What is the output of the following code? ( Assume each class is defined in a separate java file). public class MyFrame 4. private double length; private double width; public double x; private double y; public MyFrame (double len, double width, double x, double y) { this.length = len; this.width = width; this.x = x; this.y = y; 18 public void MyFrameMinimize (double per) 19 20 21 22 23 24 25 26 27 28 29 public class MyFrameTest{ length -= length * per ; public double getLength() return length; 30 31 32 33 34 35 36 37 public static void main (String [] args) { MyFrame fr = new MyFrame (10, 12, 3, 8 ); fr.MyFrameMinimize (.03); System.out.println(fr.getLength ()); A. 0.0 О В. 9.7 ОС. 10 D. 9.9
What is the output of the following code? ( Assume each class is defined in a separate java file). public class MyFrame 4. private double length; private double width; public double x; private double y; public MyFrame (double len, double width, double x, double y) { this.length = len; this.width = width; this.x = x; this.y = y; 18 public void MyFrameMinimize (double per) 19 20 21 22 23 24 25 26 27 28 29 public class MyFrameTest{ length -= length * per ; public double getLength() return length; 30 31 32 33 34 35 36 37 public static void main (String [] args) { MyFrame fr = new MyFrame (10, 12, 3, 8 ); fr.MyFrameMinimize (.03); System.out.println(fr.getLength ()); A. 0.0 О В. 9.7 ОС. 10 D. 9.9
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
java
![**Question 6:**
What is the output of the following code? (Assume each class is defined in a separate java file).
```java
public class MyFrame
{
private double length;
private double width;
public double x;
public double y;
public MyFrame(double len, double width, double x, double y) {
this.length = len;
this.width = width;
this.x = x;
this.y = y;
}
public void MyFrameMinimize(double per) {
length -= length * per;
}
public double getLength() {
return length;
}
}
public class MyFrameTest {
public static void main(String[] args) {
MyFrame fr = new MyFrame(10, 12, 3, 8);
fr.MyFrameMinimize(0.03);
System.out.println(fr.getLength());
}
}
```
**Choices:**
- A. 0.0
- B. 9.7
- C. 10
- D. 9.9
---
**Explanation:**
To determine the output, let's break down the code step by step:
1. **Class MyFrame:**
- Contains private variables `length` and `width`.
- Contains public variables `x` and `y`.
- Constructor initializes these variables.
- Method `MyFrameMinimize(double per)` reduces the `length` by a percentage `per`.
- Method `getLength()` returns the current `length`.
2. **Class MyFrameTest:**
- The `main` method creates an instance of `MyFrame` with initial variables (10, 12, 3, 8).
- Calls `MyFrameMinimize` with 0.03 (3%).
- Prints the new length using `getLength()`.
Calculation:
- Initial `length` = 10
- After `MyFrameMinimize(0.03)`:
- Length reduced by 3%: `length -= length * 0.03`
- `length = 10 - 10 * 0.03 = 10 - 0.3 = 9.7`
Therefore, the output of `System.out.println(fr.getLength());` will be:
**Answer:**
- B. 9.7](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0e6f6223-c696-4938-9015-1aa854831435%2Fea4a2097-38ef-46d8-983f-94c228639eeb%2Fuaurkal_processed.png&w=3840&q=75)
Transcribed Image Text:**Question 6:**
What is the output of the following code? (Assume each class is defined in a separate java file).
```java
public class MyFrame
{
private double length;
private double width;
public double x;
public double y;
public MyFrame(double len, double width, double x, double y) {
this.length = len;
this.width = width;
this.x = x;
this.y = y;
}
public void MyFrameMinimize(double per) {
length -= length * per;
}
public double getLength() {
return length;
}
}
public class MyFrameTest {
public static void main(String[] args) {
MyFrame fr = new MyFrame(10, 12, 3, 8);
fr.MyFrameMinimize(0.03);
System.out.println(fr.getLength());
}
}
```
**Choices:**
- A. 0.0
- B. 9.7
- C. 10
- D. 9.9
---
**Explanation:**
To determine the output, let's break down the code step by step:
1. **Class MyFrame:**
- Contains private variables `length` and `width`.
- Contains public variables `x` and `y`.
- Constructor initializes these variables.
- Method `MyFrameMinimize(double per)` reduces the `length` by a percentage `per`.
- Method `getLength()` returns the current `length`.
2. **Class MyFrameTest:**
- The `main` method creates an instance of `MyFrame` with initial variables (10, 12, 3, 8).
- Calls `MyFrameMinimize` with 0.03 (3%).
- Prints the new length using `getLength()`.
Calculation:
- Initial `length` = 10
- After `MyFrameMinimize(0.03)`:
- Length reduced by 3%: `length -= length * 0.03`
- `length = 10 - 10 * 0.03 = 10 - 0.3 = 9.7`
Therefore, the output of `System.out.println(fr.getLength());` will be:
**Answer:**
- B. 9.7
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
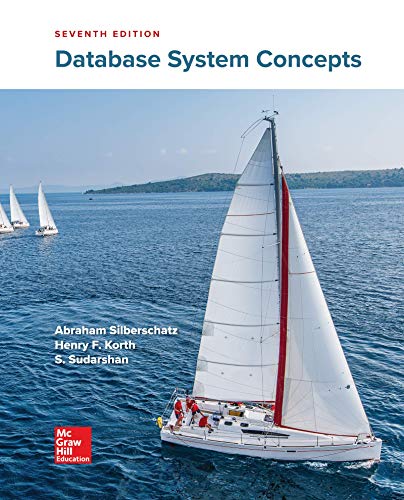
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
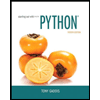
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
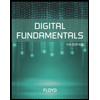
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
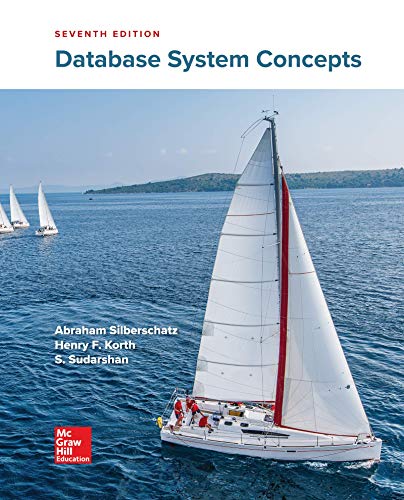
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
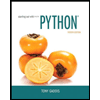
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
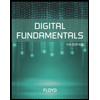
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
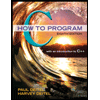
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
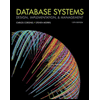
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
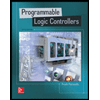
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education