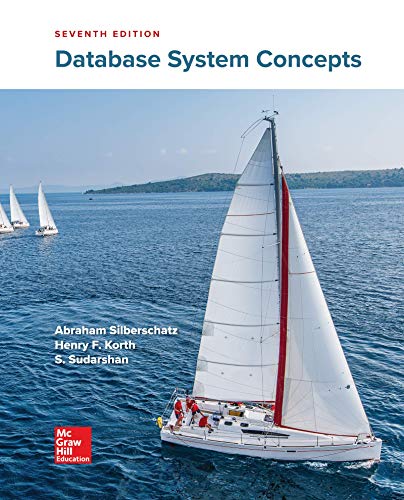
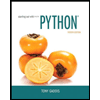
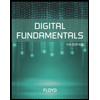
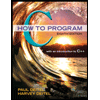
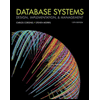
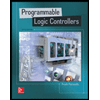