What is this code doing exactly? Please explain in detail so I can better understand the process. Thank you #include #include #include #include #include #define MAX 100 static void * threadFunc (void *arg) { char *str = (char *) arg; int count = 0; printf("\nThread: threadFunc started \n"); while(1) { sleep(1); printf("\n threadFunc: executing loop \n"); } sleep(5); printf("\nThread: threadFunc exiting \n"); } int main(int argc, char *argv[]) // init thread or main thread { pthread_t t1_id; void *res; int s; int count; s = pthread_create(&t1_id, NULL, threadFunc, NULL); if ( s != 0 ) //if creating the new thread is successful, then s will be zero. { perror("Thread create error"); } for (count = 0; count < 10; count++) { sleep(2); printf("\n main thread: count value = (%d) \n", count); } pthread_cancel(t1_id); printf("main thread: exiting now \n "); pthread_exit(NULL); //The main will exit without waiting for the thread. The thread will exit without expecting to send a // a result to the main. }
What is this code doing exactly? Please explain in detail so I can better understand the process. Thank you
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MAX 100
static void * threadFunc (void *arg)
{
char *str = (char *) arg;
int count = 0;
printf("\nThread: threadFunc started \n");
while(1) {
sleep(1);
printf("\n threadFunc: executing loop \n");
}
sleep(5);
printf("\nThread: threadFunc exiting \n");
}
int main(int argc, char *argv[]) // init thread or main thread
{
pthread_t t1_id;
void *res;
int s;
int count;
s = pthread_create(&t1_id, NULL, threadFunc, NULL);
if ( s != 0 ) //if creating the new thread is successful, then s will be zero.
{
perror("Thread create error");
}
for (count = 0; count < 10; count++)
{
sleep(2);
printf("\n main thread: count value = (%d) \n", count);
}
pthread_cancel(t1_id);
printf("main thread: exiting now \n ");
pthread_exit(NULL); //The main will exit without waiting for the thread. The thread will exit without expecting to send a
// a result to the main.
}

Step by step
Solved in 3 steps

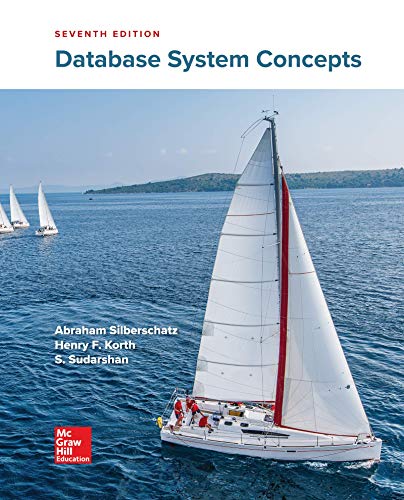
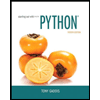
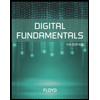
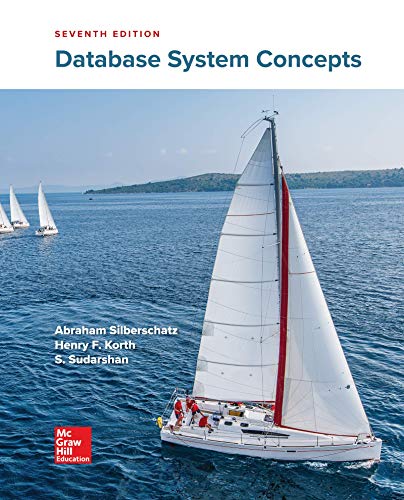
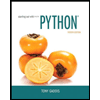
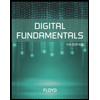
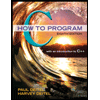
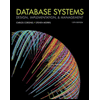
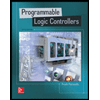