Two people play the game of Count 21 by taking turns entering a 1, 2, or 3, which is added to a running total. The player who adds the value that makes the total reach or exceed 21 loses the game. Create a game of Count 21 in which a player competes against the computer, and program a strategy that always allows the computer to win. On any turn, if the player enters a value other than 1, 2, or 3, force the player to reenter the value. Save the game as Count21.java.
I have an assignment that needs to be turn in before monday, here's the question:
Two people play the game of Count 21 by taking turns entering a 1, 2, or 3, which is added to a running total. The player who adds the value that makes the total reach or exceed 21 loses the game. Create a game of Count 21 in which a player competes against the computer, and program a strategy that always allows the computer to win. On any turn, if the player enters a value other than 1, 2, or 3, force the player to reenter the value. Save the game as Count21.java.
Here's the code that I'm working on:
import java.util.Scanner;
public class Count21 {
public static void main(String[] args) {
// Scanner object to get user input
Scanner scanner = new Scanner(System.in);
// Stores the running total.
//Displays a welcome for the user
public void promptEnterKey(){
System.out.println("Welcome to Count21! \n");
System.out.println("Press \"ENTER\" to continue...");
Scanner scanner = new Scanner(System.in);
scanner.nextLine();
}
// Game ends when running total exceeds 20.
int runningTotal = 0;
// Stores whose turn is it.
// If true, the next turn will be played by the user.
boolean isUserTurn = true;
while (true) {
// Stores the current number for the turn.
int turnNum = -1;
// If the current turn is for the user, get the turn from the user.
// else calculate the winning move for computer.
if(isUserTurn) {
// Prompt the user for his turn, until the user enters a valid
// number (1, 2, or 3)
while(turnNum < 1 || turnNum > 3) {
System.out.print("Its your turn, enter a number of 1, 2, or 3): \n");
turnNum = scanner.nextInt();
if(turnNum < 1 || turnNum > 3) {
System.out.println("Sorry, you can only enter 1, 2, or 3. \n");
}
}
} else {
// The winning move can be calculated by the following ways:
// 1. The user should play the first turn
// 2. The computer should increase the running total
// to the next multiple of 4.
for(int i=1; 4*i <= 20; i++) {
if(runningTotal < 4*i) {
turnNum = 4*i - runningTotal;
break;
}
}
// Printing computer's turn.
System.out.println("Computer turn: " + turnNum);
}
// Adding the current turn to the running total
runningTotal += turnNum;
System.out.println("Running total: " + runningTotal);
// If running total exceeds 20, game ends.
if(runningTotal >= 21) {
break;
}
isUserTurn = !isUserTurn;
}
// Print the winner according to who player last.
if(isUserTurn) {
System.out.println("Sorry you lose.. \n");
} else {
System.out.println("You win! \n");
}
}
}
What I want to add is a welcome message while the user can press ENTER or any key button to start the game but I'm having problems of getting it to work any help would be apprecitated.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

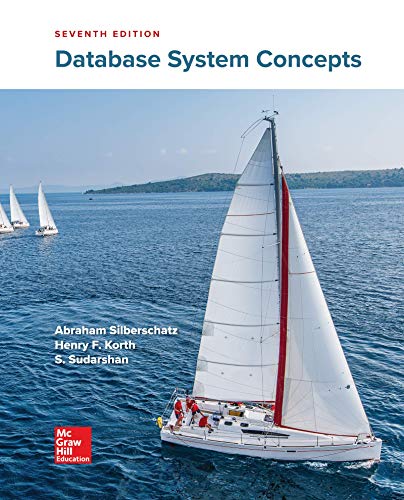
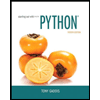
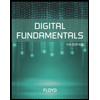
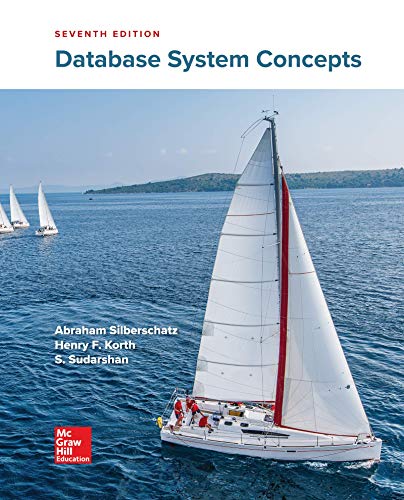
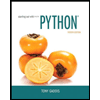
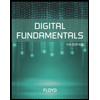
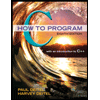
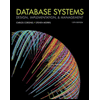
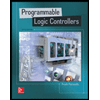