What are the core algorithms used in the code below? Provide examples for small inputs (This code Takes a year and a month as input. Prints 28-31 days paired with the corresponding days of the week. Takes into account leap years. Keeps logs of user input and resulting calendars) # Tuples list for each month along with their date ranges calendar = [('January', 31), ('Feburary', 28), ('March', 31), ('April', 30), ('May', 31), ('June', 30), ('July', 31), ('August', 31), ('September', 30), ('October', 31), ('November', 30), ('December', 31)] # Days of the week week = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'] def create_calendar(year, start_day): # Determines the current starting position on the calendar start_point = week.index(start_day) # If leap year occurs, Feburary date range changes to 29 if leap_year(year): calendar[1] = ('Feburary', 29) for month, days in calendar: print('{0} {1}'.format(month, year).center(25, ' ')) # Prints Month Titile print(' '.join(['{0:<3}'.format(w) for w in week])) # Prints Day Headings print('{0:<4}'.format('') * start_point, end='') # Adds spacing for non-zero starting point for day in range(1, days + 1): print('{0:<4}'.format(day), end='') # Prints day number start_point += 1 if start_point == 7: # If the starting point is sunday (7), start a new line print() start_point = 0 # Will reset counter print('\n') def leap_year(year): # Checks if a year is a leap year if year % 4 == 0: if year % 100 == 0: if year % 400 == 0: return True else: return False else: return True else: return False year = int(input('Enter The Year: \n')) print() if year <= 0: # If number inputed is less than or equal to zero, creates 'Invalid Input' print('Invlid Input') import sys sys.exit() start_day = input('Enter Start Day Of The Year: Sun, Mon, Tue, Wed, Thu, Fri, Sat: \n',) print() create_calendar(year,start_day)
What are the core algorithms used in the code below? Provide examples for small inputs (This code Takes a year and a month as input. Prints 28-31 days paired with the corresponding days of the week. Takes into account leap years. Keeps logs of user input and resulting calendars) # Tuples list for each month along with their date ranges calendar = [('January', 31), ('Feburary', 28), ('March', 31), ('April', 30), ('May', 31), ('June', 30), ('July', 31), ('August', 31), ('September', 30), ('October', 31), ('November', 30), ('December', 31)] # Days of the week week = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'] def create_calendar(year, start_day): # Determines the current starting position on the calendar start_point = week.index(start_day) # If leap year occurs, Feburary date range changes to 29 if leap_year(year): calendar[1] = ('Feburary', 29) for month, days in calendar: print('{0} {1}'.format(month, year).center(25, ' ')) # Prints Month Titile print(' '.join(['{0:<3}'.format(w) for w in week])) # Prints Day Headings print('{0:<4}'.format('') * start_point, end='') # Adds spacing for non-zero starting point for day in range(1, days + 1): print('{0:<4}'.format(day), end='') # Prints day number start_point += 1 if start_point == 7: # If the starting point is sunday (7), start a new line print() start_point = 0 # Will reset counter print('\n') def leap_year(year): # Checks if a year is a leap year if year % 4 == 0: if year % 100 == 0: if year % 400 == 0: return True else: return False else: return True else: return False year = int(input('Enter The Year: \n')) print() if year <= 0: # If number inputed is less than or equal to zero, creates 'Invalid Input' print('Invlid Input') import sys sys.exit() start_day = input('Enter Start Day Of The Year: Sun, Mon, Tue, Wed, Thu, Fri, Sat: \n',) print() create_calendar(year,start_day)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
What are the core
Provide examples for small inputs
(This code Takes a year and a month as input. Prints 28-31 days paired with the corresponding days of the week. Takes into account leap years. Keeps logs of user input and resulting calendars)
# Tuples list for each month along with their date ranges
calendar = [('January', 31), ('Feburary', 28), ('March', 31),
('April', 30), ('May', 31), ('June', 30),
('July', 31), ('August', 31), ('September', 30),
('October', 31), ('November', 30), ('December', 31)]
# Days of the week
week = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat']
def create_calendar(year, start_day):
# Determines the current starting position on the calendar
start_point = week.index(start_day)
# If leap year occurs, Feburary date range changes to 29
if leap_year(year):
calendar[1] = ('Feburary', 29)
for month, days in calendar:
print('{0} {1}'.format(month, year).center(25, ' ')) # Prints Month Titile
print(' '.join(['{0:<3}'.format(w) for w in week])) # Prints Day Headings
print('{0:<4}'.format('') * start_point, end='') # Adds spacing for non-zero starting point
for day in range(1, days + 1):
print('{0:<4}'.format(day), end='') # Prints day number
start_point += 1
if start_point == 7: # If the starting point is sunday (7), start a new line
print()
start_point = 0 # Will reset counter
print('\n')
def leap_year(year):
# Checks if a year is a leap year
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
return True
else:
return False
else:
return True
else:
return False
year = int(input('Enter The Year: \n'))
print()
if year <= 0: # If number inputed is less than or equal to zero, creates 'Invalid Input'
print('Invlid Input')
import sys
sys.exit()
start_day = input('Enter Start Day Of The Year: Sun, Mon, Tue, Wed, Thu, Fri, Sat: \n',)
print()
create_calendar(year,start_day)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
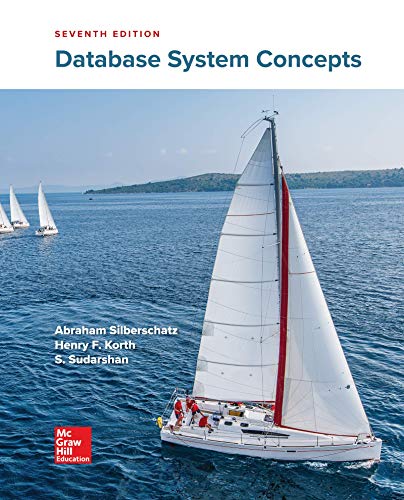
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
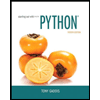
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
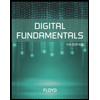
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
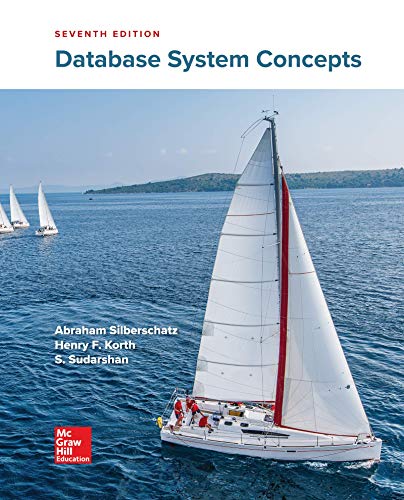
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
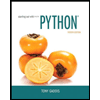
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
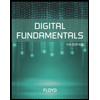
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
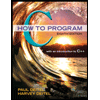
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
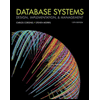
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
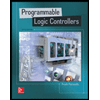
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education