What is the implementation of each instruction located in the main function of the following code? This is a sample program, i am trying to teach myself linked list and am having trouble ------------------------------------------------------------------------------- #include <iostream>#include <string> /*** node* * A node class used to make a linked list structure*/ template<typename type>struct node {node() : data(), next(nullptr) {}node(const type & value, node * link = nullptr) : data(value), next(link) {}type data;node * next;}; /*** print_list* @param list - the linked-list to print out* * Free function that prints out a linked-list space separated.* Templated so works with any node.* Use this for testing your code.*/ template<typename type>void print_list(node<type> * list) { for(node<type> * current = list; current != nullptr; current = current->next) {std::cout << current->data << ' ';}std::cout << '\n';} /*** free_list* @param list - the linked-list whose memory will be freed* * Free function that frees (deletes) a linked-list's memory* Templated so works with any node.*/template<typename type>void free_list(node<type> * list) { node<type> * current = list;while(current != nullptr) {node<type> * temp = current;current = current->next;delete temp;} } int main() {// create a dynamically allocated linked-list of integers with the values (42 12 7 9)// Use print_list to print entire list to verify // insert a new node in the list with value 1024 betweeen the nodes with values 12 and 7// Use print_list to print entire list to verify // remove the node with value 12 from the list// make sure no memory leaks// Use print_list to print entire list to verify // free the integer list // dynamically allocate a list of std::string with the values (As, you, wish)// Use print_list to print entire list to verify // Replace (you) with (pie) by deleting the node with value (you) and// inserting a new node with value (pie)// Use print_list to print entire list to verify // remove the node with value (wish) from the list// make sure no memory leaks// Use print_list to print entire list to verify // Insert a node with value (Easy) at front of list// Use print_list to print entire list to verify // free list of std::string return 0;}
What is the implementation of each instruction located in the main function of the following code?
This is a sample program, i am trying to teach myself linked list and am having trouble
-------------------------------------------------------------------------------
#include <iostream>
#include <string>
/**
* node
*
* A node class used to make a linked list structure
*/
template<typename type>
struct node {
node() : data(), next(nullptr) {}
node(const type & value, node * link = nullptr) : data(value), next(link) {}
type data;
node * next;
};
/**
* print_list
* @param list - the linked-list to print out
*
* Free function that prints out a linked-list space separated.
* Templated so works with any node.
* Use this for testing your code.
*/
template<typename type>
void print_list(node<type> * list) {
for(node<type> * current = list; current != nullptr; current = current->next) {
std::cout << current->data << ' ';
}
std::cout << '\n';
}
/**
* free_list
* @param list - the linked-list whose memory will be freed
*
* Free function that frees (deletes) a linked-list's memory
* Templated so works with any node.
*/
template<typename type>
void free_list(node<type> * list) {
node<type> * current = list;
while(current != nullptr) {
node<type> * temp = current;
current = current->next;
delete temp;
}
}
int main() {
// create a dynamically allocated linked-list of integers with the values (42 12 7 9)
// Use print_list to print entire list to verify
// insert a new node in the list with value 1024 betweeen the nodes with values 12 and 7
// Use print_list to print entire list to verify
// remove the node with value 12 from the list
// make sure no memory leaks
// Use print_list to print entire list to verify
// free the integer list
// dynamically allocate a list of std::string with the values (As, you, wish)
// Use print_list to print entire list to verify
// Replace (you) with (pie) by deleting the node with value (you) and
// inserting a new node with value (pie)
// Use print_list to print entire list to verify
// remove the node with value (wish) from the list
// make sure no memory leaks
// Use print_list to print entire list to verify
// Insert a node with value (Easy) at front of list
// Use print_list to print entire list to verify
// free list of std::string
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

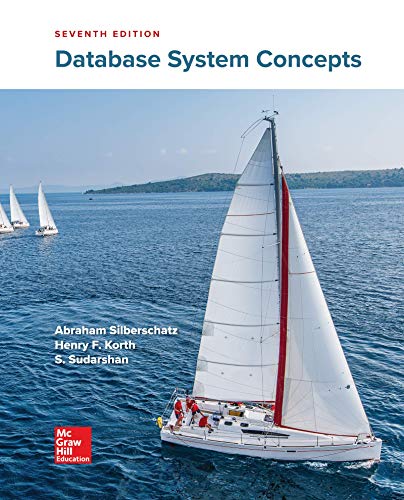
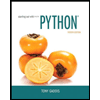
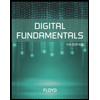
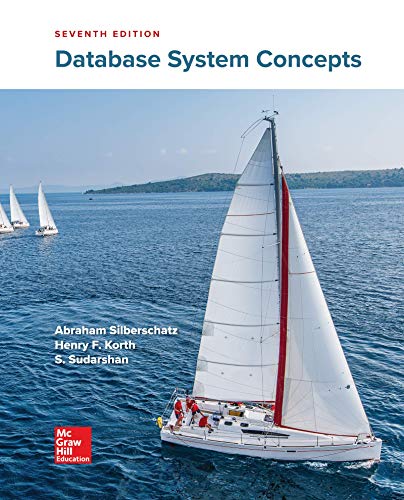
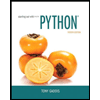
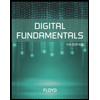
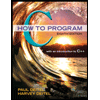
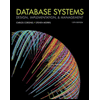
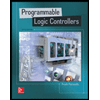