Using Kotlin in Android Studio Create 4 big buttons that fill the screen Change the background colour of all the other buttons except the button you clicked. Colour changes between two colours. Initialize the buttons with one colour. I have the below code. I would like to have it so that if you then pressed another button it changed the other buttons again and so on. I assume I should use an if statement where depending on the color of the active button the others changed to the opposite, but I have tried that and I can not get it to work as I can not find how to get it to pick up the background colour. MainActivity.kt import android.graphics.Color import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.widget.Button class MainActivity : AppCompatActivity() { lateinit var b1: Button lateinit var b2: Button lateinit var b3: Button lateinit var b4: Button override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) b1 = findViewById(R.id.button1); btn1.setBackgroundColor(getColor(R.color.black)) b2 = findViewById(R.id.button2); btn2.setBackgroundColor(getColor(R.color.black)) b3 = findViewById(R.id.button3); btn3.setBackgroundColor(getColor(R.color.black)) b4 = findViewById(R.id.button4); btn4.setBackgroundColor(getColor(R.color.black)) b1.setOnClickListener { b2.setBackgroundColor(getColor(R.color.teal_200)) b3.setBackgroundColor(getColor(R.color.teal_200)) b4.setBackgroundColor(getColor(R.color.teal_200)) } b2.setOnClickListener{ btn1.setBackgroundColor(getColor(R.color.teal_200)) btn3.setBackgroundColor(getColor(R.color.teal_200)) btn4.setBackgroundColor(getColor(R.color.teal_200)) } b3.setOnClickListener{ b1.setBackgroundColor(getColor(R.color.teal_200)) b2.setBackgroundColor(getColor(R.color.teal_200)) b4.setBackgroundColor(getColor(R.color.teal_200) } b4.setOnClickListener{ b1.setBackgroundColor(getColor(R.color.teal_200)) b2.setBackgroundColor(getColor(R.color.teal_200)) b3.setBackgroundColor(getColor(R.color.teal_200)) } } } activity_main.xml
Using Kotlin in Android Studio
Create 4 big buttons that fill the screen
Change the background colour of all the other buttons except the button you clicked.
Colour changes between two colours.
Initialize the buttons with one colour.
I have the below code. I would like to have it so that if you then pressed another button it changed the other buttons again and so on. I assume I should use an if statement where depending on the color of the active button the others changed to the opposite, but I have tried that and I can not get it to work as I can not find how to get it to pick up the background colour.
MainActivity.kt
import android.graphics.Color
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
class MainActivity : AppCompatActivity() {
lateinit var b1: Button
lateinit var b2: Button
lateinit var b3: Button
lateinit var b4: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
b1 = findViewById(R.id.button1); btn1.setBackgroundColor(getColor(R.color.black))
b2 = findViewById(R.id.button2); btn2.setBackgroundColor(getColor(R.color.black))
b3 = findViewById(R.id.button3); btn3.setBackgroundColor(getColor(R.color.black))
b4 = findViewById(R.id.button4); btn4.setBackgroundColor(getColor(R.color.black))
b1.setOnClickListener {
b2.setBackgroundColor(getColor(R.color.teal_200))
b3.setBackgroundColor(getColor(R.color.teal_200))
b4.setBackgroundColor(getColor(R.color.teal_200))
}
b2.setOnClickListener{
btn1.setBackgroundColor(getColor(R.color.teal_200))
btn3.setBackgroundColor(getColor(R.color.teal_200))
btn4.setBackgroundColor(getColor(R.color.teal_200))
}
b3.setOnClickListener{
b1.setBackgroundColor(getColor(R.color.teal_200))
b2.setBackgroundColor(getColor(R.color.teal_200))
b4.setBackgroundColor(getColor(R.color.teal_200)
}
b4.setOnClickListener{
b1.setBackgroundColor(getColor(R.color.teal_200))
b2.setBackgroundColor(getColor(R.color.teal_200))
b3.setBackgroundColor(getColor(R.color.teal_200))
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_margin="3dp"
android:textColor="@color/white"
android:text="Button1"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toStartOf="@+id/button2"
app:layout_constraintBottom_toTopOf="@id/button3"/>
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_margin="3dp"
android:textColor="@color/white"
android:text="Button2"
app:layout_constraintStart_toEndOf="@+id/button1"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toTopOf="@+id/button4"/>
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_margin="3dp"
android:textColor="@color/white"
android:text="Button3"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/button4"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1"/>
<Button
android:id="@+id/button4"
android:layout_width="0dp"
android:layout_height="0dp"
android:textColor="@color/white"
android:text="Button4"
android:layout_margin="3dp"
app:layout_constraintTop_toBottomOf="@id/button2"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/button3" />
</androidx.constraintlayout.widget.ConstraintLayout>

Step by step
Solved in 2 steps

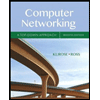
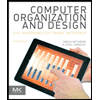
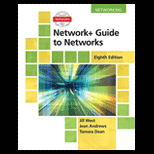
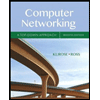
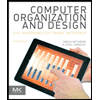
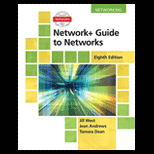
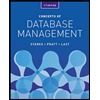
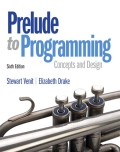
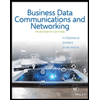