Write a function in MIPS Assembly Language that computes the next state of a linear feedback shift register (LFSR) given the current state of the LFSR. The LFSR should satisfy the taps given by the following polynomial: x^15 + x^14 +1 Your function should take the state as a 32-bit input parameter and return a 32-bit output value. Your main program for the function should call your LFSR function for the following input states and print the output state: 0x00000001 0xdeadbeef 0x200214c8 0x00000000
Write a function in MIPS Assembly Language that computes the next state of a linear feedback shift register (LFSR) given the current state of the LFSR. The LFSR should satisfy the taps given by the following polynomial:
x^15 + x^14 +1
Your function should take the state as a 32-bit input parameter and return a 32-bit output value. Your main
0x00000001
0xdeadbeef
0x200214c8
0x00000000

Given:
We have to write a function in MIPS Assembly Language that computes the next state of a linear feedback shift register (LFSR) given the current state of the LFSR. The LFSR should satisfy the taps given by the following polynomial:
x^15 + x^14 +1
Our function should take the state as a 32-bit input parameter and return a 32-bit output value. Our main program for the function should call our LFSR function for the following input states and print the output state:
0x00000001
0xdeadbeef
0x200214c8
0x00000000
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

The last line of the code, is there something missing on it? The last line says,
"move $v0, "
The output I got is 32768. Then after that line are next three lines of "(null)" with error message "Memory address out of bounds."
This is the updated code that you provided:
.data
newline:
.asciiz "\n"
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 1 # set system call for printing integer
move $a0, $t0
syscall
la $a0, newline
li $v0, 4 # set system call for printing string
syscall
move $a0, $t1
syscall
la $a0, newline
syscall
move $a0, $t2
syscall
la $a0, newline
syscall
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x8000 and lower mask with 0x0001
li $t0, 0x8000
li $t1, 0x0001
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t2, 0
li $t3, 0
lfsr_loop:
# Shift the current state to the right
srl $a0, $a0, 1
# Get the least significant bit of the current state
andi $t2, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t2, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Shift the tap mask to the right
srl $t0, $t0, 1
# If the tap mask is zero, reset it to the original mask
bne $t0, $zero, lfsr_increment
li $t0, 0x8000
lfsr_increment:
# Increment the loop counter
addi $t3, $t3, 1
# If the loop counter is less than 32, continue the loop
blt $t3, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
Can you help me fix this code? Thanks
This is the follow up question from the previous follow up question.
The code has to work in QtSpim. My professor wants the class to use the QtSpim. From the updated code, I am still getting error that says "Memory address out of bounds" and negative output. Can you help me fix this. Thanks.
.data
newline:
.asciiz "\n"
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 1 # set system call for printing integer
move $a0, $t0
syscall
la $a0, newline
li $v0, 4 # set system call for printing string
syscall
move $a0, $t1
syscall
la $a0, newline
syscall
move $a0, $t2
syscall
la $a0, newline
syscall
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x8000 and lower mask with 0x0001
lui $t0, 0x8000
ori $t1, $zero, 0x0001
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t2, 0
li $t3, 0
lfsr_loop:
# Shift the current state to the right
srl $a0, $a0, 1
# Get the least significant bit of the current state
andi $t2, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t2, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t3, $t3, 1
# If the loop counter is less than 32, continue the loop
blt $t3, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
I ran the updated code that you provide with li $v0, 34 for printing an unsigned integer. I got an error saying:
Unknown system call: 34
This the the updated code that you provided:
.data
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 34 # set system call for printing unsigned integer
move $a0, $t0
syscall
move $a0, $t1
syscall
move $a0, $t2
syscall
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x8000 and lower mask with 0x0001
lui $t0, 0x8000
ori $t1, $zero, 0x0001
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t2, 0
li $t3, 0
lfsr_loop:
# Shift the current state to the right
srl $a0, $a0, 1
# Get the least significant bit of the current state
andi $t2, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t2, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t3, $t3, 1
# If the loop counter is less than 32, continue the loop
blt $t3, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
I am running my code using the QtSpim.
How do I fix this?
I ran the updated code that you provided.
This is my output:
-2147483648100
This is the updated code that you provided:
.data
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 1
move $a0, $t0
syscall
li $v0, 1
move $a0, $t1
syscall
li $v0, 1
move $a0, $t2
syscall
li $v0, 1
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x8000 and lower mask with 0x0001
lui $t0, 0x8000
ori $t1, $zero, 0x0001
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t2, 0
li $t3, 0
lfsr_loop:
# Shift the current state to the right
srl $a0, $a0, 1
# Get the least significant bit of the current state
andi $t2, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t2, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t3, $t3, 1
# If the loop counter is less than 32, continue the loop
blt $t3, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
How do I fix this?
I am still getting the same wrong output using the updated code that you provided. I moved the andi instruction after the srl instruction.
This is the code that you provided with the fix:
.data
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 1
move $a0, $t0
syscall
li $v0, 1
move $a0, $t1
syscall
li $v0, 1
move $a0, $t2
syscall
li $v0, 1
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x7fff and lower mask with 0x8000
lui $t0, 0x7fff
ori $t0, $t0, 0x8000
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t1, 0
li $t2, 0
lfsr_loop:
# Shift the current state to the right
srl $a0, $a0, 1
# Get the least significant bit of the current state
andi $t1, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t1, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t2, $t2, 1
# If the loop counter is less than 32, continue the loop
blt $t2, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
But the output is still the same wrong output. How do I fix this?
I am still getting the wrong output with the updated code with the fix.
This is the updated coded that you provided:
.data
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
li $v0, 1
move $a0, $t0
syscall
li $v0, 1
move $a0, $t1
syscall
li $v0, 1
move $a0, $t2
syscall
li $v0, 1
move $a0, $t3
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x7fff and lower mask with 0x8000
lui $t0, 0x7fff
ori $t0, $t0, 0x8000
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t1, 0
li $t2, 0
lfsr_loop:
# Get the least significant bit of the current state
andi $t1, $a0, 1
# Shift the current state to the right
srl $a0, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t1, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t2, $t2, 1
# If the loop counter is less than 32, continue the loop
blt $t2, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
When I run this code, I am still getting the wrong output.
How do I fix this?
This is the updated code that I have:
.data
.globl main
.text
main:
# compute the next state of the LFSR for each input state
li $a0, 0x00000001
jal lfsr_next_state
move $t0, $v0
li $a0, 0xdeadbeef
jal lfsr_next_state
move $t1, $v0
li $a0, 0x200214c8
jal lfsr_next_state
move $t2, $v0
li $a0, 0x00000000
jal lfsr_next_state
move $t3, $v0
# print the output states
move $a0, $t0
li $v0, 1
syscall
move $a0, $t1
li $v0, 1
syscall
move $a0, $t2
li $v0, 1
syscall
move $a0, $t3
li $v0, 1
syscall
# exit the program
li $v0, 10
syscall
# Function to compute the next state of an LFSR
# Input parameter: $a0 = current state
# Output: $v0 = next state
lfsr_next_state:
# Initialize upper mask with 0x7fff and lower mask with 0x8000
lui $t0, 0x7fff
ori $t0, $t0, 0x8000
# Initialize result with 0
li $v0, 0
# Loop through all 32 bits of the input state
li $t1, 0
li $t2, 0
lfsr_loop:
# Get the least significant bit of the current state
andi $t1, $a0, 1
# Shift the current state to the right
srl $a0, $a0, 1
# If the least significant bit is 1, XOR with the tap mask
beq $t1, 1, lfsr_xor
j lfsr_continue
lfsr_xor:
xor $a0, $a0, $t0
lfsr_continue:
# Increment the loop counter
addi $t2, $t2, 1
# If the loop counter is less than 32, continue the loop
blt $t2, 32, lfsr_loop
# Set the result to the current state
move $v0, $a0
jr $ra
When I run this code, the output is this:
21474508800320
How do I fix this?
This is follow-up question to the answer from the previous follow-up question.
When I run the updated code, I'm only getting this output:
21474508800320
Is this correct?
This is follow-up question to the answer from the previous follow-up question.
I'm getting an error on this line:
andi $t0, $a0, 0x7fff8000 # mask off the lower 15 bits
The error says:
spim: (parser) immediate value (2147450880) out of range (0 .. 65535)
The LFSR needs to be written as function and the main function should call the LFSR function using the "jal" assemly instruction and "jr $ra" to go back to main function. Could you update the codes?
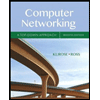
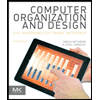
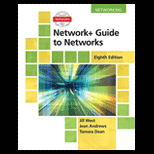
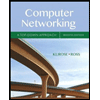
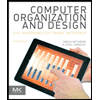
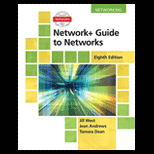
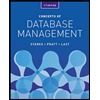
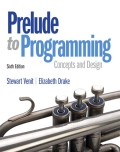
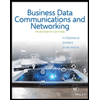