We need to create a method "reverse" which will have as input a string say sx and return a string which contains the reverse of sx. Ex: sx="Victor", the reverse of sx is : "rotciV". Remember that the "reverse" method is written from scratch. That means you cannot use another method already provided by the String method or use the StringBuilder library provided by the java compiler. Here is a skeleton of a program that I called Final_33. Please remember that the reverse() method below is where you write your code. public class Final_33 { public static void main(String[] args) { Scanner input = new Scanner(System.in); Final_33 r = new Final_33(); System.out.print("Enter the string you want to reverse: "); String s1 = input.nextLine(); System.out.println("Original String is: " + s1); System.out.println("Reverse of the string " + s1 + " is: " + r.reverse (s1)); } public String reverse(String sx) { // fill in the code here } }
We need to create a method "reverse" which will have as input a string say sx and return a string which contains the reverse of sx.
Ex: sx="Victor", the reverse of sx is : "rotciV".
Remember that the "reverse" method is written from scratch. That means you cannot use another method already provided by the String method or use the StringBuilder library provided by the java compiler.
Here is a skeleton of a program that I called Final_33. Please remember that the reverse() method below is where you write your code.
public class Final_33 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Final_33 r = new Final_33();
System.out.print("Enter the string you want to reverse: ");
String s1 = input.nextLine();
System.out.println("Original String is: " + s1);
System.out.println("Reverse of the string " + s1 + " is: " + r.reverse (s1));
}
public String reverse(String sx)
{
// fill in the code here
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

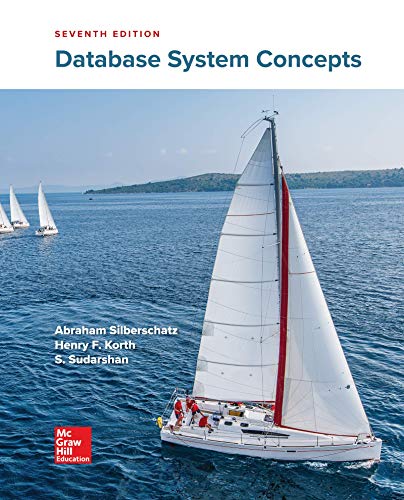
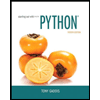
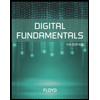
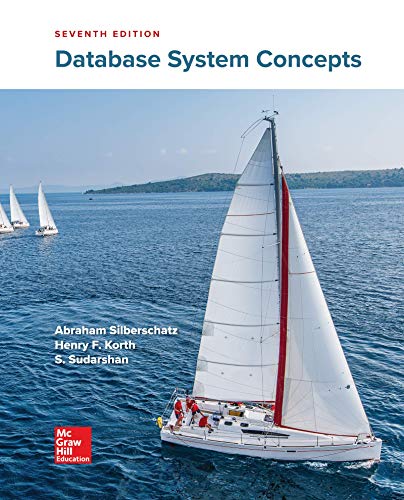
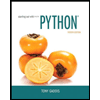
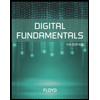
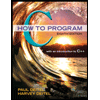
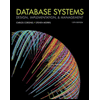
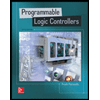