This is Java. Write the java code (not a full program) to delete an integer from a 1d array of integers. Assume the following: a. the array's physical size is 10. b. the array's logical (current size) is 7. c. we want to delete the value 600 in position 6 (index 5). 100 200 300 400 500 600 700 ? ? ? This is java please.
This is Java.
Write the java code (not a full
a. the array's physical size is 10.
b. the array's logical (current size) is 7.
c. we want to delete the value 600 in position 6 (index 5).
100 200 300 400 500 600 700 ? ? ?
This is java please.

Program Approach:
- Declaring main class
- Defining the main function
- Declaring integer type array with physical size 10
- Assigning values into an array with logical size 7
- Using for loop to printing array
- Display an array
- Declare integer type variable del
- Deleting array and replace it with the next element
- Display the message
- Using for loop to printing array
- Display the array
Program:
//declaring main class
public class Main
{
//defining main function
public static void main (String[] args)
{
//declaring integer type array with physical size 10
int arr[]=new int[10];
//assigning values into array with logical size 7
for(int i=0;i<7;i++)
{
arr[i]=(i+1)*100;
}
//using for loop to printing array
for(int i=0;i<7;i++)
{
//display an array
System.out.println(arr[i]);
}
//declare integer type variable del
//value to be deleted
int del=600;
//deleting array and replace it with next element
for(int i=0;i<7;i++)
{
if(arr[i]==del)
{
arr[i]=arr[i+1];
}
}
//display the message
System.out.println("After deletion");
//using for loop to printing array
for(int i=0;i<7;i++)
{
//display the array
System.out.println(arr[i]);
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

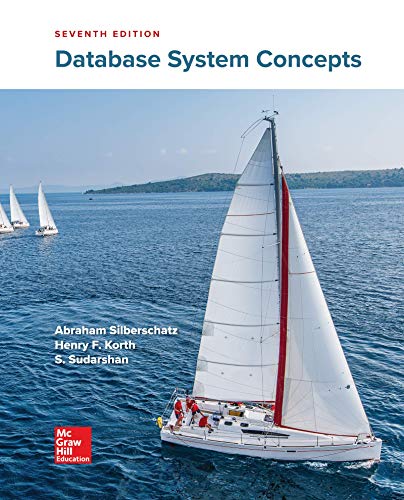
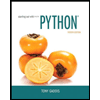
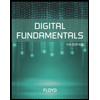
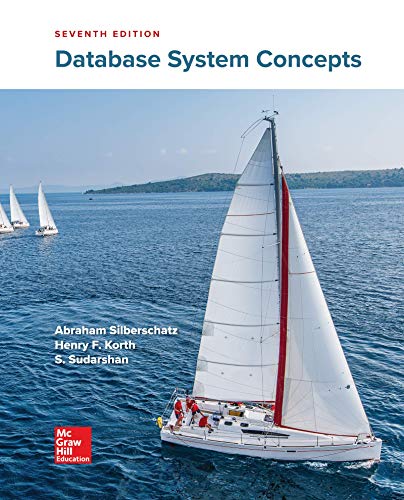
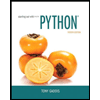
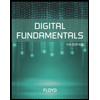
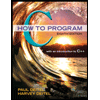
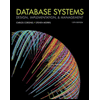
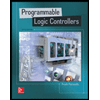