Update users passwords from database Create a form called change_password.html with the following: • A text field where the user can enter a username. • A text field where the user can the new password. • A button "Change". • The change should be done in a new page called change_password.php. In change_password.php: a connection to the database should be made. • The account with the username should have their password change to the • newly provided password.
Update users passwords from database Create a form called change_password.html with the following: • A text field where the user can enter a username. • A text field where the user can the new password. • A button "Change". • The change should be done in a new page called change_password.php. In change_password.php: a connection to the database should be made. • The account with the username should have their password change to the • newly provided password.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please solve the error

Transcribed Image Text:PHP & MYSQL
Update users passwords from database
Create a form called change_password.html with the following:
A text field where the user can enter a username.
• A text field where the user can the new password.
• A button “Change".
• The change should be done in a new page called change_password.php.
In change_password.php:
a connection to the database should be made.
• The account with the username should have their password change to the
newly provided password.
<!DOCTYPE html>
2▼ <html>
3▼ <head>
<title>Password Change</title>
</head>
6 v <body>
1.
4.
7
8.
<h3_align="center">CHANGE PASSWORD</h3>
9 v <form method="post" action="change_password.php" align="center">
10
11
Username : <br>
12
13
<input type="text" name="user_name"><span id="user_name" class="required"></span>
14
15
<br>
16
17
Current Password: <br>
18
19
<input type="password" name="currentPassword"><span id="currentPassword" class="required"></span>
20
<br>
21
22
New Password: <br>
23
24
<input type="password" name="newPassword"><span id="newPassword" class="required"></span>
25
<br>
26
27
Confirm Password: <br>
28
<input type="password" name="confirmPassword"><span id="confirmPassword" class="required"></span>
<br><br>
29
30
31
32
<input type="submit" value="Change">
33
34
</form>
35\
36
<br>
37
38
</body>
</html>
39
40
![<?php
session_start (); //Starting session
2
//Connection to database
$conn = mysqli_connect("127.0.0.1", "root", "", "student_db");
4.
5
6.
//Check if connected or not if not show error message
8 v if(!$conn) {
echo "Debugging errno:
echo "<br>";
echo "Debugging error:
12 }
7
9
. mysqli_connect_errno();
10
. mysqli_connect_error();
11
13
//Check if post data is not empty
15 v if(count($_POST)>0) {
//Fetching old password from student table
$result = mysqli_query($conn,"SELECT * FROM student WHERE name = '".$_POST['user_name']."'");
$row=mysqli_fetch_assoc($result);
14
16
17
18
19
//check if old password match with new password and new password and confirm password matches
21 v if($_POST['currentPassword'] == $row['password'] && $_POST["newPassword"] == $_POST["confirmPassword"])
{
//Updating password with new password in db
mysqli_query($conn,"UPDATE student SET password= '".$_POST['newPassword']."' WHERE name =
'".$_POST['user_name']."'");
$message = "Password changed successfully";
25 }
26 v else { //Password did't match
$message = "Password is not correct";
}
20
22
23
24
27
28
29
30
}
31
32
echo $message; //printing final result
mysqli_close ($conn); //close connection from database
33
34
35\
36
?>
user_account
HI Columns
HB New
email (UNI, varchar)
Hl password (varchar)
user_name (UNI, varchar)
u_id (PRI, int)
T+
u id
user_name a 1
email
password
Edit i Copy
Delete
7
Notice: Undefined index: user_name in /or
change password/change_password.php on line 17
Warning: mysqli_fetch_assoc() expects parameter 1 to be mysqli_result, bool given in /o;
change password/change_password.php on line 18
Notice: Trying to access array offset on value of type null in /e
Password is not correct
'change password/change_password.php on line 21](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff5019b69-b2c0-4c6c-86f3-f3c55b941c7d%2F5fb51686-35f6-4f07-bf65-fb8361480c4b%2Fx971rce_processed.jpeg&w=3840&q=75)
Transcribed Image Text:<?php
session_start (); //Starting session
2
//Connection to database
$conn = mysqli_connect("127.0.0.1", "root", "", "student_db");
4.
5
6.
//Check if connected or not if not show error message
8 v if(!$conn) {
echo "Debugging errno:
echo "<br>";
echo "Debugging error:
12 }
7
9
. mysqli_connect_errno();
10
. mysqli_connect_error();
11
13
//Check if post data is not empty
15 v if(count($_POST)>0) {
//Fetching old password from student table
$result = mysqli_query($conn,"SELECT * FROM student WHERE name = '".$_POST['user_name']."'");
$row=mysqli_fetch_assoc($result);
14
16
17
18
19
//check if old password match with new password and new password and confirm password matches
21 v if($_POST['currentPassword'] == $row['password'] && $_POST["newPassword"] == $_POST["confirmPassword"])
{
//Updating password with new password in db
mysqli_query($conn,"UPDATE student SET password= '".$_POST['newPassword']."' WHERE name =
'".$_POST['user_name']."'");
$message = "Password changed successfully";
25 }
26 v else { //Password did't match
$message = "Password is not correct";
}
20
22
23
24
27
28
29
30
}
31
32
echo $message; //printing final result
mysqli_close ($conn); //close connection from database
33
34
35\
36
?>
user_account
HI Columns
HB New
email (UNI, varchar)
Hl password (varchar)
user_name (UNI, varchar)
u_id (PRI, int)
T+
u id
user_name a 1
email
password
Edit i Copy
Delete
7
Notice: Undefined index: user_name in /or
change password/change_password.php on line 17
Warning: mysqli_fetch_assoc() expects parameter 1 to be mysqli_result, bool given in /o;
change password/change_password.php on line 18
Notice: Trying to access array offset on value of type null in /e
Password is not correct
'change password/change_password.php on line 21
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
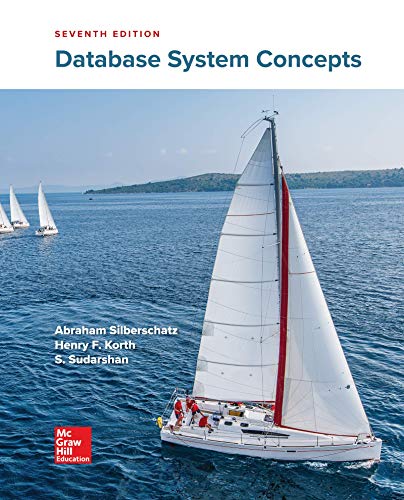
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
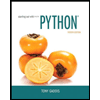
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
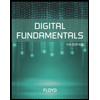
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
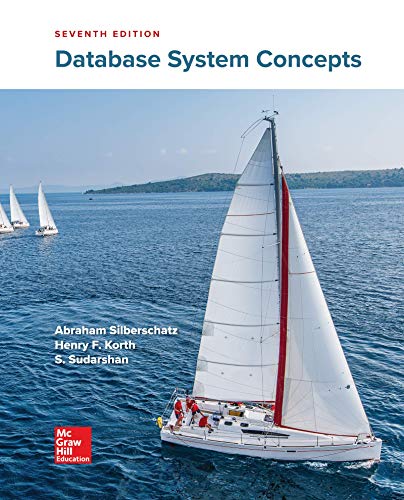
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
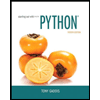
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
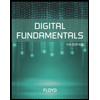
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
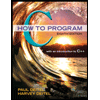
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
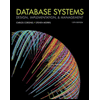
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
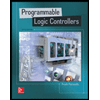
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education