using System; using System.Collections.Generic; namespace DocumentStore.Question // remove semi colon from here and put starting { and ending } at the end of the code { /// /// ! IMPORTANT ! /// The following conditions must be
//The tostring method is not returning proper results
//Please Use C#
using System;
using System.Collections.Generic;
namespace DocumentStore.Question // remove semi colon from here and put starting { and ending } at the end of the code
{
/// <summary>
/// ! IMPORTANT !
/// The following conditions must be satisfied:
/// - The documents property show return the contents of the documents store.
/// This content should be readonly, ensure that there is no way to edit the data.
/// - The `AddDocument(string document)` function should add a new document to the store.
/// If the store is full, an InvalidOperationException should be thrown.
/// - The ToString() function should return the document store's description in the format of
/// "Document store: number of documents/capacity"
/// For example: if the store contains 1 document, and the capacity is 2, the expected output is:
/// "Document store: 1/2"
/// - If a document is a duplicate, it will be ignored when being added.
///
/// Please identify and fix any/all issues with the code!
/// </summary>
public class DocumentStoreService
{
/// create A list of `documents`. Which are just strings for the purpose of this example.
/// <summary>
/// A list of `documents`. Which are just strings for the purpose of this example.
/// </summary>
private readonly List<string> documents = new List<string>();
/// <summary>
/// The maximum capacity allowed in the document store.
/// </summary>
private readonly int capacity;
public DocumentStoreService(int capacity)
{
this.capacity = capacity; // both variables have same name - compiler will get confused and give error
}
public int Capacity { get { return capacity; } }
public IEnumerable<string> Documents
{
get { return documents; } // returning the contents
}
public void AddDocument(string document)
{
if (documents.Capacity > capacity) // capacity is variable while Capacity is property of list
throw new InvalidOperationException();
var isDuplicated = false; // Would we set this to false to start off with?
for(int i=0; i<documents.Capacity; i++)
{
for(int j=1; j<documents.Capacity; j++)
{
if (documents[i] == documents[j]) // both for loop are the same, they need to be different
{
isDuplicated = true;
}
}
}
if (!isDuplicated)
{
documents.Add(document);
}
}
public override string ToString()
{
return $"Document store: " + (documents.Capacity / capacity); // incorrect placement of quotes
}
}
// class having main() method
class Test
{
public static void Main(string[]args)
{
DocumentStoreService ob = new DocumentStoreService(2);
ob.AddDocument("1");
//ob.AddDocument("2");
// ob.AddDocument("3");
Console.WriteLine(ob.ToString());
}
}
} // namespace ends

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

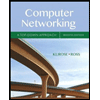
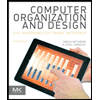
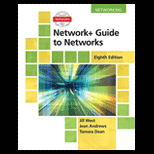
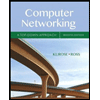
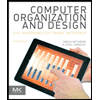
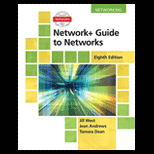
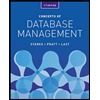
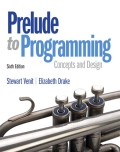
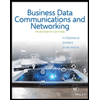