This is a python code Fix these issues: -1 1. Checking: handleCheckMenu: Why check for option 4? It'll never get into the while loop if the choice is 4! -3 2. Savings Menu After any option in savings menu, sends back to main. Why? Should continue displaying savings menu until user selects "Back to Main" option. Interest should be calculated using 2%. Your program uses 20% -3 3. While in checking menu, after deposit, sends back to main. Why? After any option in checking menu, sends back to main. Why? Should continue displaying checking menu until user selects "Back to Main" option. Checking menu: enters infinite loop if user enters 9 for the choice: Checking Menu 1. Deposit 2. Withdrawal 3. Display Checking Balance 4. Return to Main Menu Enter a choice: 9 ================================== def createMenu(listOfChoices, MenuTitle): ct = 1 MenuTitle += '\n' for i in listOfChoices: choice = str(ct) + '. ' + i + '\n' MenuTitle += choice ct += 1 return MenuTitle def getValidChoice(Menu,upperLimit): print(Menu) ch = input("Enter a choice: ") while ch.isdigit() == False or int (ch) < 1 or int (ch) > upperLimit: print('Invalid input') #The message would be false because you entered a less print(Menu) ch = input("Enter a choice: ") return int(ch) def deposit(checkingBalance): amt = int(input("Enter the amount to deposit: ")) checkingBalance = checkingBalance+amt print("Successfully deposited $",amt," to your account.") return checkingBalance def handleCheckMenu(upperLimit,MenuStr,CheckBalance): ch = getValidChoice(MenuStr,upperLimit) while ch != upperLimit: if ch == 1: CheckBalance= deposit (CheckBalance) return CheckBalance elif ch == 2: CheckBalance= withdrawal (CheckBalance) return CheckBalance elif ch == 3: print('Checking Balance is:$ ', CheckBalance) return CheckBalance elif ch == 4: return def handleSaveMenu(upperLimit,MenuStr,SaveBalance): ch = getValidChoice(MenuStr,upperLimit) while ch != upperLimit: if ch == 1: showSavingBalance (SaveBalance) return SaveBalance elif ch == 2: SaveBalance=showInterestEarned (int(SaveBalance)) return SaveBalance elif ch == 3: return SaveBalance #ch = getValidChoice(MenuStr,upperLimit) #return SaveBalance def showInterestEarned(savingBalance): interest = (0.2)*savingBalance savingBalance = savingBalance + (0.2)*savingBalance print("you have earned $ ",interest," as interest") return savingBalance def withdrawal(checkingBalance): #To Withdrawal money from account amt = int(input("Enter the amount to withdraw: $ ")) if (checkingBalance - amt) >=0 : checkingBalance = checkingBalance-amt print("Successfully withdraw $ ",amt," from your account.") else: print("Withdrawal isn't possible, overdraft not allowed") return checkingBalance def showSavingBalance(savingBalance): print("Savings Balance = ",savingBalance) def showcheckingBalance(checkingBalance): print("Savings Balance = ",savingBalance) def main(): mainMenu = createMenu(['Checking Account','Saving Account','Exit'],'Main Menu') checkingMenu = createMenu(['Deposit','Withdrawal','Display Checking Balance','Return to Main Menu'],'Checking Menu') savingMenu = createMenu(['Display Savings Balance','Display Interest Earned',\ 'Return to Main Menu'],'Saving Menu' ) ch = getValidChoice(mainMenu,len(mainMenu)) savingBalance = 100 CheckingBalance = 0 while ch != len(mainMenu): if ch == 1: CheckingBalance=handleCheckMenu(len(checkingMenu),checkingMenu,CheckingBalance) if ch == 2: savingBalance=handleSaveMenu(len(savingMenu),savingMenu,savingBalance) ch = getValidChoice(mainMenu,len(mainMenu)) if ch ==3: return print('Thanks for using this program') if __name__=='__main__': main()
This is a python code
Fix these issues:
-1 1. Checking:
handleCheckMenu:
Why check for option 4? It'll never get into the while loop if the choice is 4!
-3 2. Savings Menu
After any option in savings menu, sends back to main. Why? Should continue displaying savings menu until user selects "Back to Main" option.
Interest should be calculated using 2%. Your program uses 20%
-3 3. While in checking menu, after deposit, sends back to main. Why?
After any option in checking menu, sends back to main. Why? Should continue displaying checking menu until user selects "Back to Main" option.
Checking menu: enters infinite loop if user enters 9 for the choice:
Checking Menu
1. Deposit
2. Withdrawal
3. Display Checking Balance
4. Return to Main Menu
Enter a choice: 9
==================================
def createMenu(listOfChoices, MenuTitle):
ct = 1
MenuTitle += '\n'
for i in listOfChoices:
choice = str(ct) + '. ' + i + '\n'
MenuTitle += choice
ct += 1
return MenuTitle
def getValidChoice(Menu,upperLimit):
print(Menu)
ch = input("Enter a choice: ")
while ch.isdigit() == False or int (ch) < 1 or int (ch) > upperLimit:
print('Invalid input')
#The message would be false because you entered a less
print(Menu)
ch = input("Enter a choice: ")
return int(ch)
def deposit(checkingBalance):
amt = int(input("Enter the amount to deposit: "))
checkingBalance = checkingBalance+amt
print("Successfully deposited $",amt," to your account.")
return checkingBalance
def handleCheckMenu(upperLimit,MenuStr,CheckBalance):
ch = getValidChoice(MenuStr,upperLimit)
while ch != upperLimit:
if ch == 1:
CheckBalance= deposit (CheckBalance)
return CheckBalance
elif ch == 2:
CheckBalance= withdrawal (CheckBalance)
return CheckBalance
elif ch == 3:
print('Checking Balance is:$ ', CheckBalance)
return CheckBalance
elif ch == 4:
return
def handleSaveMenu(upperLimit,MenuStr,SaveBalance):
ch = getValidChoice(MenuStr,upperLimit)
while ch != upperLimit:
if ch == 1:
showSavingBalance (SaveBalance)
return SaveBalance
elif ch == 2:
SaveBalance=showInterestEarned (int(SaveBalance))
return SaveBalance
elif ch == 3:
return SaveBalance
#ch = getValidChoice(MenuStr,upperLimit)
#return SaveBalance
def showInterestEarned(savingBalance):
interest = (0.2)*savingBalance
savingBalance = savingBalance + (0.2)*savingBalance
print("you have earned $ ",interest," as interest")
return savingBalance
def withdrawal(checkingBalance):
#To Withdrawal money from account
amt = int(input("Enter the amount to withdraw: $ "))
if (checkingBalance - amt) >=0 :
checkingBalance = checkingBalance-amt
print("Successfully withdraw $ ",amt," from your account.")
else:
print("Withdrawal isn't possible, overdraft not allowed")
return checkingBalance
def showSavingBalance(savingBalance):
print("Savings Balance = ",savingBalance)
def showcheckingBalance(checkingBalance):
print("Savings Balance = ",savingBalance)
def main():
mainMenu = createMenu(['Checking Account','Saving Account','Exit'],'Main Menu')
checkingMenu = createMenu(['Deposit','Withdrawal','Display Checking Balance','Return to Main Menu'],'Checking Menu')
savingMenu = createMenu(['Display Savings Balance','Display Interest Earned',\
'Return to Main Menu'],'Saving Menu' )
ch = getValidChoice(mainMenu,len(mainMenu))
savingBalance = 100
CheckingBalance = 0
while ch != len(mainMenu):
if ch == 1:
CheckingBalance=handleCheckMenu(len(checkingMenu),checkingMenu,CheckingBalance)
if ch == 2:
savingBalance=handleSaveMenu(len(savingMenu),savingMenu,savingBalance)
ch = getValidChoice(mainMenu,len(mainMenu))
if ch ==3:
return print('Thanks for using this program')
if __name__=='__main__':
main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

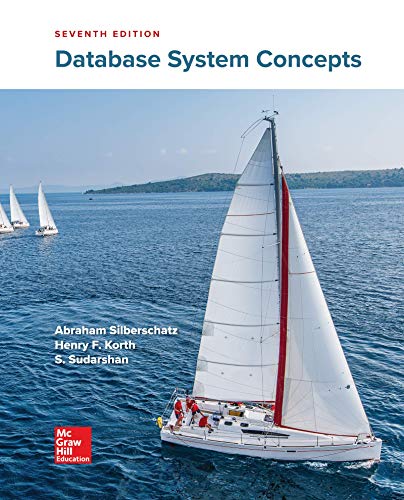
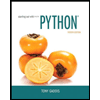
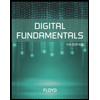
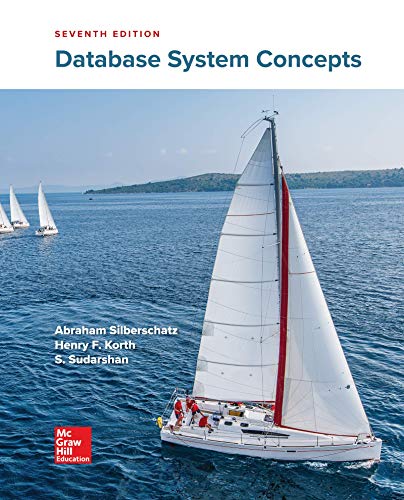
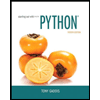
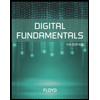
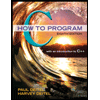
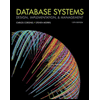
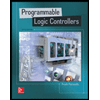