Use stack to convert the decimal digit to octal digit. Define a function of conversion, create a stack, read N and push it into stack. Get the remainder of N%8 and push into stack. Go on the %8 until the remainder is 0. Pop the remainder and get the octal digit. Header file #define MAXSIZE 100 typedef int Elemtype; typedef struct { Elemtype stack[MAXSIZE]; int top; }Sstack; Source file #include #include #include #include "stack.h" void initialize(Sstack *L)//initialize a stack {//initiate a stack ; } int stackIsEmpty(Sstack *L)//check if the stack is empty. If it is empty, return 1, else return 0. {//determine an empty stack ; ; } int push(Sstack *L, Elemtype x)//Push element into stack. If the stack is full, hint the user and return 0. Else, push the element into the stack and return 1. {//push element x into stack ; ; } Elemtype pop(Sstack *L)//Stack pop:if the stack is empty return 0,else pop an element and return the value of poped element. {//pop an element Elemtype a; ; ; } Elemtype getTop(Sstack *L)//read and return the value of the top element. {//get the top element ; } void conversion( )//Number conversion { ElemType e; Sstack *L; ;//allocate stack memory for L ;//Initialize L; int N; printf("Please input a number to be converted\n"); scanf("%d",&N); while(N)//When N is not 0 { ; ; } printf("The number converted into octal form is\n");//output the changed number ; } void main()//main function { conversion( ); }
Use stack to convert the decimal digit to octal digit. Define a function of conversion, create a stack, read N and push it into stack. Get the remainder of N%8 and push into stack. Go on the %8 until the remainder is 0. Pop the remainder and get the octal digit.
Header file
#define MAXSIZE 100
typedef int Elemtype;
typedef struct
{
Elemtype stack[MAXSIZE];
int top;
}Sstack;
Source file
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include "stack.h"
void initialize(Sstack *L)//initialize a stack
{//initiate a stack
;
}
int stackIsEmpty(Sstack *L)//check if the stack is empty. If it is empty, return 1, else return 0.
{//determine an empty stack
;
;
}
int push(Sstack *L, Elemtype x)//Push element into stack. If the stack is full, hint the user and return 0. Else, push the element into the stack and return 1.
{//push element x into stack
;
;
}
Elemtype pop(Sstack *L)//Stack pop:if the stack is empty return 0,else pop an element and return the value of poped element.
{//pop an element
Elemtype a;
;
;
}
Elemtype getTop(Sstack *L)//read and return the value of the top element.
{//get the top element
;
}
void conversion( )//Number conversion
{
ElemType e;
Sstack *L;
;//allocate stack memory for L
;//Initialize L;
int N;
printf("Please input a number to be converted\n");
scanf("%d",&N);
while(N)//When N is not 0
{
;
;
}
printf("The number converted into octal form is\n");//output the changed number
;
}
void main()//main function
{
conversion( );
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

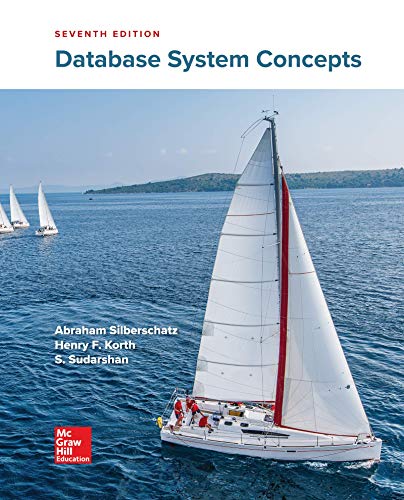
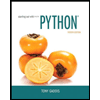
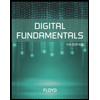
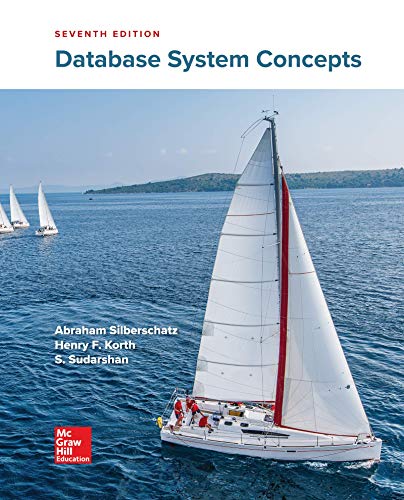
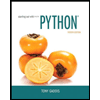
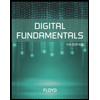
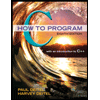
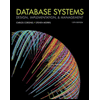
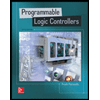