Topologically Sorting DAGS def naive topsort(G,S=None): if S is None: S=set(G) # Default: All nodes if len(S)==1: return list(S)# Base case, single node # Reduction: Remove a node # Recursion (assumption), n=1 v=S.pop () seq=naive topsort (G,S) min i=0 for i,u in enumerate(seq): if v in G[u]: min i=i+1 seq.insert (min i,v) # After all dependencies return seq Using adjacency sets or dicts to represent a DAG.
Topologically Sorting DAGS def naive topsort(G,S=None): if S is None: S=set(G) # Default: All nodes if len(S)==1: return list(S)# Base case, single node # Reduction: Remove a node # Recursion (assumption), n=1 v=S.pop () seq=naive topsort (G,S) min i=0 for i,u in enumerate(seq): if v in G[u]: min i=i+1 seq.insert (min i,v) # After all dependencies return seq Using adjacency sets or dicts to represent a DAG.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please show all work so i can better understand
![# Topologically Sorting DAGs
Below is a Python function `naive_topsort` demonstrating how to perform a topological sort on a Directed Acyclic Graph (DAG). This implementation assumes a set-based representation of the graph nodes.
```python
def naive_topsort(G, S=None):
if S is None: S = set(G) # Default: All nodes
if len(S) == 1: return list(S) # Base case, single node
v = S.pop() # Reduction: Remove a node
seq = naive_topsort(G, S) # Recursion (assumption), n=1
min_i = 0
for i, u in enumerate(seq):
if v in G[u]: min_i = i + 1 # After all dependencies
seq.insert(min_i, v)
return seq
```
- **Explanation**
- **Line 2-3**: If the set `S` is not provided, initialize it with all nodes in `G`.
- **Line 4-5**: Base case for recursion. If there's only one node, return it as a list.
- **Line 6**: Remove a node `v` from the set `S`.
- **Line 7**: Recursively call `naive_topsort` for the reduced graph.
- **Lines 9-13**: Determine the position `min_i` to insert `v` after all dependencies.
- **Line 14**: Insert `v` into the sequence at the appropriate position.
- **Line 15**: Return the updated sequence.
- **Notes**
- The graph `G` is represented using adjacency sets or dictionaries.
- This script poses the question, "What’s the expected runtime?," prompting a discussion on the algorithm’s efficiency.
The function provides a foundational approach for educational purposes, illustrating the recursive nature of topological sorting and dependency management in DAGs.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa71f6ed3-a5c4-487e-8e5f-80efc3c4678f%2F8d75f1de-7589-4745-8ba1-1282651ccefe%2Fk35v1a_processed.png&w=3840&q=75)
Transcribed Image Text:# Topologically Sorting DAGs
Below is a Python function `naive_topsort` demonstrating how to perform a topological sort on a Directed Acyclic Graph (DAG). This implementation assumes a set-based representation of the graph nodes.
```python
def naive_topsort(G, S=None):
if S is None: S = set(G) # Default: All nodes
if len(S) == 1: return list(S) # Base case, single node
v = S.pop() # Reduction: Remove a node
seq = naive_topsort(G, S) # Recursion (assumption), n=1
min_i = 0
for i, u in enumerate(seq):
if v in G[u]: min_i = i + 1 # After all dependencies
seq.insert(min_i, v)
return seq
```
- **Explanation**
- **Line 2-3**: If the set `S` is not provided, initialize it with all nodes in `G`.
- **Line 4-5**: Base case for recursion. If there's only one node, return it as a list.
- **Line 6**: Remove a node `v` from the set `S`.
- **Line 7**: Recursively call `naive_topsort` for the reduced graph.
- **Lines 9-13**: Determine the position `min_i` to insert `v` after all dependencies.
- **Line 14**: Insert `v` into the sequence at the appropriate position.
- **Line 15**: Return the updated sequence.
- **Notes**
- The graph `G` is represented using adjacency sets or dictionaries.
- This script poses the question, "What’s the expected runtime?," prompting a discussion on the algorithm’s efficiency.
The function provides a foundational approach for educational purposes, illustrating the recursive nature of topological sorting and dependency management in DAGs.
Expert Solution

Recurrence Analysis :
- The given algorithm recursively finds the solution of the topological sort of the graph.
- Let T(n) be the time complexity for finding the topological sort of the graph having n number of vertices :
- T(n) = T(n - c) + O(1) where c = number of the neightbour of nth vetrex
- Here, c= O(V) i.e the maximum number of neighbors can be at a maximum of a total number of vertices.
- This recurrence equation is used for finding the solution to the time complexity of the given equation.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
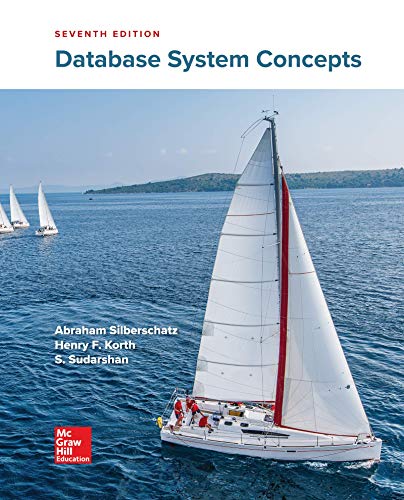
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
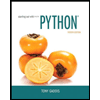
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
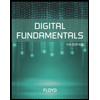
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
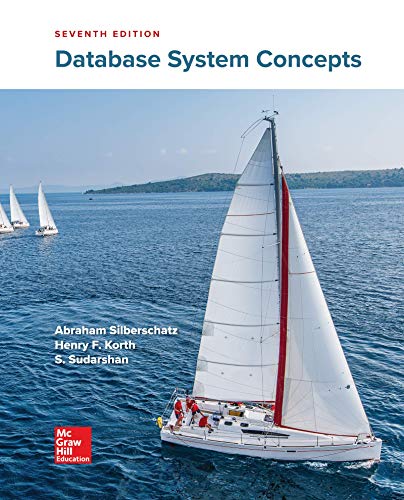
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
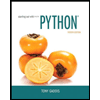
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
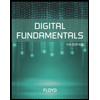
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
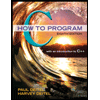
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
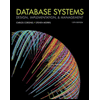
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
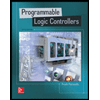
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education