ull code us beloweed WalkBehindMower, GasPoweredMower, PushReelMower, and MowerWarehouse class. I need the output to look exactly like the example in the photo. I pasted the "dataset" for the program. I pasted the others codes I've worked on so far to go off of. Please modify them as well. Dataset: public class Engine { private String manufacturer; private double horsePower; private int cylinders; public Engine() { manufacturer = ""; horsePower = 0; cylinders = 0; } public Engine(String manufacturer, double horsePower, int cylinders) { this.manufacturer = manufacturer; this.horsePower = horsePower; this.cylinders = cylinders; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public double getHorsePower() { return horsePower; } public void setHorsePower(double horsePower
N full code us beloweed WalkBehindMower, GasPoweredMower, PushReelMower, and MowerWarehouse class. I need the output to look exactly like the example in the photo. I pasted the "dataset" for the program. I pasted the others codes I've worked on so far to go off of. Please modify them as well.
Dataset:
public class Engine { private String manufacturer; private double horsePower; private int cylinders; public Engine() { manufacturer = ""; horsePower = 0; cylinders = 0; } public Engine(String manufacturer, double horsePower, int cylinders) { this.manufacturer = manufacturer; this.horsePower = horsePower; this.cylinders = cylinders; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public double getHorsePower() { return horsePower; } public void setHorsePower(double horsePower) { this.horsePower = horsePower; } public int getCylinders() { return cylinders; } public void setCylinders(int cylinders) { this.cylinders = cylinders; } @Override public String toString() { return "Engine: " + "\nmanufacturer=" + manufacturer + "\nhorsePower=" + horsePower + "\ncylinders=" + cylinders; } }
============================================================================================
public abstract class Mower { private String manufacturer; private int year; private String serialNumber; public Mower() { manufacturer = ""; year = 0; serialNumber = ""; } public Mower(String manufacturer, int year, String serialNumber) { this.manufacturer = manufacturer; this.year = year; this.serialNumber = serialNumber; } public String getManufacturer() { return manufacturer; } public void setManufacturer(String manufacturer) { this.manufacturer = manufacturer; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getSerialNumber() { return serialNumber; } public void setSerialNumber(String serialNumber) { this.serialNumber = serialNumber; } @Override public String toString() { return "Mower: " + "\nmanufacturer=" + manufacturer + "\nyear=" + year + "\nserialNumber='" + serialNumber; } }
============================================================================================
public class LawnTractor extends Mower { private Engine enginer; private String model; private double deckWidth; public LawnTractor() { } public Engine getEnginer() { return enginer; } public void setEnginer(Engine enginer) { this.enginer = enginer; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public double getDeckWidth() { return deckWidth; } public void setDeckWidth(double deckWidth) { this.deckWidth = deckWidth; } @Override public String toString() { return "LawnTractor:" + "\nenginer=" + enginer + "\nmodel=" + model + "\ndeckWidth=" + deckWidth ; } }
============================================================================================
public class CommericalMower extends LawnTractor { private double operatingHours; private boolean zeroTurnRadius; public CommericalMower() { operatingHours = 0; zeroTurnRadius = false; } public double getOperatingHours() { return operatingHours; } public void setOperatingHours(double operatingHours) { this.operatingHours = operatingHours; } public boolean isZeroTurnRadius() { return zeroTurnRadius; } public void setZeroTurnRadius(boolean zeroTurnRadius) { this.zeroTurnRadius = zeroTurnRadius; } @Override public String toString() { return "CommericalMower{" + "\nOperating Hours=" + operatingHours + "\nZero Turn Radius=" + zeroTurnRadius; } }.



Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

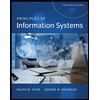
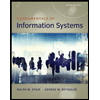
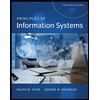
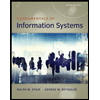