What is the result of this code? int number = 5; while(number } > 3) { System.out.println(number); number--;
What is the result of this code? int number = 5; while(number } > 3) { System.out.println(number); number--;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![---
### UNIT 2 — MILESTONE 2
#### Question 11
**What is the result of this code?**
```java
int number = 5;
while(number > 3) {
System.out.println(number);
number--;
}
```
##### Options:
- [ ] 5
- [ ] 5 4
- [ ] 3 4 5
- [x] 5 4 3
---
### Explanation:
This code snippet is written in Java and it uses a `while` loop to print numbers. Here is a breakdown of how the code works:
1. The variable `number` is initialized to 5.
2. The `while` loop checks if `number` is greater than 3.
3. If the condition is true, it prints the current value of `number`.
4. The statement `number--` decrements the value of `number` by 1.
5. The loop repeats this process until the condition `(number > 3)` is no longer true.
**Detailed Execution:**
- `number` starts at 5.
- It checks `5 > 3` → true
- Prints `5`
- Decrements to `4`
- `number` is now 4.
- It checks `4 > 3` → true
- Prints `4`
- Decrements to `3`
- `number` is now 3.
- It checks `3 > 3` → false
- Loop ends since the condition is no longer met.
Thus, the numbers printed sequentially are **5 4**, and the correct answer is the second option: **5 4 3**.
---](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa9ed15ea-b247-4d4a-84f5-d7f808a88737%2F533bf63d-4dac-4a99-addb-4cfe21346f4c%2Fhbg3w1_processed.jpeg&w=3840&q=75)
Transcribed Image Text:---
### UNIT 2 — MILESTONE 2
#### Question 11
**What is the result of this code?**
```java
int number = 5;
while(number > 3) {
System.out.println(number);
number--;
}
```
##### Options:
- [ ] 5
- [ ] 5 4
- [ ] 3 4 5
- [x] 5 4 3
---
### Explanation:
This code snippet is written in Java and it uses a `while` loop to print numbers. Here is a breakdown of how the code works:
1. The variable `number` is initialized to 5.
2. The `while` loop checks if `number` is greater than 3.
3. If the condition is true, it prints the current value of `number`.
4. The statement `number--` decrements the value of `number` by 1.
5. The loop repeats this process until the condition `(number > 3)` is no longer true.
**Detailed Execution:**
- `number` starts at 5.
- It checks `5 > 3` → true
- Prints `5`
- Decrements to `4`
- `number` is now 4.
- It checks `4 > 3` → true
- Prints `4`
- Decrements to `3`
- `number` is now 3.
- It checks `3 > 3` → false
- Loop ends since the condition is no longer met.
Thus, the numbers printed sequentially are **5 4**, and the correct answer is the second option: **5 4 3**.
---
![### Unit 2 — Milestone 2
#### Problem Statement
Given the following method, the output is expected to search an array of 4 characters for the letter 'x'. If it is found, the location of it is returned.
**Question:** What is the issue?
```java
public static int checkForValue(char[] val) {
int location = 0;
for(int num = 0; num <= 3; num++) {
if(val[num] == 'x') {
location = num + 1;
break;
}
}
return num;
}
```
**Possible Options:**
1. The loop.
2. The concatenation.
3. The parameter.
4. The return statement.
#### Analysis
- **The Loop:** This part of the code is correctly iterating through the first four elements of the array.
- **Concatenation:** There is no concatenation involved, so this is not the cause of the issue.
- **The Parameter:** The parameter is correctly set as an array of characters; hence, it is not the issue.
- **The Return Statement:** This might be the issue in the method because returning `num` does not accurately reflect the location of 'x' if found. It should return `location` instead. If 'x' is not found within the loop, `num` would be out of scope.
#### Corrected Code
Here's the corrected code with the return statement fixed to return `location` instead of `num`.
```java
public static int checkForValue(char[] val) {
int location = 0;
for(int num = 0; num <= 3; num++) {
if(val[num] == 'x') {
location = num + 1;
break;
}
}
return location;
}
```
By returning `location`, the method correctly outputs the position of 'x' within the array of characters.
---
This textual explanation along with code snippets could serve as an excellent resource for students learning about debugging and common pitfalls in basic array operations.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa9ed15ea-b247-4d4a-84f5-d7f808a88737%2F533bf63d-4dac-4a99-addb-4cfe21346f4c%2Fut2wksd_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Unit 2 — Milestone 2
#### Problem Statement
Given the following method, the output is expected to search an array of 4 characters for the letter 'x'. If it is found, the location of it is returned.
**Question:** What is the issue?
```java
public static int checkForValue(char[] val) {
int location = 0;
for(int num = 0; num <= 3; num++) {
if(val[num] == 'x') {
location = num + 1;
break;
}
}
return num;
}
```
**Possible Options:**
1. The loop.
2. The concatenation.
3. The parameter.
4. The return statement.
#### Analysis
- **The Loop:** This part of the code is correctly iterating through the first four elements of the array.
- **Concatenation:** There is no concatenation involved, so this is not the cause of the issue.
- **The Parameter:** The parameter is correctly set as an array of characters; hence, it is not the issue.
- **The Return Statement:** This might be the issue in the method because returning `num` does not accurately reflect the location of 'x' if found. It should return `location` instead. If 'x' is not found within the loop, `num` would be out of scope.
#### Corrected Code
Here's the corrected code with the return statement fixed to return `location` instead of `num`.
```java
public static int checkForValue(char[] val) {
int location = 0;
for(int num = 0; num <= 3; num++) {
if(val[num] == 'x') {
location = num + 1;
break;
}
}
return location;
}
```
By returning `location`, the method correctly outputs the position of 'x' within the array of characters.
---
This textual explanation along with code snippets could serve as an excellent resource for students learning about debugging and common pitfalls in basic array operations.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
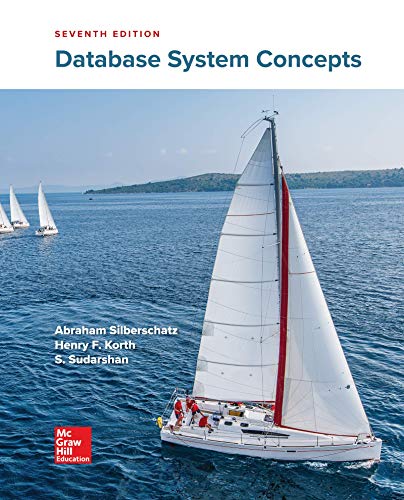
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
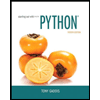
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
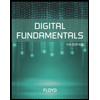
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
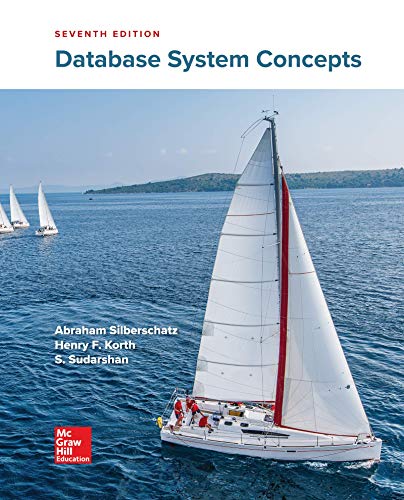
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
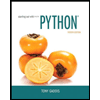
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
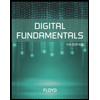
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
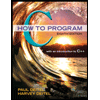
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
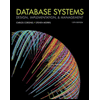
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
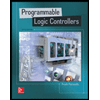
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education