Using your code from the previous question, write a program that reads a m
Using your code from the previous question, write a program that reads a multi-line message up to the first full stop and then modifies the contents of the message, so that all whitespace characters are removed. Finally,it should print out the modified message using a single printf statement.
If the user types:
A simple sentence.
the program should output
Asimplesentence.
For the purposes of this question, a whitespace character is either a space, a tab or a newline. Note in particular that the sentence might be spread over several lines.
For example:
Input | Result |
---|---|
A simple sentence. | Asimplesentence. |
A sentence with a newline. | Asentencewithanewline. |
Code i was given:
#include <stdio.h>
#include <stdbool.h>
#define MAX_MSG_LEN 256
int main(void)
{
int i = 0;
char msg[MAX_MSG_LEN+1];
/* insert appropriate variable declarations */
/* read the message from the concole up to first full stop */
/* loop through the message and keep only non-whitespace characters */
i = 0;
while (msg[i]!='\0')
{
/* only keep a character if it is not a whitespace */
if (/* insert appropriate guard */) {
/* assign character into modified message */
}
i++;
}
/* DO NOT modify print statement */
printf("%s\n", msg);
return 0;
}

Step by step
Solved in 3 steps with 1 images

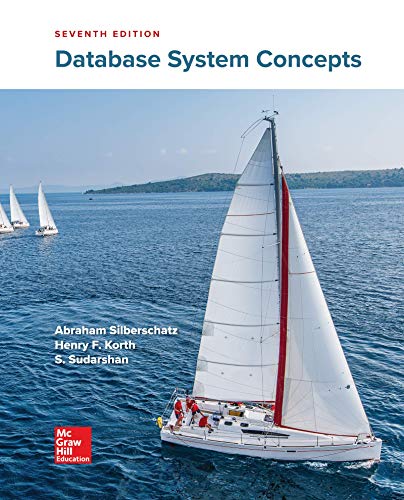
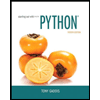
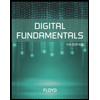
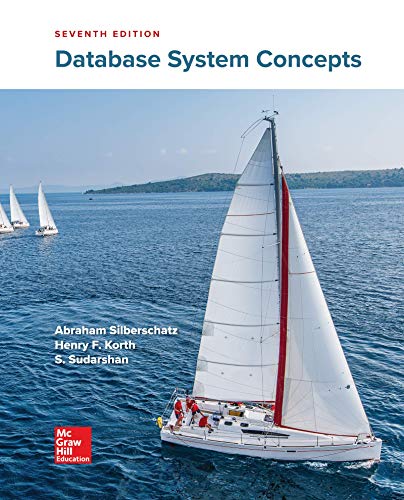
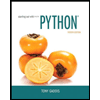
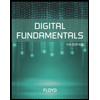
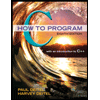
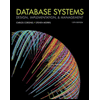
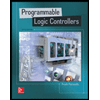