USE C++ OR JAVA ---- POST SOURCE CODE WILL DOWNVOTE FOR INCORRECT ANSWER WILL REPORT FOR REPEAT ANSWER -------------------------------------------------------------------------------------------------------------------- Create a simple program that plays poker 1000 times with the following rules: Each time the program will: Shuffle the 52-card deck and deal yourself 5 cards. Using the remaining 47 cards, deal other 5 players their 5 cards. Determine if your hand would win or lose compared to the others. (Poker hands ranking listed below for reference) Record how many games out of 1000 you won. For each rank of hand, report the percentage of hands having that rank, and the average winning percentage for each rank (out of those 1000 games).
USE C++ OR JAVA ---- POST SOURCE CODE
WILL DOWNVOTE FOR INCORRECT ANSWER
WILL REPORT FOR REPEAT ANSWER
--------------------------------------------------------------------------------------------------------------------
Create a simple program that plays poker 1000 times with the following rules:
Each time the program will:
- Shuffle the 52-card deck and deal yourself 5 cards.
- Using the remaining 47 cards, deal other 5 players their 5 cards.
- Determine if your hand would win or lose compared to the others. (Poker hands ranking listed below for reference)
- Record how many games out of 1000 you won.
- For each rank of hand, report the percentage of hands having that rank, and the average winning percentage for each rank (out of those 1000 games).
--------------------------------------------------------------------------------------------------------------------
Your program will produce 2 output files (1000 entries):
- A session log output as a CSV (comma-separated value) file, with each hand (you and 5 other hands) on a separate line.
- For each hand: Output the cards in the hand; what the hand was evaluated as; and its winning percentage.
- A summary showing the percentage of hands falling into each rank, and the overall win percentage for each rank, as a ‘normal’ text file.
- Don’t just list the percentages; add enough text to make it reader-friendly.
--------------------------------------------------------------------------------------------------------------------
The system random-number generator (random() and related functions) for the most common development environments will be more than adequate for this project.
Picture reference for card rankings:



import java.util.Arrays
import java.util.Scanner;
/**
Program: Poker
*/
public final class Poker {
String[] suits = {"Hearts", "Diamonds", "Spades", "Clubs"};
char[] value = {'2', '3', '4', '5', '6', '7', '8', '9', 'T', 'J', 'Q', 'K', 'A'};
// check cards in deck
char[][] cards = new char[13][4];
// array for [player][card][suit/val]
int[][][] pc = new int[2][5][2];
// comp and players hand, determines winner
int[] hand = new int[2];
int[] handVal = new int[2];
// for ai to complete without hand exposed
boolean aiDone = false;
// user input
Scanner input = new Scanner(System.in);
// for betting
int money = 100;
int bet = 0;
public void clear() {
System.out.println("\n\n");
}// end of clear
/**
* Make a bet
*/
public void makeBet() {
// check if money is available
if (money <= 0) {
System.out.println("You have no money!!!");
} else {
do {
System.out.print("You currently have $" + money
+ ".\nPlease place a bet:");
bet = input.nextInt();// user placed bet
} while (!valid(bet, money, 0));
}// end of if else
}// end of makeBet
/**
* Make sure bounds are kept
* @param tester number to be tested
* @param high highest limit
* @param low lowest limit
* @return go or no go
*/
public boolean valid(int tester, int high, int low) {
if (low <= tester && tester <= high) {
return true; // valid
}// end of if
// not valid
System.out.println("Please enter a valid response");
return false;
}// end of valid
/**
* Generate a hand
* @param player comp or human
*/
public void generateHand(int player) {
// is card taken
boolean cardTaken;
// card
int crd;
// current value
int curVal;
// current suit
int curSuit;
cardTaken = true;
// issue card
for (crd = 0; crd < 4; crd++) {
do {
// create a random value
pc[player][crd][0] = ((int) (Math.random() * 52) % 13);
// create a random suit
pc[player][crd][1] = ((int) (Math.random() * 52) % 4);
curVal = pc[player][crd][0];
curSuit = pc[player][crd][1];
if (cards[curVal][curSuit] == 'T') {
cardTaken = true;
} else {
cardTaken = false;
}
} while (cardTaken || pc[player][crd][0] > 12);
cards[curVal][curSuit] = ' ';
}// end of for loop
}// end of generate hand
/**
* Determine type of hand
* @param player comp or human
*/
public void determineHand(int player) {
boolean group1 = false;
// sort cards in numeric order
sortCards(player);
// easy to determine hand
int cardVal1 = pc[player][0][0];
int cardVal2 = pc[player][1][0];
int cardVal3 = pc[player][2][0];
int cardVal4 = pc[player][3][0];
int cardVal5 = pc[player][4][0];
int suitVal1 = pc[player][0][1];
int suitVal2 = pc[player][1][1];
int suitVal3 = pc[player][2][1];
int suitVal4 = pc[player][3][1];
int suitVal5 = pc[player][4][1];
hand[player] = 0;
// determine a pair
if (cardVal1 == cardVal2 || cardVal2 == cardVal3 || cardVal3 == cardVal4
|| cardVal4 == cardVal5) {
group1 = true;
hand[player] = 1;
// determine two pair
}
if (group1) {
if (cardVal1 == cardVal2 && cardVal3 == cardVal4
|| cardVal1 == cardVal2 && cardVal4 == cardVal5
|| cardVal2 == cardVal3 && cardVal4 == cardVal5) {
hand[player] = 2;
}
//Three of a kind
if (cardVal1 == cardVal2 && cardVal1 == cardVal3
|| cardVal2 == cardVal3 && cardVal2 == cardVal4
|| cardVal3 == cardVal4 && cardVal3 == cardVal5) {
hand[player] = 3;
}
// determine a fullhouse
if (cardVal1 == cardVal2 && cardVal3 == cardVal4
&& cardVal3 == cardVal5 || cardVal1 == cardVal2
&& cardVal1 == cardVal3 && cardVal4 == cardVal5) {
hand[player] = 6;
/**
* Determine type of hand
* @param player comp or human
*/
public void determineHand(int player) {
boolean group1 = false;
// sort cards in numeric order
sortCards(player);
// easy to determine hand
int cardVal1 = pc[player][0][0];
int cardVal2 = pc[player][1][0];
int cardVal3 = pc[player][2][0];
int cardVal4 = pc[player][3][0];
int cardVal5 = pc[player][4][0];
int suitVal1 = pc[player][0][1];
int suitVal2 = pc[player][1][1];
int suitVal3 = pc[player][2][1];
int suitVal4 = pc[player][3][1];
int suitVal5 = pc[player][4][1];
hand[player] = 0;
// determine a pair
if (cardVal1 == cardVal2 || cardVal2 == cardVal3 || cardVal3 == cardVal4
|| cardVal4 == cardVal5) {
group1 = true;
hand[player] = 1;
// determine two pair
}
if (group1) {
if (cardVal1 == cardVal2 && cardVal3 == cardVal4
|| cardVal1 == cardVal2 && cardVal4 == cardVal5
|| cardVal2 == cardVal3 && cardVal4 == cardVal5) {
hand[player] = 2;
}
//Three of a kind
if (cardVal1 == cardVal2 && cardVal1 == cardVal3
|| cardVal2 == cardVal3 && cardVal2 == cardVal4
|| cardVal3 == cardVal4 && cardVal3 == cardVal5) {
hand[player] = 3;
}
// determine a fullhouse
if (cardVal1 == cardVal2 && cardVal3 == cardVal4
&& cardVal3 == cardVal5 || cardVal1 == cardVal2
&& cardVal1 == cardVal3 && cardVal4 == cardVal5) {
hand[player] = 6;
}
// determine four of a kind
if (cardVal1 == cardVal2 && cardVal1 == cardVal3
&& cardVal1 == cardVal4 || cardVal2 == cardVal3
&& cardVal2 == cardVal4 && cardVal2 == cardVal5) {
hand[player] = 7;
}
}
group1 = false;
}
// check suits
if (!group1) {
// determine a straight
if (cardVal1 == (cardVal2 - 1)
&& cardVal2 == (cardVal3 - 1) && cardVal3
== (cardVal4 - 1) && cardVal4
== (cardVal5 - 1)) {
hand[player] = 4;
}
// determine straight flush
if (suitVal1 == suitVal2 && suitVal1 == suitVal3
&& suitVal1 == suitVal4
&& suitVal1 == suitVal5) {
hand[player] = 8;
}
// determine a royal flush
if (cardVal5 == 14)//Royal Flush
{
hand[player] = 9;
}// end of royalflush if
// determine flush
else if (suitVal1 == suitVal2
&& suitVal1 == suitVal3 && suitVal1
== suitVal4 && suitVal1 == suitVal5) {
hand[player] = 5;
} else {// determine just high Card
hand[player] = 0;
}// end of else
}
// display what player has
switch (player) {
case 0:
System.out.println("PLAYER has ");
break;
case 1:
System.out.println("CPU has ");
break;
}
switch (hand[player]) {
case 0:
System.out.println("A HIGH CARD");
break;
case 1:
System.out.println("A PAIR");
break;
case 2:
System.out.println("TWO PAIR");
break;
case 3:
System.out.println("THREE OF A KIND");
break;
case 4:
System.out.println("A STRAIGHT");
break;
case 5:
System.out.println("A FLUSH");
break;
case 6:
System.out.println("A FULL HOUSE");
break;
case 7:
System.out.println("FOUR OF A KIND");
break;
case 8:
System.out.println("STRAIGHT FLUSH");
break;
case 9:
System.out.println("ROYAL FLUSH");
break;
}// end of switch hand
}// end of determine hand
/**
* Determine the string of card value
* @param val index of array
* @return The string value (ie. Ace, 2, 3, ...)
*/
public String cardValString(int val) {
String tmpValStr = "";
if (val == 0) {
tmpValStr = "2";
}
if (val == 1) {
tmpValStr = "3";
}
if (val == 2) {
tmpValStr = "4";
}
if (val == 3) {
tmpValStr = "5";
}
if (val == 4) {
tmpValStr = "6";
}
if (val == 5) {
tmpValStr = "7";
}
if (val == 6) {
tmpValStr = "8";
}
if (val == 7) {
tmpValStr = "9";
}
if (val == 8) {
tmpValStr = "10";
}
if (val == 9) {
tmpValStr = "JACK";
}
if (val == 10) {
tmpValStr = "QUEEN";
}
if (val == 11) {
tmpValStr = "KING";
}
if (val == 12) {
tmpValStr = "ACE";
}
return tmpValStr + " of ";
}// end of cardValStr
/**
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

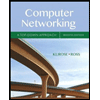
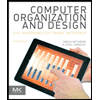
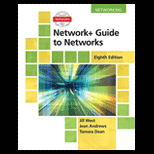
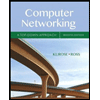
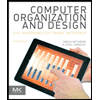
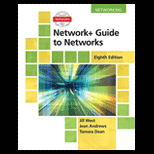
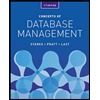
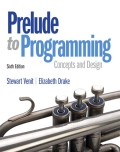
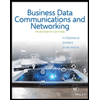