Where do I place a return method for finding the area of a square, triangle, and rectangle? Also are the math equations correct for each shape? //PSEUDOCODE //Write a program that demonstrates method overloading by defining and calling methods that return the area //of a triangle //a rectangle //a square private static void area(float a) { System.out.println("The area of the square is " + String.format("%,.2f", Math.pow(a,2)) + " sq units"); } private static void area1 (float a, float b) { System.out.println("The area a of the rectangle is " + String.format("%,.2f", a * b) + " sq units"); } private static void area (float a, float b) { double c = 0.5 * (a * b); System.out.println("The area of the triangle is " + String.format("%,.2f", c) + " sq units"); } public static void main(String[] args) { area(5); area1(05,21); area(05,05); } }
Where do I place a return method for finding the area of a square, triangle, and rectangle? Also are the math equations correct for each shape?
//PSEUDOCODE
//Write a program that demonstrates method overloading by defining and calling methods that return the area
//of a triangle
//a rectangle
//a square
private static void area(float a) {
System.out.println("The area of the square is " + String.format("%,.2f", Math.pow(a,2)) + " sq units");
}
private static void area1 (float a, float b) {
System.out.println("The area a of the rectangle is " + String.format("%,.2f", a * b) + " sq units");
}
private static void area (float a, float b) {
double c = 0.5 * (a * b);
System.out.println("The area of the triangle is " + String.format("%,.2f", c) + " sq units");
}
public static void main(String[] args) {
area(5);
area1(05,21);
area(05,05);
}
}

Step by step
Solved in 2 steps

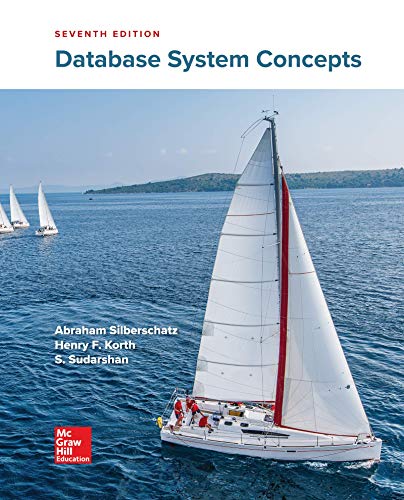
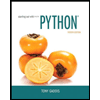
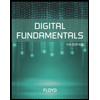
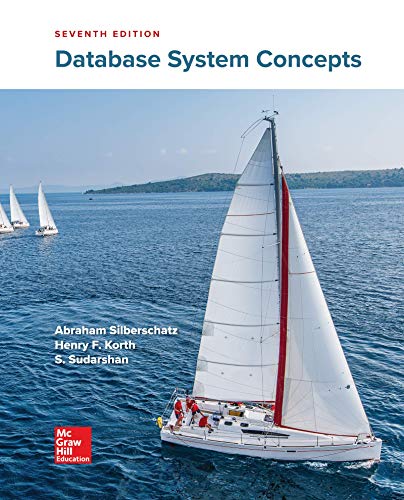
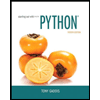
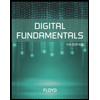
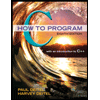
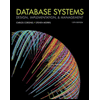
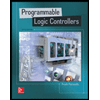