Upgrade the BankAccount class to store accounts in a vector called accountsVector. Create a static variable called count that stores the number of BankAccount objects created. Read data as before from the same file BankData.dat and create objects accordingly and store them in the vector. You may need to add or modify methods in your BankAccount class, as well as helper methods in your main class. Extend the BankAccount class to include a static int variable called count that stores the number of objects created. Note you will need to use the scope resolution operator :: to access this variable. BankAccount -string accountName // First and Last name of Account holder -int accountId // secret social security number -int accountNumber // integer -double accountBalance // current balance amount + static int count // number of objects created ** + BankAccount() //default constructor that sets name to "", account number to 0 and balance to 0 +BankAccount(string accountName,int id, int accountNumber, double accountBalance) // regular constructor +getAccountBalance(): double // returns the balance +getAccountName: string // returns name +getAccountNumber: int +setAccountBalance(double amount) : void +equals(BankAccount other) : bool // returns true if this equals other. False otherwise ** +getCount(): int // returns count ** -getId():void +withdraw(double amount) : bool //deducts from balance and returns true if resulting balance is less than minimum balance +deposit(double amount): void //adds amount to balance. If amount is greater than rewards amount, calls // addReward method -addReward(double amount) void // adds rewards rate * amount to balance +toString(): String // return the account information as a string with three lines. "Account Name: " name "Account Number:" number "Account Balance:" balance Create the specification file BankAccountHeader.h which meets the above specifications Create the implementation file BankAccount.cpp which implements the BankAccount class as given in the UML diagram above. The class will have member variables( attributes/data) and instance methods(behaviours/functions that initialize, access and process data) make a driver class to do the following: Read data from the given file txt and create and vector of BankAccount Objects The order in the file is first name, last name, id, account number, balance. Note that account name consists of both first and last name Print the vector (without secret id) as in Homework3. Find the account with largest balance and print it Find the account with the smallest balance and print it. Print the number of objects using static count variable as well as vector size method Determine if there are duplicate accounts in the vector If there are then erase the account from the vector. Note the difference in the method return value and the procedure to the equals method in Homework 3. See how array deletion is different from vector deletion. If there are duplications then reprint the vector as before Again, print the number of objects using static count variable as well as vector size method See if there is a difference. Insert three new accounts at position 3, 5 and 7 of the vector. Remember your vector will keep increasing in size as you add! The three accounts are: Name : "Amy Machado" Id: 387623 Account Number : 1244 Balance: 1023.67 Name: "Tak Phen" Id: 981243 Account Number: 1262 Balance: 6423.03 Name: "Celia Beatle" Id: 465281 Account Number: 1276 Balance: 3.56 Please note that you may use a simple linear search based on bank balance as search key in this assignment For now, you may declare the function prototype at the beginning of the driver file, and the function definition at the bottom of the same file. We will separate the function definitions into another function header file when the number gets larger. BankData.txt: Matilda Patel 453456 1232 -4.00 Fernando Diaz 323468 1234 250.0 Vai vu 432657 1240 987.56 Howard Chen 234129 1236 194.56 Vai vu 432657 1240 -888987.56 Sugata Misra 987654 1238 10004.8 Fernando Diaz 323468 1234 8474.0 Lily Zhaou 786534 1242 001.98 THE OUTPUT SHOULD LOOK LIKE SCREENSHOT
Upgrade the BankAccount class to store accounts in a vector called accountsVector. Create a static variable called count that stores the number of BankAccount objects created. Read data as before from the same file BankData.dat and create objects accordingly and store them in the vector. You may need to add or modify methods in your BankAccount class, as well as helper methods in your main class.
- Extend the BankAccount class to include a static int variable called count that stores the number of objects created.
- Note you will need to use the scope resolution operator :: to access this variable.
BankAccount
-string accountName // First and Last name of Account holder
-int accountId // secret social security number
-int accountNumber // integer
-double accountBalance // current balance amount
+ static int count // number of objects created **
+ BankAccount() //default constructor that sets name to "", account number to 0
and balance to 0
+BankAccount(string accountName,int id, int accountNumber, double accountBalance) //
regular constructor
+getAccountBalance(): double // returns the balance
+getAccountName: string // returns name
+getAccountNumber: int
+setAccountBalance(double amount) : void
+equals(BankAccount other) : bool // returns true if this equals other. False otherwise **
+getCount(): int // returns count **
-getId():void
+withdraw(double amount) : bool //deducts from balance and returns true if resulting balance
is less than minimum balance
+deposit(double amount): void //adds amount to balance. If amount is greater than rewards
amount, calls
// addReward method
-addReward(double amount) void // adds rewards rate * amount to balance
+toString(): String // return the account information as a string with three lines. "Account
Name: " name
"Account Number:" number
"Account Balance:" balance
- Create the specification file BankAccountHeader.h which meets the above specifications
- Create the implementation file BankAccount.cpp which implements the BankAccount class as given in the UML diagram above. The class will have member variables( attributes/data) and instance methods(behaviours/functions that initialize, access and process data)
- make a driver class to do the following:
- Read data from the given file txt and create and vector of BankAccount Objects
The order in the file is first name, last name, id, account number, balance. Note that account name consists of both first and last name
- Print the vector (without secret id) as in Homework3.
- Find the account with largest balance and print it
- Find the account with the smallest balance and print it.
- Print the number of objects using static count variable as well as vector size method
- Determine if there are duplicate accounts in the vector If there are then erase the account from the vector. Note the difference in the method return value and the procedure to the equals method in Homework 3. See how array deletion is different from vector deletion.
- If there are duplications then reprint the vector as before
- Again, print the number of objects using static count variable as well as vector size method
See if there is a difference.
- Insert three new accounts at position 3, 5 and 7 of the vector. Remember your vector will keep increasing in size as you add! The three accounts are:
Name : "Amy Machado" Id: 387623 Account Number : 1244 Balance: 1023.67
Name: "Tak Phen" Id: 981243 Account Number: 1262 Balance: 6423.03
Name: "Celia Beatle" Id: 465281 Account Number: 1276 Balance: 3.56
Please note that you may use a simple linear search based on bank balance as search key in this assignment
For now, you may declare the function prototype at the beginning of the driver file, and the function definition at the bottom of the same file. We will separate the function definitions into another function header file when the number gets larger.
BankData.txt:
Matilda Patel 453456 1232 -4.00
Fernando Diaz 323468 1234 250.0
Vai vu 432657 1240 987.56
Howard Chen 234129 1236 194.56
Vai vu 432657 1240 -888987.56
Sugata Misra 987654 1238 10004.8
Fernando Diaz 323468 1234 8474.0
Lily Zhaou 786534 1242 001.98
THE OUTPUT SHOULD LOOK LIKE SCREENSHOT

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

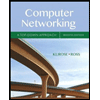
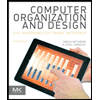
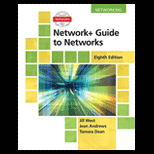
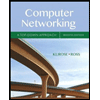
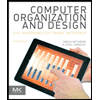
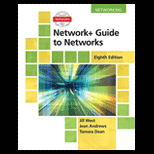
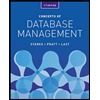
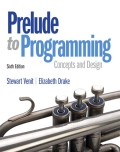
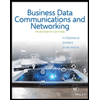