Using gaussian elimination and backwards substitution, solve for the x terms in the augmented matrix below: 2 4 -2 x1 3 -1 5 -2 1 1 1 2 3 ✓ [Choose ]
import numpy as np
np.set_printoptions(precision=7, suppress=True, linewidth=100)
def gauss_jordan(A, b):
n = len(b)
# Combine A and b into augmented matrix
Ab = np.concatenate((A, b.reshape(n,1)), axis=1)
# Perform elimination
for i in range(n):
# Find pivot row
max_row = i
for j in range(i+1, n):
if abs(Ab[j,i]) > abs(Ab[max_row,i]):
max_row = j
# Swap rows to bring pivot element to diagonal
Ab[[i,max_row], :] = Ab[[max_row,i], :] # operation 1 of row operations
# Divide pivot row by pivot element
pivot = Ab[i,i]
Ab[i,:] = Ab[i,:] / pivot
# Eliminate entries below pivot
for j in range(i+1, n):
factor = Ab[j,i]
Ab[j,:] -= factor * Ab[i,:] # operation 2 of row operations
# Perform back-substitution
for i in range(n-1, -1, -1):
for j in range(i-1, -1, -1):
factor = Ab[j,i]
Ab[j,:] -= factor * Ab[i,:]
# Extract solution
x = Ab[:,n]
return x
if __name__ == "__main__":
A = np.array([[2,3,-1],
[4,-2,1],
[-2,1,2]])
b = np.array([5,1,3])
x = gauss_jordan(A, b)
print(x)
![Using gaussian elimination and backwards substitution, solve for the x terms in the augmented
matrix below:
2
4
- 2
x1
x2
x3
3
-2
2
1
-1 5
1
1
2
3
✓ [Choose ]
2.0
1.65
.952
1.1
.725
1.4
4 pts](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fce515de5-1fac-4346-b9b4-b377b97902d4%2F8260f427-2446-46ff-b65b-6c34ac26d995%2Fi9jrq6_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 30 images

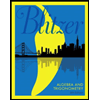
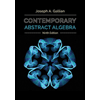
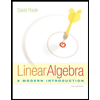
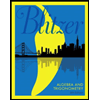
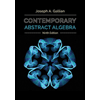
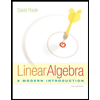
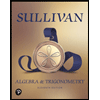
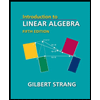
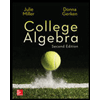