Program Specifications: Write a program to calculate U.S. income tax owed given wages, taxable interest, unemployment compensation, status (single or married), and taxes withheld. Taxpayers are only allowed to use this short form if adjusted gross income (AGI) is less than $120000. Dollar amounts are displayed as integers with no comma separators. For example, cout << "Deduction: $" << deduction Step 1 . Input wages, taxable interest, unemployment compensation, status (1=single and 2=married), and taxes withheld as integers. Calculate and output AGI (wages + interest + unemployment). Output error message if AGI is above $120000 and the program stops with no additional output. Submit for grading to confirm two tests pass. Ex: If the input is: 20000 23 500 1 400 The output is: AGI: $20523 Ex: If the input is: 120000 23 500 1 400 The output is: AGI: $120523 Error: Income too high to use this form Step 2. Identify deduction amount based on status: (1) Single=$12000 or (2) Married=$24000. Set status to 1 if not input as 1 or 2. Calculate taxable income (AGI - deduction). Set taxable income to zero if negative. Output deduction and taxable income. Submit for grading to confirm five tests pass. Ex: If the input is: 20000 23 500 1 400 Ex: The additional output is: AGI: $20523 Deduction: $12000 Taxable income: $8523 Step 3. Calculate tax amount based on exemption and taxable income (see tables below). The tax amount should be stored as a double and rounded to the nearest whole number using round(). Submit for grading to confirm eight tests pass. Ex: If the input is: 20000 23 500 1 400 Ex: The additional output is: AGI: $20523 Deduction: $12000 Taxable income: $8523 Federal tax: $852 Step 4. Calculate the amount of tax due (tax - withheld). If the amount due is negative the taxpayer receives a refund. Output tax due or tax refund as positive values. Submit for grading to confirm all tests pass. Ex: If the input is: 80000 0 500 2 12000 Ex: The additional output is: AGI: $80500 Deduction: $24000 Taxable income: $56500 Federal tax: $6380 Tax refund: $5620
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
PLEASE ANSWER USING C++
Step 1 . Input wages, taxable interest, unemployment compensation, status (1=single and 2=married), and taxes withheld as integers. Calculate and output AGI (wages + interest + unemployment). Output error message if AGI is above $120000 and the program stops with no additional output. Submit for grading to confirm two tests pass.
Ex: If the input is:
20000 23 500 1 400
The output is:
AGI: $20523
Ex: If the input is:
120000 23 500 1 400
The output is:
AGI: $120523
Error: Income too high to use this form
Step 2. Identify deduction amount based on status: (1) Single=$12000 or (2) Married=$24000. Set status to 1 if not input as 1 or 2. Calculate taxable income (AGI - deduction). Set taxable income to zero if negative. Output deduction and taxable income. Submit for grading to confirm five tests pass.
Ex: If the input is:
20000 23 500 1 400
Ex: The additional output is:
AGI: $20523
Deduction: $12000
Taxable income: $8523
Step 3. Calculate tax amount based on exemption and taxable income (see tables below). The tax amount should be stored as a double and rounded to the nearest whole number using round(). Submit for grading to confirm eight tests pass.
Ex: If the input is:
20000 23 500 1 400
Ex: The additional output is:
AGI: $20523
Deduction: $12000
Taxable income: $8523 Federal tax: $852
Step 4. Calculate the amount of tax due (tax - withheld). If the amount due is negative the taxpayer receives a refund. Output tax due or tax refund as positive values. Submit for grading to confirm all tests pass.
Ex: If the input is:
80000 0 500 2 12000
Ex: The additional output is:
AGI: $80500
Deduction: $24000
Taxable income: $56500
Federal tax: $6380
Tax refund: $5620



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Using the follow-up answer provided I am getting more errors than before. Can you please fix the errors? Thank you.
This problem has now cost me 3 questions.
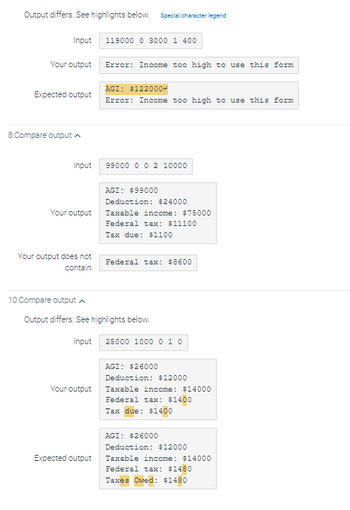
USING C++ can you please fix according to the errors received using your code? Thank you.
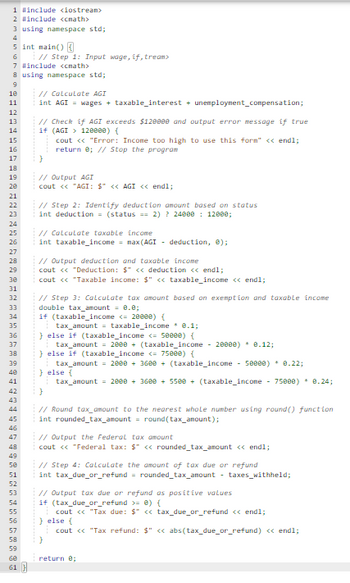
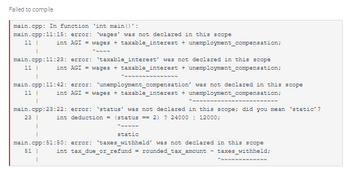
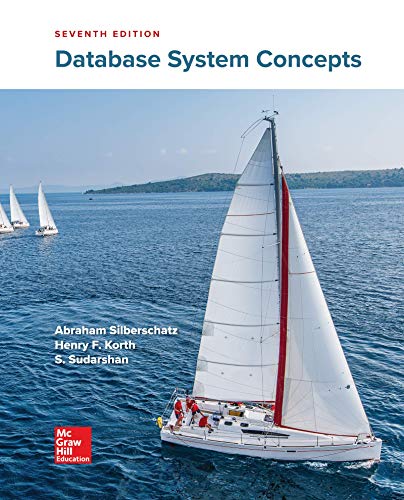
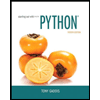
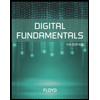
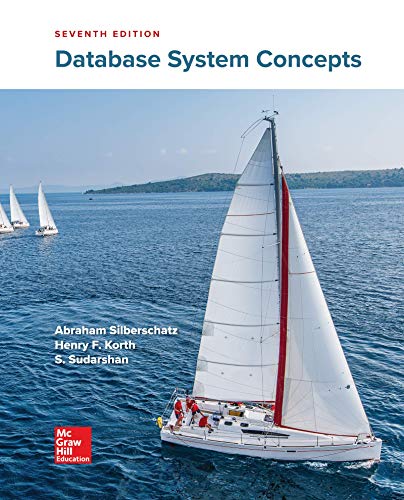
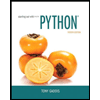
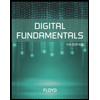
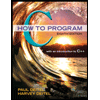
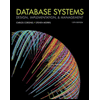
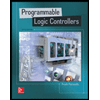