this computer JAVA program that is supposed to give you 3 attempts to insert a password and when you have exceeded that amout, the program ends and when you inser the right password, it's supposed to say access granted. For some reason, when I insert my password, it says that i should try again. Can someone look at my code and see whats the problem? Thank yo
this computer JAVA program that is supposed to give you 3 attempts to insert a password and when you have exceeded that amout, the program ends and when you inser the right password, it's supposed to say access granted. For some reason, when I insert my password, it says that i should try again. Can someone look at my code and see whats the problem? Thank yo
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Hi, I created this computer JAVA
For some reason, when I insert my password, it says that i should try again. Can someone look at my code and see whats the problem? Thank you
![The image shows an online compiler interface with an error message. Here is a detailed transcription and explanation:
### Code Snippet:
```java
public class CellPhone {
String password = "Batman";
}
```
### Error Message:
The compiler outputs the following error:
```
Main.java:33: error: variable purchaser is already defined in method main(String[])
String purchaser;
^
1 error
```
### Explanation:
- **Code Section**: The code snippet defines a simple Java class named `CellPhone` with a single `String` variable called `password`, initialized to "Batman".
- **Error**: The error message indicates an issue in a file named `Main.java` on line 33. It states that the variable `purchaser` is already defined within the `main` method, leading to a compilation failure.
### Interface Overview:
- **Editor Section**: On the left, code can be written and edited.
- **Compile and Run Options**: At the top are options to run, debug, stop, share, save, and beautify the code.
- **Output/Error Pane**: Below the editor is a pane displaying errors and output.
### Graphs/Diagrams:
There are no graphs or diagrams in the image.
### Summary:
This image illustrates a common error encountered during Java programming—defining a variable more than once within the same scope, which causes compilation issues.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7daa69d8-205a-4b72-abf5-96966d755823%2Fb5e876dc-c7be-4da0-ae40-981846e9ac4d%2Fvdmx7p7_processed.png&w=3840&q=75)
Transcribed Image Text:The image shows an online compiler interface with an error message. Here is a detailed transcription and explanation:
### Code Snippet:
```java
public class CellPhone {
String password = "Batman";
}
```
### Error Message:
The compiler outputs the following error:
```
Main.java:33: error: variable purchaser is already defined in method main(String[])
String purchaser;
^
1 error
```
### Explanation:
- **Code Section**: The code snippet defines a simple Java class named `CellPhone` with a single `String` variable called `password`, initialized to "Batman".
- **Error**: The error message indicates an issue in a file named `Main.java` on line 33. It states that the variable `purchaser` is already defined within the `main` method, leading to a compilation failure.
### Interface Overview:
- **Editor Section**: On the left, code can be written and edited.
- **Compile and Run Options**: At the top are options to run, debug, stop, share, save, and beautify the code.
- **Output/Error Pane**: Below the editor is a pane displaying errors and output.
### Graphs/Diagrams:
There are no graphs or diagrams in the image.
### Summary:
This image illustrates a common error encountered during Java programming—defining a variable more than once within the same scope, which causes compilation issues.
![The image displays a Java programming environment in the OnlineGDB compiler. The main code segment includes a class named `Main` with a `main` method. The method aims to validate a password with limited attempts.
Here's a detailed description of the code:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
CellPhone purchaser = new CellPhone();
int multiAttempts = 0;
boolean isPassValid = false;
Scanner keyboard = new Scanner(System.in);
System.out.print("Enter password here: ");
String entPass = keyboard.nextLine();
String password = "";
do {
if (entPass.equals(purchaser.password)) {
System.out.println("Access Granted");
isPassValid = true;
}
if (!isPassValid) {
System.out.println("Error! Try again");
password = keyboard.nextLine();
multiAttempts++;
}
} while (multiAttempts < 3 && !isPassValid);
if (multiAttempts == 3) {
System.out.println("# of attempts exceeded, PROGRAM TERMINATED!!");
System.exit(0);
}
}
}
```
### Key Points:
- **Scanner Usage:** Captures user input for password validation.
- **Conditional Logic:** Verifies the entered password against the stored password, indicating either "Access Granted" or prompting to retry upon failure.
- **Loop and Attempts:** Allows a maximum of three attempts using a `do-while` loop.
- **Exit Condition:** Terminates the program if the maximum attempts are exceeded.
### Error Description:
In the lower console panel, a compilation error is highlighted:
```
Main.java:13: error: variable purchaser is already defined in method main(String[])
String purchaser;
^
1 error
```
This error indicates that there is a redeclaration of the `purchaser` variable in the `main` method, which is causing a conflict with the existing `CellPhone purchaser`.
This error must be resolved for successful compilation by removing or renaming the redundant `purchaser` variable.
### Graphs or Diagrams:
There are no graphs or diagrams in the image.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7daa69d8-205a-4b72-abf5-96966d755823%2Fb5e876dc-c7be-4da0-ae40-981846e9ac4d%2F1zn5a5ce_processed.png&w=3840&q=75)
Transcribed Image Text:The image displays a Java programming environment in the OnlineGDB compiler. The main code segment includes a class named `Main` with a `main` method. The method aims to validate a password with limited attempts.
Here's a detailed description of the code:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
CellPhone purchaser = new CellPhone();
int multiAttempts = 0;
boolean isPassValid = false;
Scanner keyboard = new Scanner(System.in);
System.out.print("Enter password here: ");
String entPass = keyboard.nextLine();
String password = "";
do {
if (entPass.equals(purchaser.password)) {
System.out.println("Access Granted");
isPassValid = true;
}
if (!isPassValid) {
System.out.println("Error! Try again");
password = keyboard.nextLine();
multiAttempts++;
}
} while (multiAttempts < 3 && !isPassValid);
if (multiAttempts == 3) {
System.out.println("# of attempts exceeded, PROGRAM TERMINATED!!");
System.exit(0);
}
}
}
```
### Key Points:
- **Scanner Usage:** Captures user input for password validation.
- **Conditional Logic:** Verifies the entered password against the stored password, indicating either "Access Granted" or prompting to retry upon failure.
- **Loop and Attempts:** Allows a maximum of three attempts using a `do-while` loop.
- **Exit Condition:** Terminates the program if the maximum attempts are exceeded.
### Error Description:
In the lower console panel, a compilation error is highlighted:
```
Main.java:13: error: variable purchaser is already defined in method main(String[])
String purchaser;
^
1 error
```
This error indicates that there is a redeclaration of the `purchaser` variable in the `main` method, which is causing a conflict with the existing `CellPhone purchaser`.
This error must be resolved for successful compilation by removing or renaming the redundant `purchaser` variable.
### Graphs or Diagrams:
There are no graphs or diagrams in the image.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
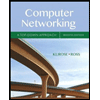
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
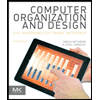
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
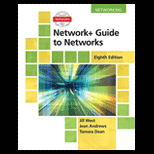
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
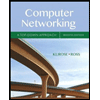
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
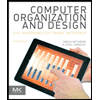
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
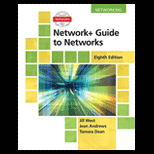
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
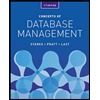
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
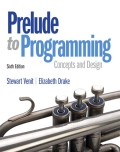
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
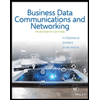
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY