When I was compling my code this is the output I got. yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data And this is what the output is supposed to looked like Dynamic memory for 0 CNodes freed Dynamic memory for 1 PNodes freed 11 11 Dynamic memory for 1 CNodes freed Dynamic memory for 1 PNodes freed 11 12 13 14 11 12 13 14 Dynamic memory for 4 CNodes freed Dynamic memory for 1 PNodes freed 21 22 23 24 21 22 23 24 Dynamic memory for 0 CNodes freed Dynamic memory for 4 CNodes freed Dynamic memory for 2 PNodes freed
When I was compling my code this is the output I got.
yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data (int): Add more cList data? (n = no, others = yes) Enter cList data
And this is what the output is supposed to looked like
Dynamic memory for 0 CNodes freed
Dynamic memory for 1 PNodes freed
11
11
Dynamic memory for 1 CNodes freed
Dynamic memory for 1 PNodes freed
11 12 13 14
11 12 13 14
Dynamic memory for 4 CNodes freed
Dynamic memory for 1 PNodes freed
21 22 23 24
21 22 23 24
Dynamic memory for 0 CNodes freed
Dynamic memory for 4 CNodes freed
Dynamic memory for 2 PNodes freed
11 12 13 14
11 12 13 14
Dynamic memory for 4 CNodes freed
Dynamic memory for 0 CNodes freed
Dynamic memory for 2 PNodes freed
11 12 13 14 21 41 42
11 21 41 12 42 13 14
Dynamic memory for 4 CNodes freed
Dynamic memory for 1 CNodes freed
Dynamic memory for 0 CNodes freed
Dynamic memory for 2 CNodes freed
Dynamic memory for 4 PNodes freed
21 22 23 24 31 51 52
21 31 51 22 52 23 24
Dynamic memory for 0 CNodes freed
Dynamic memory for 4 CNodes freed
Dynamic memory for 1 CNodes freed
Dynamic memory for 0 CNodes freed
Dynamic memory for 2 CNodes freed
Dynamic memory for 5 PNodes freed
#ifndef NODES_LLOLL_H
#define NODES_LLOLL_H
#include <iostream> // for ostream
namespace CS3358_SP2023_A5P2
{
// child node
struct CNode
{
int data;
CNode* link;
};
// parent node
struct PNode
{
CNode* data;
PNode* link;
};
// toolkit functions for LLoLL based on above node definitions
void Destroy_cList(CNode*& cListHead);
void Destroy_pList(PNode*& pListHead);
void ShowAll_DF(PNode* pListHead, std::ostream& outs);
void ShowAll_BF(PNode* pListHead, std::ostream& outs);
}
#endif
#ifndef CN_PTR_QUEUE_H
#define CN_PTR_QUEUE_H
#include <cstdlib> // for size_t
#include <stack> // for STL stack template
#include "nodes_LLoLL.h" // for CNode
namespace CS3358_SP2023_A5P2
{
class cnPtrQueue
{
public:
typedef std::size_t size_type;
cnPtrQueue();
bool empty() const;
size_type size() const; // returns # of items in queue
CNode* front();
void push(CNode* cnPtr);
void pop();
private:
std::stack<CNode*> inStack;
std::stack<CNode*> outStack;
size_type numItems; // # of items in queue
};
}
#endif



Step by step
Solved in 4 steps with 1 images

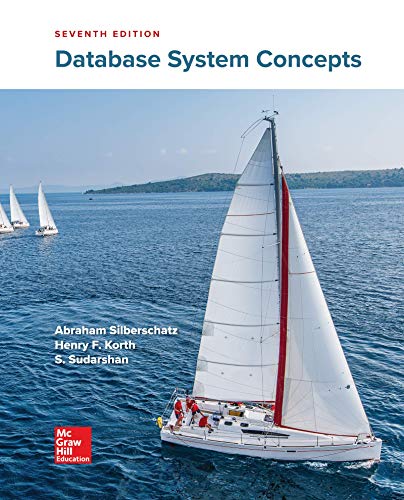
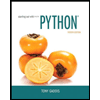
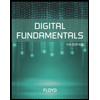
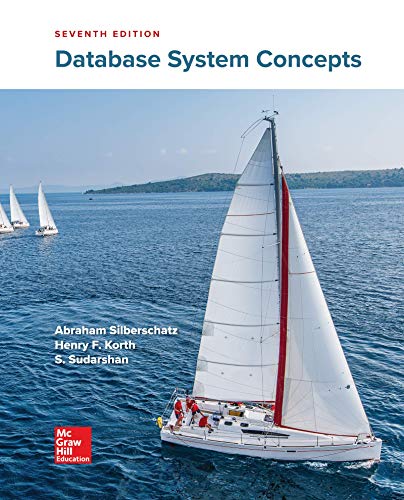
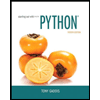
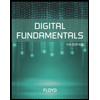
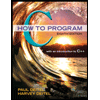
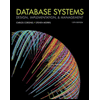
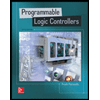