Given is Parser.java. Methods must accept tokens appropriately and generates OperationNode or returns partial result. I need the methods to be created for the following: -Post Increment/Decrement -Exponents -Factor -Term -Expression -Concatenation -Boolean Compare -Match -Array Membership -And -Or -Ternary -Assignment Parser.java import java.text.ParseException; import java.util.LinkedList; import java.util.List; import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList tokens; public Parser(LinkedList tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional currentToken = tokenHandler.getCurrentToken(); if (currentToken.isPresent() && (currentToken.get().getType() == Token.TokenType.NEWLINE || currentToken.get().getType() == Token.TokenType.SEMICOLON)) { tokenHandler.consumeMatchedToken(); foundSeparator = true; } else { break; } } return foundSeparator; } public ProgramNode Parse() throws ParseException { } return false; } private boolean parseAction(ProgramNode programNode) { Optional functionNameToken = tokenHandler.getCurrentToken(); if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) { ActionMap actionMap = new ActionMap(); programNode.addNode(actionMap); AcceptSeparators(); return true; } return false; } public VariableReferenceNode ParseLValueVariable(String variableName) { return new VariableReferenceNode(variableName, Optional.empty()); } public VariableReferenceNode ParseLValueArray(String variableName, int index) { return new VariableReferenceNode(variableName, Optional.of(index)); } public OperationNode ParseLValueDollar() { return new OperationNode("$"); } public ConstantNode ParseBottomLevelConstantsAndPatterns() { ConstantNodePatternNode node = new ConstantOrNodePatternNode(); node.setConstant("example"); node.setPattern("PATTERN_1"); return node; } public OperationNode ParseBottomLevelParenthesis() throws ParseException { if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) { OperationNode operationNode = ParseExpression(); if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) { return operationNode; } else { throw new ParseException("Expected ')'", tokenHandler.getCurrentToken().getStart()); } return null; } else { throw new ParseException("Expected '('", tokenHandler.getCurrentToken().getStart()); } } private OperationNode ParsePower() throws ParseException { OperationNode left = ParseBottomLevelParenthesis(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.CARROT); if (op.isEmpty()) { return left; } OperationNode right = ParseBottomLevelParenthesis(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseLogicalAnd() throws ParseException { OperationNode left = ParseComparison(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.AND); if (op.isEmpty()) { return left; } OperationNode right = ParseComparison(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseComparison() throws ParseException { OperationNode left = ParseAdditionOrSubtraction(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.EQUAL) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.NOT_EQUAL)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN_OR_EQUAL)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN_OR_EQUAL)); if (op.isEmpty()) { return left; } OperationNode right = ParseAdditionOrSubtraction(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseAdditionOrSubtraction() { return null; } private OperationNode ParseLogicalOr() throws ParseException { OperationNode left = ParseLogicalAnd();
Given is Parser.java. Methods must accept tokens appropriately and generates OperationNode or returns partial result. I need the methods to be created for the following: -Post Increment/Decrement -Exponents -Factor -Term -Expression -Concatenation -Boolean Compare -Match -Array Membership -And -Or -Ternary -Assignment Parser.java import java.text.ParseException; import java.util.LinkedList; import java.util.List; import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList tokens; public Parser(LinkedList tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional currentToken = tokenHandler.getCurrentToken(); if (currentToken.isPresent() && (currentToken.get().getType() == Token.TokenType.NEWLINE || currentToken.get().getType() == Token.TokenType.SEMICOLON)) { tokenHandler.consumeMatchedToken(); foundSeparator = true; } else { break; } } return foundSeparator; } public ProgramNode Parse() throws ParseException { } return false; } private boolean parseAction(ProgramNode programNode) { Optional functionNameToken = tokenHandler.getCurrentToken(); if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) { ActionMap actionMap = new ActionMap(); programNode.addNode(actionMap); AcceptSeparators(); return true; } return false; } public VariableReferenceNode ParseLValueVariable(String variableName) { return new VariableReferenceNode(variableName, Optional.empty()); } public VariableReferenceNode ParseLValueArray(String variableName, int index) { return new VariableReferenceNode(variableName, Optional.of(index)); } public OperationNode ParseLValueDollar() { return new OperationNode("$"); } public ConstantNode ParseBottomLevelConstantsAndPatterns() { ConstantNodePatternNode node = new ConstantOrNodePatternNode(); node.setConstant("example"); node.setPattern("PATTERN_1"); return node; } public OperationNode ParseBottomLevelParenthesis() throws ParseException { if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) { OperationNode operationNode = ParseExpression(); if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) { return operationNode; } else { throw new ParseException("Expected ')'", tokenHandler.getCurrentToken().getStart()); } return null; } else { throw new ParseException("Expected '('", tokenHandler.getCurrentToken().getStart()); } } private OperationNode ParsePower() throws ParseException { OperationNode left = ParseBottomLevelParenthesis(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.CARROT); if (op.isEmpty()) { return left; } OperationNode right = ParseBottomLevelParenthesis(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseLogicalAnd() throws ParseException { OperationNode left = ParseComparison(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.AND); if (op.isEmpty()) { return left; } OperationNode right = ParseComparison(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseComparison() throws ParseException { OperationNode left = ParseAdditionOrSubtraction(); while (true) { Optional op = tokenHandler.MatchAndRemove(Token.TokenType.EQUAL) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.NOT_EQUAL)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN_OR_EQUAL)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN)) .or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN_OR_EQUAL)); if (op.isEmpty()) { return left; } OperationNode right = ParseAdditionOrSubtraction(); left = new OperationNode(left, op.get(), right); } } private OperationNode ParseAdditionOrSubtraction() { return null; } private OperationNode ParseLogicalOr() throws ParseException { OperationNode left = ParseLogicalAnd();
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Given is Parser.java. Methods must accept tokens appropriately and generates OperationNode or returns partial result. I need the methods to be created for the following:
-Post Increment/Decrement
-Exponents
-Factor
-Term
-Expression
-Concatenation
-Boolean Compare
-Match
-Array Membership
-And
-Or
-Ternary
-Assignment
Parser.java
import java.text.ParseException;
import java.util.LinkedList;
import java.util.List;
import java.util.Optional;
import javax.swing.ActionMap;
public class Parser {
private TokenHandler tokenHandler;
private LinkedList<Token> tokens;
public Parser(LinkedList<Token> tokens) {
this.tokenHandler = new TokenHandler(tokens);
this.tokens = tokens;
}
public boolean AcceptSeparators() {
boolean foundSeparator = false;
while (tokenHandler.MoreTokens()) {
Optional<Token> currentToken = tokenHandler.getCurrentToken();
if (currentToken.isPresent() && (currentToken.get().getType() == Token.TokenType.NEWLINE || currentToken.get().getType() == Token.TokenType.SEMICOLON)) {
tokenHandler.consumeMatchedToken();
foundSeparator = true;
} else {
break;
}
}
return foundSeparator;
}
public ProgramNode Parse() throws ParseException {
}
return false;
}
private boolean parseAction(ProgramNode programNode) {
Optional<Token> functionNameToken = tokenHandler.getCurrentToken();
if (tokenHandler.MatchAndRemove(Token.TokenType.IDENTIFIER) != null) {
ActionMap actionMap = new ActionMap();
programNode.addNode(actionMap);
AcceptSeparators();
return true;
}
return false;
}
public VariableReferenceNode ParseLValueVariable(String variableName) {
return new VariableReferenceNode(variableName, Optional.empty());
}
public VariableReferenceNode ParseLValueArray(String variableName, int index) {
return new VariableReferenceNode(variableName, Optional.of(index));
}
public OperationNode ParseLValueDollar() {
return new OperationNode("$");
}
public ConstantNode ParseBottomLevelConstantsAndPatterns() {
ConstantNodePatternNode node = new ConstantOrNodePatternNode();
node.setConstant("example");
node.setPattern("PATTERN_1");
return node;
}
public OperationNode ParseBottomLevelParenthesis() throws ParseException {
if (tokenHandler.MatchAndRemove(Token.TokenType.LPAREN) != null) {
OperationNode operationNode = ParseExpression();
if (tokenHandler.MatchAndRemove(Token.TokenType.RPAREN) != null) {
return operationNode;
} else {
throw new ParseException("Expected ')'", tokenHandler.getCurrentToken().getStart());
}
return null;
} else {
throw new ParseException("Expected '('", tokenHandler.getCurrentToken().getStart());
}
}
private OperationNode ParsePower() throws ParseException {
OperationNode left = ParseBottomLevelParenthesis();
while (true) {
Optional<Token> op = tokenHandler.MatchAndRemove(Token.TokenType.CARROT);
if (op.isEmpty()) {
return left;
}
OperationNode right = ParseBottomLevelParenthesis();
left = new OperationNode(left, op.get(), right);
}
}
private OperationNode ParseLogicalAnd() throws ParseException {
OperationNode left = ParseComparison();
while (true) {
Optional<Token> op = tokenHandler.MatchAndRemove(Token.TokenType.AND);
if (op.isEmpty()) {
return left;
}
OperationNode right = ParseComparison();
left = new OperationNode(left, op.get(), right);
}
}
private OperationNode ParseComparison() throws ParseException {
OperationNode left = ParseAdditionOrSubtraction();
while (true) {
Optional<Token> op = tokenHandler.MatchAndRemove(Token.TokenType.EQUAL)
.or(() -> tokenHandler.MatchAndRemove(Token.TokenType.NOT_EQUAL))
.or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN))
.or(() -> tokenHandler.MatchAndRemove(Token.TokenType.LESS_THAN_OR_EQUAL))
.or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN))
.or(() -> tokenHandler.MatchAndRemove(Token.TokenType.GREATER_THAN_OR_EQUAL));
if (op.isEmpty()) {
return left;
}
OperationNode right = ParseAdditionOrSubtraction();
left = new OperationNode(left, op.get(), right);
}
}
private OperationNode ParseAdditionOrSubtraction() {
return null;
}
private OperationNode ParseLogicalOr() throws ParseException {
OperationNode left = ParseLogicalAnd();
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
This is an incomplete code. Where are the implementations for each method you mentioned in the comments? I need the implementation logic (code) for each method you mentioned in the comments. Please include the code implementation logic for each method instead of the comments.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
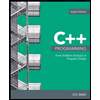
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
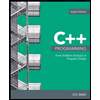
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning