When the add button is pressed, the number in the (0)Cart link should increase. When the remove button is pressed, the number should decrease. When I click on the link, it should take me to a checkout.html page. The checkout.html page should display all the items added in the cart, and a total price. Existing code: products.html Products (0) Cart Price: $10 Name: Clorox Description: Household Product ID: product1 Add to cart Remove from cart Price: $15 Name: Oxiclean Description: Product Packaging ID: product2 Add to cart Remove from cart Price: $20 Name: Pinesol Description: Household Product ID: product3 Add to cart Remove from cart Price: $15 Name: Mr Clean Description: Household Product ID: product4 Add to cart Remove from cart Price: $10 Name: Windex Description: Household Product ID: product5 Add to cart Remove from cart shoppingcart.js var cart=[]; var productArray=[]; productArray.push({Price:"$10",Name:"Clorox",Description:"Household Product",ID:"product1"}); productArray.push({Price:"$15",Name:"Oxiclean",Description:"Product Packaging",ID:"product2"}); productArray.push({Price:"$20",Name:"Pinesol",Description:"Household Product",ID:"product3"}); productArray.push({Price:"$15",Name:"Mr Clean",Description:"Household Product",ID:"product4"}); productArray.push({Price:"$10",Name:"Windex",Description:"Household Product",ID:"product5"}); function Add(id){ const index = productArray.findIndex(x => x.ID ===id); cart.push(productArray[index]); } function Remove(id){ const index = cart.findIndex(x => x.ID ===id); cart.splice(index, 1); document.getElementById("whereToPrint").innerHTML = JSON.stringify(cart, null, 4); return whereToPrint; }
When the add button is pressed, the number in the (0)Cart link should increase. When the remove button is pressed, the number should decrease. When I click on the link, it should take me to a checkout.html page. The checkout.html page should display all the items added in the cart, and a total price.
Existing code:
products.html
<!DOCTYPE html>
<html>
<head>
<title>Products</title>
<script src="./js/shoppingcart.js"></script>
</head>
<body>
<div>
<div>
<a href="Checkout.html">
<span style="float:right"><p>(<span>0</span>) Cart</p></span>
</a>
</div>
<div class="product">
<img src="img/clorox.jpg">
<p class="price">Price: $10</p>
<p class="name">Name: Clorox</p>
<p class="description">Description: Household Product</p>
<p class="id">ID: product1</p>
<span><button id="product1">
Add to cart
</button>
<button>
Remove from cart
</button>
</span>
</div>
<br>
<div class="product">
<img src="img/oxiclean.jpg">
<p class="price">Price: $15</p>
<p class="name">Name: Oxiclean</p>
<p class="description">Description: Product Packaging</p>
<p class="id">ID: product2</p>
<span><button id="product2">
Add to cart
</button>
<button>
Remove from cart
</button>
</span>
</div>
<br>
<div class="product">
<img src="img/pinesol.jpg">
<p class="price">Price: $20</p>
<p class="name">Name: Pinesol</p>
<p class="description">Description: Household Product</p>
<p class="id">ID: product3</p>
<span><button id="product3">
Add to cart
</button>
<button>
Remove from cart
</button>
</span>
</div>
<br>
<div class="product">
<img src="img/mrclean.jpg">
<p class="price">Price: $15</p>
<p class="name">Name: Mr Clean</p>
<p class="description">Description: Household Product</p>
<p class="id">ID: product4</p>
<span><button id="product4">
Add to cart
</button>
<button>
Remove from cart
</button>
</span>
</div>
<br>
<div class="product">
<img src="img/windex.jpg">
<p class="price">Price: $10</p>
<p class="name">Name: Windex</p>
<p class="description">Description: Household Product</p>
<p class="id">ID: product5</p>
<span><button id="product5">
Add to cart
</button>
<button>
Remove from cart
</button>
</span>
</div>
<br>
</div>
<pre id="whereToPrint"></pre>
</body>
</html>
shoppingcart.js
var cart=[];
var productArray=[];
productArray.push({Price:"$10",Name:"Clorox",Description:"Household Product",ID:"product1"});
productArray.push({Price:"$15",Name:"Oxiclean",Description:"Product Packaging",ID:"product2"});
productArray.push({Price:"$20",Name:"Pinesol",Description:"Household Product",ID:"product3"});
productArray.push({Price:"$15",Name:"Mr Clean",Description:"Household Product",ID:"product4"});
productArray.push({Price:"$10",Name:"Windex",Description:"Household Product",ID:"product5"});
function Add(id){
const index = productArray.findIndex(x => x.ID ===id);
cart.push(productArray[index]);
}
function Remove(id){
const index = cart.findIndex(x => x.ID ===id);
cart.splice(index, 1);
document.getElementById("whereToPrint").innerHTML = JSON.stringify(cart, null, 4);
return whereToPrint;
}

Step by step
Solved in 2 steps

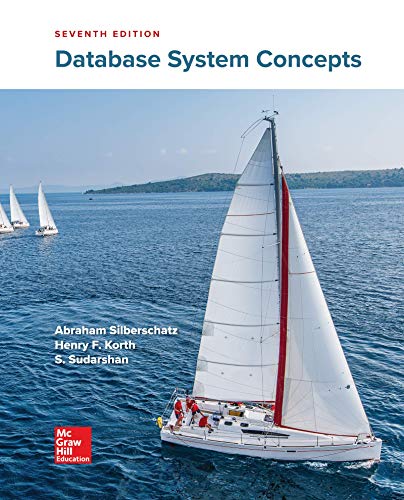
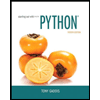
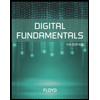
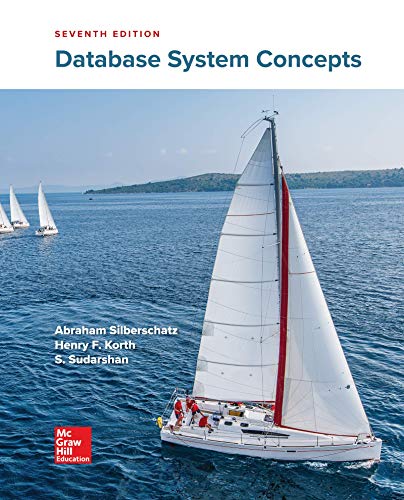
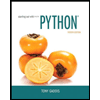
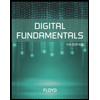
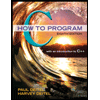
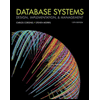
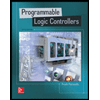