Use C code to consider the following contents of a text file called attendance.txt which records the student daily attendance for Class A and B. Day ClassA ClassB 1 50 38 2 48 40 3 44 36 Write C statements to perform the following: -Declare two file pointers named fp1 and fp2. -Open the attendance.txt text file for reading using fp1 file pointer. If the file is not opened successfully, display an error message “File opening errors” and exit the program. -Open the attnAverage.txt text file for writing using fp2 file pointer.
Use C code to consider the following contents of a text file called attendance.txt which records the student daily attendance for Class A and B. Day ClassA ClassB 1 50 38 2 48 40 3 44 36 Write C statements to perform the following: -Declare two file pointers named fp1 and fp2. -Open the attendance.txt text file for reading using fp1 file pointer. If the file is not opened successfully, display an error message “File opening errors” and exit the program. -Open the attnAverage.txt text file for writing using fp2 file pointer.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Use C code to consider the following contents of a text file called attendance.txt which records the student daily attendance for Class A and B.
Day ClassA ClassB 1 50 38 2 48 40 3 44 36 |
Write C statements to perform the following:
-Declare two file pointers named fp1 and fp2.
-Open the attendance.txt text file for reading using fp1 file pointer. If the file is not opened
successfully, display an error message “File opening errors” and exit the program.
-Open the attnAverage.txt text file for writing using fp2 file pointer.
Expert Solution

Step 1
I have implemented the given requirements as per the specification. The code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <sstream>
int main(void)
{
FILE *fp1, *fp2;
fp1 = fopen("attendence.txt", "r");
fp2 = fopen("attnAverage.txt", "w+");
if (fp1 == NULL || fp2 == NULL)
{
perror("File opening error!");
exit(1);
}
char line[128];
char val[3];
int attendence[50] = {0};
int k = 0, len = 0, count = -1, digit = 0;
while (fgets(line, sizeof(line), fp1) != NULL)
{
count++;
if (count == 0)
continue;
int l = sizeof(line) / sizeof(line[0]);
for (int i = 0; i < l; i++)
{
char ch = line[i];
if (ch == ' ')
continue;
else if (ch == '\0')
break;
else
{
if (i == 0)
continue;
val[k++] = ch;
if (line[i + 1] == ' ' || line[i + 1] == '\0')
{
if (val[0] == '\0' || val[1] == '\0')
continue;
digit = (val[0] - '0') * 10 + (val[1] - '0');
attendence[len++] = digit;
k = 0;
val[0] = '\0';
val[1] = '\0';
val[2] = '\0';
}
}
}
}
float avgA = 0.0, avgB = 0.0;
int size = sizeof(attendence) / sizeof(attendence[0]);
for (int i = 0; i < size; i++)
{
if (i % 2 == 0)
avgA += attendence[i];
else
avgB += attendence[i];
}
avgA /= count;
avgB /= count;
std::ostringstream osa;
std::ostringstream osb;
fprintf(fp2, "Average of class A is ");
osa << avgA;
fprintf(fp2, osa.str().c_str());
fprintf(fp2, "\nAverage of class B is ");
osb << avgB;
fprintf(fp2, osb.str().c_str());
printf("\nAverage of class A is %f.", avgA);
printf("\nAverage of class B is %f.", avgB);
fclose(fp1);
}
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
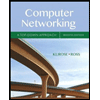
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
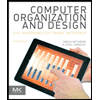
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
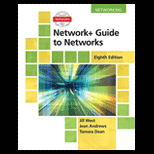
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
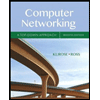
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
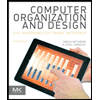
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
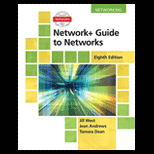
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
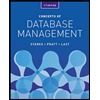
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
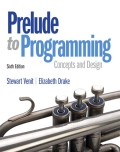
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
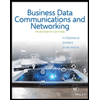
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY