This is in C++. Write a function that, given a string, index, and character, returns the index of the first occurrence of the character starting the search at the index. If not found, return -1. If the inputs are "Hello-friends", 3, and 'e', the function returns 9 (indexing the 'e' in friends). Use a for loop along with a boolean variables to indicate the character was found, which should end the for loop immediately. main.cpp #include #include using namespace std; // Type your code here int main() { string inputString; int startIndex; char searchChar; cin >> inputString; cin >> startIndex; cin >> searchChar; cout << FindNextCharInString(inputString, startIndex, searchChar) << endl; return 0; }
This is in C++.
Write a function that, given a string, index, and character, returns the index of the first occurrence of the character starting the search at the index. If not found, return -1. If the inputs are "Hello-friends", 3, and 'e', the function returns 9 (indexing the 'e' in friends). Use a for loop along with a boolean variables to indicate the character was found, which should end the for loop immediately.
main.cpp
#include <iostream>
#include <string>
using namespace std;
// Type your code here
int main() {
string inputString;
int startIndex;
char searchChar;
cin >> inputString;
cin >> startIndex;
cin >> searchChar;
cout << FindNextCharInString(inputString, startIndex, searchChar) << endl;
return 0;
}
Thank you

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

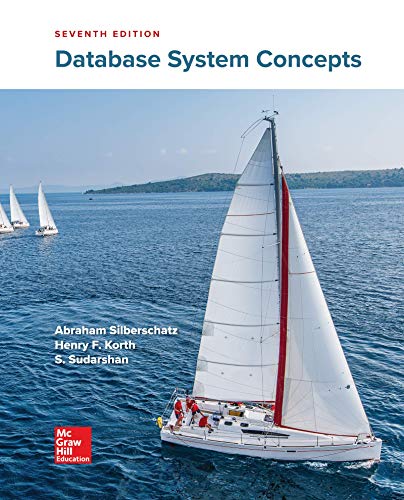
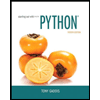
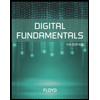
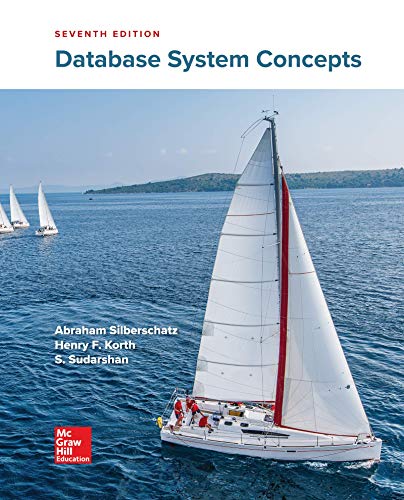
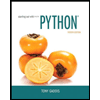
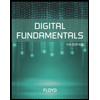
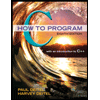
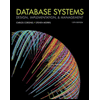
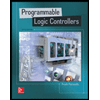