We want to develop a delivery vehicle that can carry a limited number of equally weighted boxes. To do this we can build off of our previous task by extending the BasicVehicle class. Instructions 1. Create a new class called DeliveryVehicle that is a subclass of BasicVehicle: • a private attribute cargoList of type String[] • a private attribute numItems of type int 2. Create a constructor that takes two parameters: a String for registration, and an int for maxCapacity: • pass on the registration parameter to the super constructor along with 0.0 for altitude, longitude and latitude. • use the maxCapacity parameter to create a new array for cargoList 3. Create a boolean method called isFull that returns if the cargoList is full. 4. Create a boolean method called isEmpty that returns if there are no boxes loaded.
Task 2: Delivery Vehicle
We want to develop a delivery vehicle that can carry a limited number of equally weighted boxes. To do this we can build off of our previous task by extending the BasicVehicle class.
Instructions
1. Create a new class called DeliveryVehicle that is a subclass of BasicVehicle:
• a private attribute cargoList of type String[]
• a private attribute numItems of type int
2. Create a constructor that takes two parameters:
a String for registration, and an int for maxCapacity:
• pass on the registration parameter to the super constructor along with 0.0 for altitude, longitude and latitude.
• use the maxCapacity parameter to create a new array for cargoList
3. Create a boolean method called isFull that returns if the cargoList is full.
4. Create a boolean method called isEmpty that returns if there are no boxes loaded.
5. Create a method called loadCargo that takes a String parameter description and adds the cargo description to the cargo list (if it is not already full).
6. Override the printInfo() method to print out the cargo list in addition to the BasicVehicle info or “No cargo yet.” if the list is empty
Hint: The cargoList should be treated as a partially filled array using the numItems attribute.
Sample Input/Output
The following class can be used to test your assignment and produce the output below
public class RunAssignment {
public static void main(String[] args) {
BasicVehicle vehicleCS1 = new BasicVehicle();
vehicleCS1.setRegistration("ZU-CS1");
vehicleCS1.setAltitude(18000.0);
vehicleCS1.setLatitude(22.0);
vehicleCS1.setLongitude(31.0);
vehicleCS1.printInfo();
BasicVehicle vehicleC0S = new BasicVehicle("ZU-C0S", 10.0, 18.0, 19000.0);
vehicleC0S.printInfo(); System.out.println("Distance between vehicles: " + vehicleCS1.distanceTo(vehicleC0S)); DeliveryVehicle deliveryCA1 = new DeliveryDrone("ZU-CA1", 5);
deliveryCA1.printInfo();
System.out.println("Delivery vehicle is empty: " + deliveryCA1.isEmpty());
System.out.println("Delivery vehicle is full: " + deliveryCA1.isFull()); deliveryCA1.loadDelivery("50000 units paracetamol 500mg");
deliveryCA1.loadDelivery("2000 units melatonin 5mg");
deliveryCA1.printInfo();
System.out.println("Delivery vehicle is empty: " + deliveryCA1.isEmpty());
System.out.println("Delivery vehicle is full: " + deliveryCA1.isFull()); } }
Output:
Registration: ZU-CS1
Current position:
Alt: 18000.0
Lat: 22.0
Long: 31.0
Registration: ZU-C0S
Current position:
Alt: 19000.0
Lat: 10.0
Long: 18.0
Distance between vehicles: 1000.1564877557911
Delivery vehicle: ZU-CA1
Max capacity: 5
No cargo yet.
Delivery vehicle is empty: true
Delivery vehicle is full: false
Delivery vehicle: ZU-CA1
Max capacity: 5
50000 units paracetamol 500mg
2000 units melatonin 5mg
Delivery vehicle is empty: false
Delivery vehicle is full: false

Step by step
Solved in 3 steps

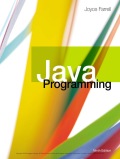
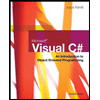
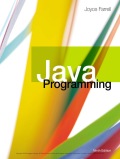
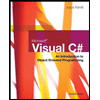