What does the following program do. Explain why. #include class Base { public: void f(int) { std::cout << "i"; } } ; class Derived : Base { public: void f(double) { std::cout << "d"; } }; int main () { Derived d; int i = 0; d.f(i); }
What does the following program do. Explain why. #include class Base { public: void f(int) { std::cout << "i"; } } ; class Derived : Base { public: void f(double) { std::cout << "d"; } }; int main () { Derived d; int i = 0; d.f(i); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help with C++ Question

Transcribed Image Text:### Program Explanation
The provided C++ program demonstrates the concept of method overloading and method hiding in inheritance.
**Code:**
```cpp
#include <iostream>
class Base {
public:
void f(int) { std::cout << "i"; }
};
class Derived : Base {
public:
void f(double) { std::cout << "d"; }
};
int main() {
Derived d;
int i = 0;
d.f(i);
}
```
**Explanation:**
1. **Classes and Inheritance:**
- The program has two classes: `Base` and `Derived`.
- `Derived` inherits from `Base`.
2. **Method Definitions:**
- `Base` has a public method `f(int)` that outputs `"i"`.
- `Derived` defines a method `f(double)` which outputs `"d"`. This method hides the `f(int)` method from the `Base` class because it has the same name.
3. **Main Function:**
- An object `d` of type `Derived` is created.
- An integer `i` is initialized to `0`.
- The method `f(i)` is called on the `Derived` object `d`.
**Behavior and Output:**
Despite the integer `i` being passed, the `Derived` class method `f(double)` is chosen, due to method hiding. Thus, the integer `i` is implicitly converted to a double, and "d" is printed.
**Key Concepts:**
- **Function Overloading:** The ability to define multiple functions with the same name but different parameters.
- **Method Hiding:** In C++, methods in a derived class hide all overloaded methods of the same name in the base class, unless explicitly brought into scope using `using` or similar.
This behavior highlights the nuances of method resolution in C++ inheritance. To access the hidden method from the base class, you could explicitly call it using `Derived::Base::f(int)` or restructure the class inheritance using `using Base::f;` inside the `Derived` class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
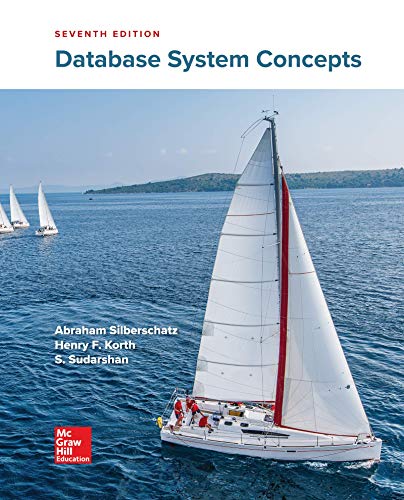
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
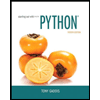
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
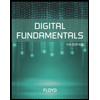
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
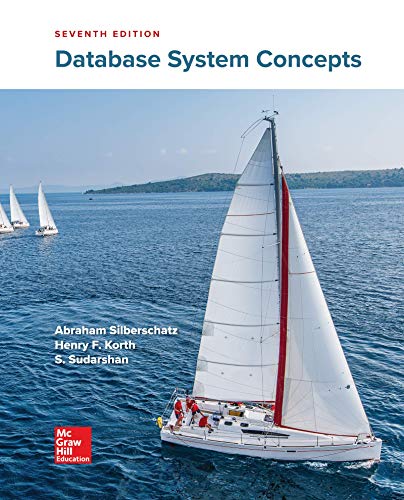
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
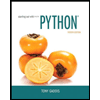
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
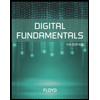
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
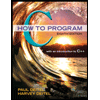
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
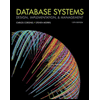
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
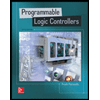
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education