We learned about the standard (i.e. not in place) ways to perform set operations. Using any of those as needed, create a small program to take the two dictionaries below and (1) finding all keys used in the two, and (2) finding which keys are in one dictionary but not the other. admissions_record 'first': 'Julia', 'last': 'Brown', 'id': 'AX012E4' student record = = 'admitted':'2020-05-15'} { " { 'first': 'Julia', 'last': 'Brown' " 'id': 'AX012E4', 'major': 'Data Science', 'minor': 'Mathematics' I 'advisor':'Franklin, Jack'}
Use Python to solve:


1. Input: Two dictionaries `admissions_record` and `student_record`.
2. Initialize an empty set `all_keys` to store all keys used in both dictionaries.
3. Initialize two empty sets `keys_in_admissions_record` and `keys_in_student_record` to store the keys specific to each dictionary.
4. Loop through the keys in `admissions_record`:
- Add each key to the `keys_in_admissions_record` set.
- Add each key to the `all_keys` set.
5. Loop through the keys in `student_record`:
- Add each key to the `keys_in_student_record` set.
- Add each key to the `all_keys` set.
6. Calculate the keys that are only in `admissions_record` by subtracting `keys_in_student_record` from `keys_in_admissions_record`. Store the result in `keys_only_in_admissions`.
7. Calculate the keys that are only in `student_record` by subtracting `keys_in_admissions_record` from `keys_in_student_record`. Store the result in `keys_only_in_student`.
8. Print the following results:
- "All keys used in both dictionaries:" followed by the `all_keys` set.
- "Keys only in admissions_record:" followed by the `keys_only_in_admissions` set.
- "Keys only in student_record:" followed by the `keys_only_in_student` set.
9. End.
Step by step
Solved in 4 steps with 1 images

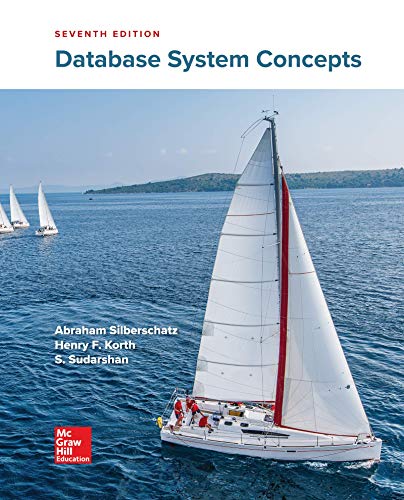
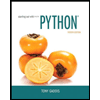
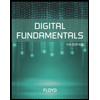
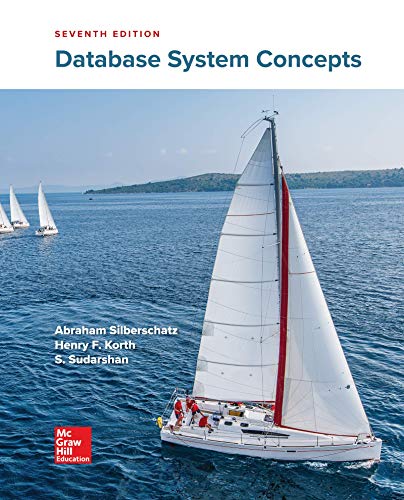
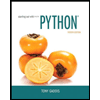
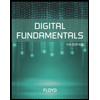
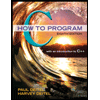
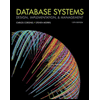
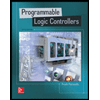