Part 1 - Create a header comment with the title of this program, your name, and the date. Part 2 (Taking in user input) Part 2 Ask the user for two numbers. You can assume that the user will always give two positive integer numbers or "DONE". Prompt the user for an operand, the accepted operands are 11 1 1
Part 1 - Create a header comment with the title of this program, your name, and the date. Part 2 (Taking in user input) Part 2 Ask the user for two numbers. You can assume that the user will always give two positive integer numbers or "DONE". Prompt the user for an operand, the accepted operands are 11 1 1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can you solve this problem using Python? Thanks.

Transcribed Image Text:**Lesson: Understanding Basic Programming Operations**
---
**Part 1: Header**
In this lesson, students will create a simple program that takes user input to perform basic calculations. Begin by creating a header that includes the title of the program, your name, and the date.
---
**Part 2: Taking in User Input**
Start by creating a program that prompts the user for two numbers and an operation. The program should continue to prompt the user until they type "DONE." Each operation will be calculated and displayed immediately.
**Example Input/Output:**
```
What is the first number: 7
What is the second number: 7
What operation: +
7 + 7 = 14
What is the first number: 6
What is the second number: 4
What operation: DONE
```
If an invalid operation is entered, notify the user:
```
What is the first number: 7
What is the second number: 3
What operation: *
Invalid operand
What is the first number: DONE
What is the second number: 8
What operation: DONE
```
---
**Part 3: Calculations**
**1) Basic Addition:**
Add the first number to the second number.
Example:
```
What is the first number: 6
What is the second number: 7
What operation: +
6 + 7 = 13
```
**2) Multiplication:**
Multiply the first number by the second number.
Example:
```
What is the first number: 6
What is the second number: 12
What operation: *
6 * 12 = 72
```
**3) Division:**
Divide the first number by the second number and state the remainder if necessary.
Example:
```
What is the first number: 47
What is the second number: 3
What operation: /
47 / 3 = 15 with a remainder of 2
```
**Complete Mock User Input:**
Example:
```
What is the first number: 6
What is the second number: 9
What operation: +
6 + 9 = 15
What is the first number: 40
What is the second number: 3
What operation: /
40 / 3 = 13 with a remainder of 1
What is the first number: 9
What is the second number:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
When I input "DONE", how will I do like example shown in image?
Thanks
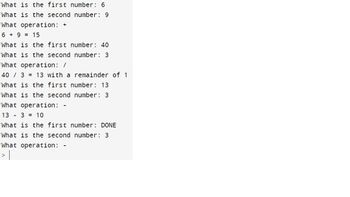
Transcribed Image Text:### Mathematical Operations and Examples
1. **Addition Example:**
- What is the first number: 6
- What is the second number: 9
- What operation: +
- Calculation: \(6 + 9 = 15\)
2. **Division Example:**
- What is the first number: 40
- What is the second number: 3
- What operation: /
- Calculation: \(40 / 3 = 13\) with a remainder of 1
3. **Subtraction Example:**
- What is the first number: 13
- What is the second number: 3
- What operation: -
- Calculation: \(13 - 3 = 10\)
4. **Termination:**
- What is the first number: DONE
- What is the second number: 3
- What operation: -
This sequence demonstrates basic arithmetic operations: addition, division with remainder, and subtraction. The input concludes with the word "DONE," indicating the end of operations.
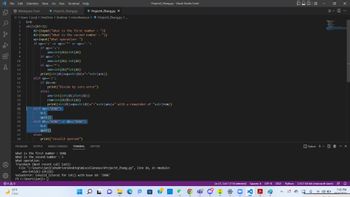
Transcribed Image Text:## Python Script for Basic Arithmetic Operations
This Python script is designed to perform basic arithmetic operations—addition, subtraction, multiplication, and division—on two input numbers. Below is a detailed breakdown of the code structure.
### Code Explanation:
- **Initialization**:
```python
b=0
while(b!=1):
```
The variable `b` is used as a control for the while loop, which keeps the program running until an exit condition is met.
- **Input Handling**:
```python
d1=(input("What is the first number : "))
d2=(input("What is the second number : "))
op=input("What operation: ")
```
The program takes input for two numbers (`d1` and `d2`) and one arithmetic operation (`op`).
- **Condition Checking**:
```python
if op== "done" or op =="DONE":
```
This section checks if the user wants to exit the program.
- **Operations**:
- **Addition**:
```python
if op=="+":
ans=int(d1)+int(d2)
print(str(d1)+op+str(d2)+"="+str(ans))
```
- **Subtraction**:
```python
if op=="-":
ans=int(d1)-int(d2)
print(str(d1)+op+str(d2)+"="+str(ans))
```
- **Multiplication**:
```python
if op=="*":
ans=int(d1)*int(d2)
print(str(d1)+op+str(d2)+"="+str(ans))
```
- **Division**:
```python
elif op=="/":
if d2=="0":
print("Divide by zero error")
else:
ans=int(d1)/int(d2)
rem=int(d1)%int(d2)
print(str(d1)+op+str(d2)+"="+str(ans)+ " with a remainder of "+str(rem))
```
- **Exit Conditions**:
```python
elif op=="DONE" or d2=="DONE":
b=1
quit()
```
If the user inputs "DONE", the program will terminate.
- **Error Handling**:
```python
else:
print("Invalid operand")
```
Solution
Follow-up Question
I have a question. When I want to input "DONE" in inputing number part like below:
What is the first number: DONE
or
What is the second number: DONE
How will I do that? Thanks.
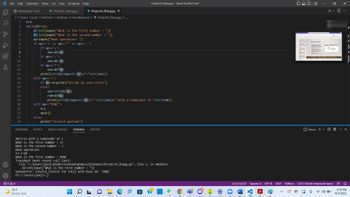
Transcribed Image Text:### Python Program to Perform Basic Arithmetic Operations
This Python code is designed to perform basic arithmetic operations: addition, subtraction, multiplication, and division. Let's go through the code step-by-step:
1. **Variable Initialization**:
- `b = 0`: This variable is used as a flag for the while loop control.
2. **While Loop**:
- The loop continues as long as `b` is equal to 1.
- Two numbers, `d1` and `d2`, are input from the user.
- The user is prompted to specify an operation: `+` for addition, `-` for subtraction, `*` for multiplication, or `/` for division.
3. **Operation Execution**:
- If the input operator is `+`, `d1` and `d2` are added.
- If the input operator is `-`, `d2` is subtracted from `d1`.
- If the input operator is `*`, `d1` and `d2` are multiplied.
- If the input operator is `/`, it checks for a divide-by-zero condition. If not zero, it divides `d1` by `d2` and calculates the remainder.
4. **Exit Command**:
- If the user inputs `DONE`, the loop terminates.
5. **Error Handling**:
- If an invalid operator is entered, the program prints "Invalid operand".
### Terminal Output
- The terminal output shows a history of inputs and results along with corresponding operations and potential errors.
- Example outputs include results of operations and error messages for invalid inputs.
This program allows users to perform continuous calculations until they choose to exit by typing "DONE". Note that it handles common errors like division by zero and invalid operand entries.
Solution
Follow-up Question
How to fix the error shown in the picture? Thanks.
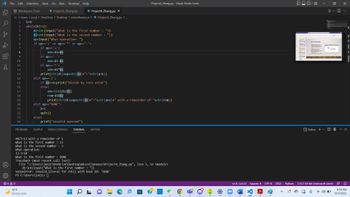
Transcribed Image Text:% 4 A
File Edit Selection View Go Run Terminal Help
Workspace Trust
→ Project3_Zhang.py
Project4_Zhang.py X
C: > Users > junji > OneDrive > Desktop > miscellaneous > Project4_Zhang.py > ...
1
b=0
2
3
4
5
6
7
8
9
Ⓒ⁰A0
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
while(b!=1):
d1=int (input ("What is the first number : "))
d2=int (input ("What is the second number : "))
op=input("What operation: ")
if op=='+' or op=='*' or op=='-':
if op=='+':
ans=d1+d2
if op=='-':
56°F
Mostly clear
ans=d1-d2
if op=='*':
ans=d1*d2
print (str(d1)+op+str(d2)+"="+str(ans))
elif op=='/' :
if d2==0:print("Divide by zero error")
else:
else:
ans-int (d1/d2)
rem=d1%d2
elif op=="DONE":
print (str(d1) +op+str(d2)+"="+str(ans)+" with a remainder of "+str(rem))
b=1
quit()
print("Invalid operand")
PROBLEMS OUTPUT DEBUG CONSOLE TERMINAL JUPYTER
Project4_Zhang.py - Visual Studio Code
40/3=13 with a remainder of 1
What is the first number: 13
What is the second number : 3
What operation:
13-3=10
What is the first number : DONE
Traceback (most recent call last):
File "c:\Users\junji\OneDrive\Desktop\miscellaneous\Project4_Zhang.py", line 3, in <module>
d1=int (input("What is the first number : "))
ValueError: invalid_literal for int() with base 10: 'DONE'
PS C:\Users\junji> [
H
is
■
Ln 8, Col 22 Spaces: 4
UTF-8
08
US 01 LOGIN PAGE
Python + ✓
0
$
☐ Û
8
X
...
CRLF Python 3.10.7 64-bit (microsoft store) o
6:59 PM
10/3/2022
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
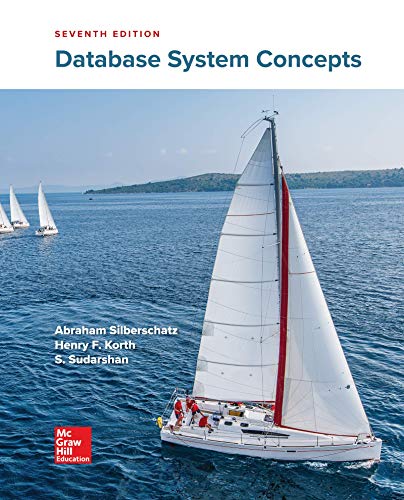
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
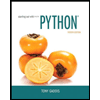
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
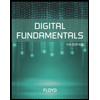
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
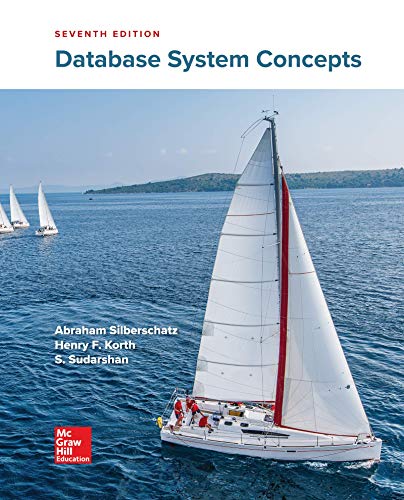
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
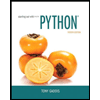
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
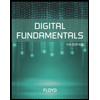
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
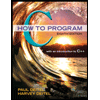
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
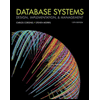
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
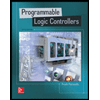
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education