This must be the Output Enter a number to choose a program: 1 - Convert Roman Numeral to Decimal Number 2 - Convert Decimal Number to Roman Numeral 3 - Exit/Quit Enter your selection: 1 Enter a Roman Numeral: V The decimal/integer form of Roman Numeral is: 5 Enter a number to choose a program: 1 - Convert Roman Numeral to Decimal Number 2 - Convert Decimal Number to Roman Numeral 3 - Exit/Quit Enter your selection: 2 Enter a Decimal Number: 5 The roman numeral form of decimal number is: V Enter a number to choose a program: 1 - Convert Roman Numeral to Decimal Number 2 - Convert Decimal Number to Roman Numeral 3 - Exit/Quit Enter your selection: 3 Thank you! Please enter a valid option.
This must be the Output
Enter a number to choose a
1 - Convert Roman Numeral to Decimal Number
2 - Convert Decimal Number to Roman Numeral
3 - Exit/Quit
Enter your selection: 1
Enter a Roman Numeral: V
The decimal/integer form of Roman Numeral is: 5
Enter a number to choose a program:
1 - Convert Roman Numeral to Decimal Number
2 - Convert Decimal Number to Roman Numeral
3 - Exit/Quit
Enter your selection: 2
Enter a Decimal Number: 5
The roman numeral form of decimal number is: V
Enter a number to choose a program:
1 - Convert Roman Numeral to Decimal Number
2 - Convert Decimal Number to Roman Numeral
3 - Exit/Quit
Enter your selection: 3
Thank you! Please enter a valid option.
What codes to use in java?

Introduction of the Program:
The Java program takes the user choice e.g. if the user press 1 then the program converts the roman numeral to a decimal number and if the user press 2 then the program converts a decimal number to the roman numeral and if user press 3 then we exit from the program.
Source code of the Program: Save the below code as Main.java
import java.util.*;
public class Main {
//Method returns decimal value corresponding to the Roman character
int value(char roman){
if (roman == 'I')
return 1;
if (roman == 'V')
return 5;
if (roman == 'X')
return 10;
if (roman == 'L')
return 50;
if (roman == 'C')
return 100;
if (roman == 'D')
return 500;
if (roman == 'M')
return 1000;
return -1;
}
//Method converts decimal value of the roman number
int romanToDecimal(String str){
int res = 0;
for (int i = 0; i < str.length(); i++) {
int str1 = value(str.charAt(i));
if (i + 1 < str.length()) {
int str2 = value(str.charAt(i + 1));
if (str1 >= str2){
res = res + str1;
}else{
res = res + str2 - str1;
i++;
}
}else {
res = res + str1;
}
}
return res;
}
static int sub_digit(char num1, char num2, int i, char[] c) {
c[i++] = num1;
c[i++] = num2;
return i;
}
// To add symbol 'ch' n times after index i in c[]
static int digit(char ch, int n, int i, char[] c) {
for (int j = 0; j < n; j++) {
c[i++] = ch;
}
return i;
}
//method to convert decimal to roman numeral
static void printRoman(int number) {
char c[] = new char[10001];
int i = 0;
if (number <= 0) {
System.out.printf("Invalid number");
return;
}
while (number != 0) {
if (number >= 1000) {
i = digit('M', number / 1000, i, c);
number = number % 1000;
} else if (number >= 500) {
if (number < 900) {
i = digit('D', number / 500, i, c);
number = number % 500;
} else {
i = sub_digit('C', 'M', i, c);
number = number % 100;
}
} else if (number >= 100) {
if (number < 400) {
i = digit('C', number / 100, i, c);
number = number % 100;
} else {
i = sub_digit('C', 'D', i, c);
number = number % 100;
}
} else if (number >= 50) {
if (number < 90) {
i = digit('L', number / 50, i, c);
number = number % 50;
} else {
i = sub_digit('X', 'C', i, c);
number = number % 10;
}
} else if (number >= 10) {
if (number < 40) {
i = digit('X', number / 10, i, c);
number = number % 10;
} else {
i = sub_digit('X', 'L', i, c);
number = number % 10;
}
}else if (number >= 5) {
if (number < 9) {
i = digit('V', number / 5, i, c);
number = number % 5;
} else {
i = sub_digit('I', 'X', i, c);
number = 0;
}
} else if (number >= 1) {
if (number < 4) {
i = digit('I', number, i, c);
number = 0;
} else {
i = sub_digit('I', 'V', i, c);
number = 0;
}
}
}
// Printing equivalent Roman Numeral
System.out.printf("The roman numeral form of decimal number is : ");
for (int j = 0; j < i; j++) {
System.out.printf("%c", c[j]);
}
}
// Driver Code
public static void main(String args[])
{
Main obj = new Main();
Scanner scanner= new Scanner(System.in);
int choice;
do{
System.out.println("Enter a number to choose a program:");
System.out.println("1- Convert Roman Numeral to Decimal Number");
System.out.println("2- Convert Decimal Number to Roman Numeral");
System.out.println("3- Exit/Quit");
System.out.print("\nEnter Your selection: ");
choice=scanner.nextInt();
if(choice==1){
System.out.print("Enter a Roman Numeral : ");
String str = scanner.next();
System.out.print("The decimal/integer form of Roman Numeral is : "
+ obj.romanToDecimal(str));
System.out.println("\n");
}else if(choice==2){
System.out.print("Enter a Decimal Number : ");
int number = scanner.nextInt();
printRoman(number);
System.out.println("\n");
}else
System.out.println("Thank you!Please Enter a valid option.");
}while(choice==1 || choice==2);
}
}
Step by step
Solved in 3 steps with 1 images

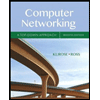
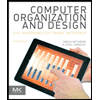
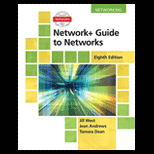
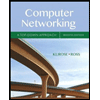
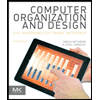
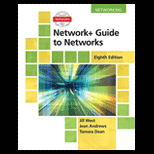
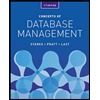
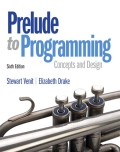
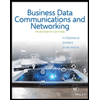