What error is in this code? I keep getting this error but can't find how to solve it. 'printValues (int, int&, int&, int&)' This is the code in c++: /* This program processes the bioryhthm of the users input based on the current date and their birthdate */ #include using namespace std; int daysAlive(); int numDaysReport(); void BioProcessed(int numDaysReport, int daysAlive); void calcValues(int numDaysReport, int&, int&, int&); void printValues(int, int&, int&, int&); int main(void) { int days = daysAlive(); int report = numDaysReport(); BioProcessed (report, days); return 0; } int daysAlive () { int d; cout << "How many days have you been alive? "; cin >> d; return d; } int numDaysReport () { int n; cout << "How many days would you like to be reported? "; cin >> n; return n; } void BioProcessed (int numDaysReport, int daysAlive) { cout << "This report is for someone who has been alive "; cout << daysAlive << endl; int physical; int emotional; int mental; for (int i = 0; i < numDaysReport; i++) { calcValues(daysAlive + i, physical, emotional, mental); printValues(daysAlive + i, physical, emotional, mental); } } void calcValues(int daysAlive, int& physical, int& emotional, int& mental) { physical = sin(2 * 3.14592 * daysAlive / 23.0) * 25 + 25; emotional = sin(2 * 3.14592 * daysAlive / 28.0) * 25 + 25; physical = sin(2 * 3.14592 * daysAlive / 33.0) * 25 + 25; } void printValues(int daysAlive, int physical, int emotional, int mental) { cout << "Day: " << daysAlive << "\t" << physical << "\t" << emotional << "\t" << mental << endl; string output = ".........................|........................."; output[physical] = 'P'; output[emotional] = 'E'; output[mental] = 'M'; cout << output <
What error is in this code? I keep getting this error but can't find how to solve it. 'printValues (int, int&, int&, int&)'
This is the code in c++:
/*
This program processes the bioryhthm of the users input based on the current date and their birthdate
*/
#include <bits/stdc++.h>
using namespace std;
int daysAlive();
int numDaysReport();
void BioProcessed(int numDaysReport, int daysAlive);
void calcValues(int numDaysReport, int&, int&, int&);
void printValues(int, int&, int&, int&);
int main(void)
{
int days = daysAlive();
int report = numDaysReport();
BioProcessed (report, days);
return 0;
}
int daysAlive ()
{
int d;
cout << "How many days have you been alive? ";
cin >> d;
return d;
}
int numDaysReport ()
{
int n;
cout << "How many days would you like to be reported? ";
cin >> n;
return n;
}
void BioProcessed (int numDaysReport, int daysAlive)
{
cout << "This report is for someone who has been alive ";
cout << daysAlive << endl;
int physical;
int emotional;
int mental;
for (int i = 0; i < numDaysReport; i++)
{
calcValues(daysAlive + i, physical, emotional, mental);
printValues(daysAlive + i, physical, emotional, mental);
}
}
void calcValues(int daysAlive, int& physical, int& emotional, int& mental)
{
physical = sin(2 * 3.14592 * daysAlive / 23.0) * 25 + 25;
emotional = sin(2 * 3.14592 * daysAlive / 28.0) * 25 + 25;
physical = sin(2 * 3.14592 * daysAlive / 33.0) * 25 + 25;
}
void printValues(int daysAlive, int physical, int emotional, int mental)
{
cout << "Day: " << daysAlive << "\t" << physical << "\t" << emotional << "\t" << mental << endl;
string output = ".........................|.........................";
output[physical] = 'P';
output[emotional] = 'E';
output[mental] = 'M';
cout << output << endl;
}


According to the question below the solution:
After fix the errors
Output:
Step by step
Solved in 2 steps with 3 images

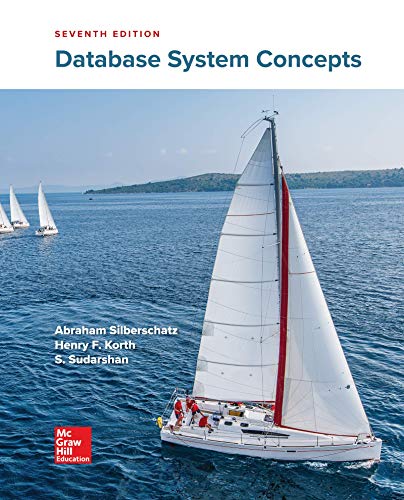
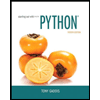
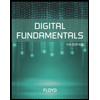
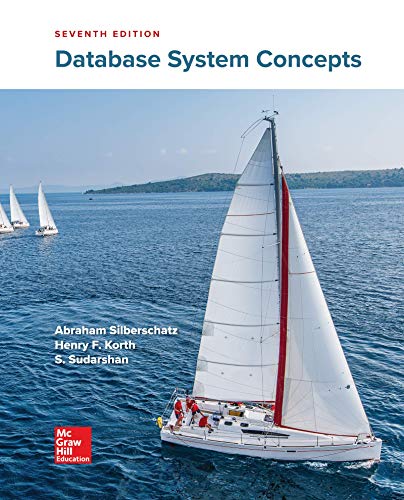
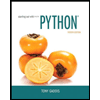
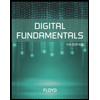
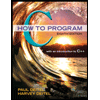
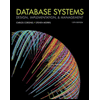
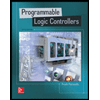