Use relational and logical operators to make decisions using selection structure Use switch statement to design a simple menu selection decision
Use relational and logical operators to make decisions using selection structure Use switch statement to design a simple menu selection decision
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use relational and logical operators to make decisions using selection structure
Use switch statement to design a simple menu selection decision

Transcribed Image Text:Task 2 Sample Output
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 5
Invalid selection!
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 4
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 1
Radius of circle (m) : -1
Negative value is not allowed
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 2
Enter length of rectangle (m) : 4
Enter width of rectangle (m) : -1
Negative value is not allowed
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 1
Enter radius of circle (m) : 6.2
The area of the circle is: 120.76 m^2
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 3
Enter base of triangle (m): 5.5
Enter height of triangle (m) : 9
The area of the triangle is: 24.75 m^2

Transcribed Image Text:Write a program GeometryCalculator.cpp that displays the following menu:
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1-4) :
The menu must be constructed using the switch statement.
If the user enters 1, the program should ask for the radius of the circle then display its area. Use the
following formula:
area = n xX r2
Use 3.14159 for a and the radius of the circle for r.
If the user enters 2, the program should ask for the length and width of the rectangle, then display
the rectangle's area. Use the following formula:
area = length x width
If the user enters 3, the program should ask for the length of the triangle's base and its height, then
display its area. Use the fo following formula:
area = base x height x 0.5
If the user enters 4, the program should end.
Input validation: Display an error message if the user enters a number outside the range of 1
through 4 when selecting an item from the menu. Do not accept negative values for the circle's
radius, the rectangle's length or width, or the triangle's base or height. Terminate the program if
the user enters invalid input.
Task 2 Sample Output
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 5
Invalid selection!
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 4
Geometry Calculator:
1. Calculate the Area of a Circle
2. Calculate the Area of a Rectangle
3. Calculate the Area of a Triangle
4. Quit
Enter your choice (1 - 4): 1
Radius of circle (m) : -1
Negative value is not allowed
Expert Solution

Explaination
Working of the program:-
- I keep the switch inside the while loop
- While loop will keep running until choice of the user is 4 . When choice becomes 4 user exit.
- I had created three separate functions in order to calculate the area they return the double value area.
- After taking the input we have if to check if the value entered is greater than 0 and not negative
- If negative we just give the false message and break
- Else we call the function if all the values are postive and print the results.
- After calling the function in switch statment i store the output in the local area variable and then display it.
- Default case give errror message in case intput is not among 1,2,3 and 4.
Everything is mentioned in the code comments
Code and output is provided in the step 2
Also screenshots of the both are added .
Step by step
Solved in 2 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
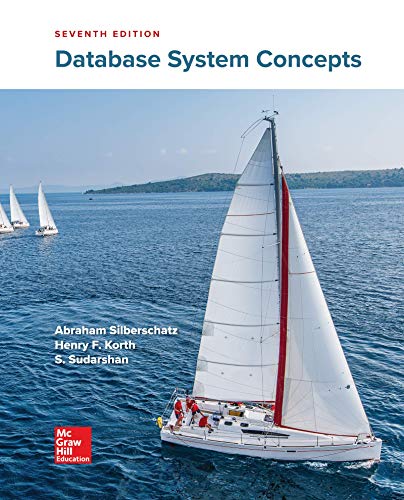
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
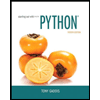
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
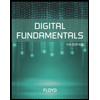
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
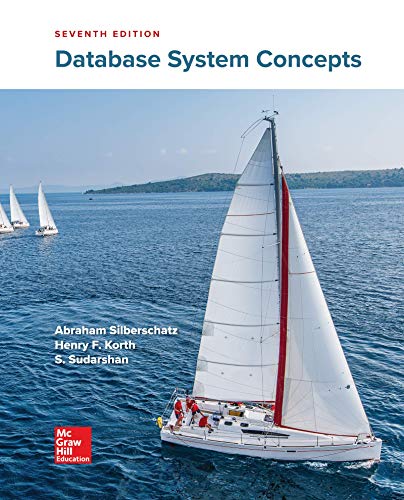
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
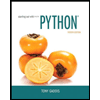
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
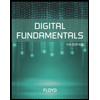
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
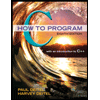
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
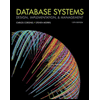
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
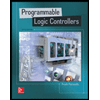
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education