This is computer architecture! PLEASE HELP ME FIX THE CODE AS THERE IS ERROR! # This program corrects bad data using Hamming codes # It requests the user to enter a 12-bit Hamming code and determines if it is correct or not # If correct, it displays a message to that effect. If incorrect, it displays a message # saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. # This program is designed to handle single bit errors only.
This is computer architecture!
PLEASE HELP ME FIX THE CODE AS THERE IS ERROR!
# This
# It requests the user to enter a 12-bit Hamming code and determines if it is correct or not
# If correct, it displays a message to that effect. If incorrect, it displays a message
# saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex.
# This program is designed to handle single bit errors only.
.data
prompt: .asciiz "Enter a 12-bit Hamming code: "
correct_msg: .asciiz "The Hamming code is correct."
incorrect_msg: .asciiz "The Hamming code is incorrect. The correct code is: "
newline: .asciiz "\n"
.text
.globl main
main:
# Display prompt to enter Hamming code
li $v0, 4
la $a0, prompt
syscall
# Read Hamming code from user
li $v0, 5
syscall
move $t0, $v0
# Calculate parity bits
andi $t1, $t0, 0b1111 # Parity bit p1
andi $t2, $t0, 0b011001100110 # Parity bit p2
srl $t2, $t2, 1
xor $t1, $t1, $t2
andi $t2, $t0, 0b000011110000 # Parity bit p4
srl $t2, $t2, 4
xor $t1, $t1, $t2
andi $t2, $t0, 0b000000001111 # Parity bit p8
srl $t2, $t2, 8
xor $t1, $t1, $t2
# Check if Hamming code is correct
beq $t1, 0, print_correct_msg
# Hamming code is incorrect, correct it
xor $t0, $t0, 1 << ($t1 - 1)
print_incorrect_msg:
# Display incorrect message
li $v0, 4
la $a0, incorrect_msg
syscall
# Display correct Hamming code
li $v0, 34
move $a0, $t0
syscall
# Display newline
li $v0, 4
la $a0, newline
syscall
# Exit program
li $v0, 10
syscall
print_correct_msg:
# Display correct message
li $v0, 4
la $a0, correct_msg
syscall
# Exit program
li $v0, 10
syscall
Heres the errors:
line 55 column 20: andi $t1, $t0, 0b1111 # Parity bit p1
Invalid language element: 0b1111
line 57 column 20: andi $t2, $t0, 0b011001100110 # Parity bit p2
Invalid language element: 0b011001100110
line 63 column 20: andi $t2, $t0, 0b000011110000 # Parity bit p4
Invalid language element: 0b000011110000
line 69 column 20: andi $t2, $t0, 0b000000001111 # Parity bit p8
Invalid language element: 0b000000001111
line 85 column 21: xor $t0, $t0, 1 << ($t1 - 1)
Invalid language element: <<
line 55 column 20: andi $t1, $t0, 0b1111 # Parity bit p1
Invalid language element: 0b1111
line 57 column 20: andi $t2, $t0, 0b011001100110 # Parity bit p2
Invalid language element: 0b011001100110
line 63 column 20: andi $t2, $t0, 0b000011110000 # Parity bit p4
Invalid language element: 0b000011110000
line 69 column 20: andi $t2, $t0, 0b000000001111 # Parity bit p8
Invalid language element: 0b000000001111
line 85 column 21: xor $t0, $t0, 1 << ($t1 - 1)
Invalid language element: <<
Assemble: operation completed with errors.

Step by step
Solved in 3 steps

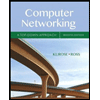
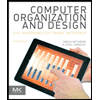
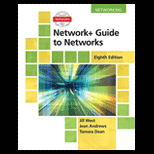
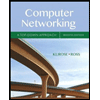
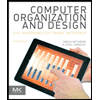
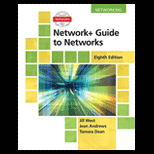
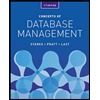
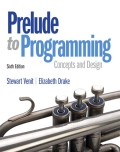
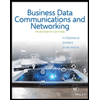