This function takes a list of points and returns the total distance of the path that results from traversing the points as ordered in the list The path should contain at least one point Use the developed functions below to create the function path_distance: 1. Function distance: def distance(d1, d2) : distance = sqrt (((d1 [0] - d2 [0]) **2) + ((d1 [1] - d2 [1]) **2)) return (distance) 2. Function find_closest: def find_closest(start_point, remaining_points): closest_distance = 99999 #declaring the closest distance with maximum value #iterating through all the points of the list for point in remaining_points: dist=distance(start_point,point) if(dist
PYTHON CODING
This function takes a list of points and returns the total distance of the path that results from traversing the points as ordered in the list
The path should contain at least one point
Use the developed functions below to create the function path_distance:
1. Function distance:
def distance(d1, d2) :
distance = sqrt (((d1 [0] - d2 [0]) **2) + ((d1 [1] - d2 [1]) **2))
return (distance)
2. Function find_closest:
def find_closest(start_point, remaining_points):
closest_distance = 99999 #declaring the closest distance with maximum value
#iterating through all the points of the list
for point in remaining_points:
dist=distance(start_point,point)
if(dist<closest_distance): # if the distance is minimum, update the closest_distance and closest_point
closest_distance=dist
closest_point=point
return closest_point
Use the above functions to develop the code for the function below:
def path_distance(path) :
# insert code here
return total_distance
A test case for the path_distance function
path_distance([(5.5,3), (3.5,1.5), (2.5,3.5)])
# the distance from the first point (5.5,3) to the second point (3.5,1.5) is 2.5 and
# the distance from the second point (3.5,1.5) to the third point (2.5,3.5) is 2.23606797749979,
# so the total distance returned should be 4.73606797749979

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

# This function takes a list of points and returns a new list of points,
# beginning with the first point and then at each stage, moving to the closest point that has not yet been visited.
# Note: the function should return a new list, not modify the existing list.
# Hint: use a while loop to repeat while the list of remaining points is not empty
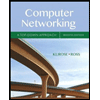
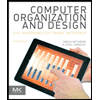
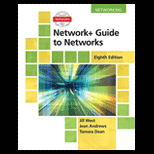
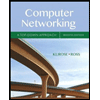
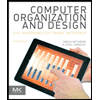
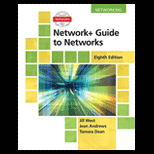
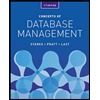
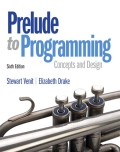
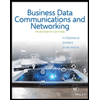