This code needs to be written in C#: If the user guesses the number in 3rd try you will assign: Points = (10 – 3)^2/100 = 7 ^ 2 = 49 points. When the user presses the ‘Start Game’ button, the game will start. User will enter a number in the large textbox and press Submit. If number is correct display the Messagebox, else continue with game. Also tell the user if the number entered was higher or lower than actual number. The problem flow will be as follows: 1. Player enters name and number of chances 2. Player presses Start game 3. Computer generates a secret random number 4. Player enters choice 5. Program checks if number matches guesses number. If number matches jump to step #10 6. Program updates message whether the guessed number is more or less than actual 7. Program reduces available chances by 1 and display messages on changes 8. If chances become 0 stop game and tell use Game has ended, and she will have to press ‘Start Game’ again. Same message should be spoken by the program using text to speech. Note that player gets 0 points if she does not win 9. Repeat steps 4-8 10. If user has guessed the number correctly, display a message box that he won the game and total point accumulated by user based on point system above 11. Using Text to Speech, the program also speaks the message that is displayed Make sure your code follows these guidelines (you will lose points if any guideline is not followed): Display the initial screen with correct layout The game state should be maintained inside a separate class The random number generation should be in separate class The separate class should not have any user interface (MessageBox etc) Correctly compute the points won by the user Code should be well commented and indented Code should compile and run without any error or warnings and should work correctly as per the specifications Code uses exception handling to handle incorrect input from user Text to speech works correctly if user wins or loses
This code needs to be written in C#:
If the user guesses the number in 3rd try you will assign:
Points = (10 – 3)^2/100 = 7 ^ 2 = 49 points.
When the user presses the ‘Start Game’ button, the game will start. User will enter a number in the large
textbox and press Submit. If number is correct display the Messagebox, else continue with game. Also tell
the user if the number entered was higher or lower than actual number.
The problem flow will be as follows:
1. Player enters name and number of chances
2. Player presses Start game
3. Computer generates a secret random number
4. Player enters choice
5.
6. Program updates message whether the guessed number is more or less than actual
7. Program reduces available chances by 1 and display messages on changes
8. If chances become 0 stop game and tell use Game has ended, and she will have to press ‘Start Game’
again. Same message should be spoken by the program using text to speech. Note that player gets 0 points
if she does not win
9. Repeat steps 4-8
10. If user has guessed the number correctly, display a message box that he won the game and total point
accumulated by user based on point system above
11. Using Text to Speech, the program also speaks the message that is displayed
Make sure your code follows these guidelines (you will lose points if any guideline is not followed):
Display the initial screen with correct layout
The game state should be maintained inside a separate class
The random number generation should be in separate class
The separate class should not have any user interface (MessageBox etc)
Correctly compute the points won by the user
Code should be well commented and indented
Code should compile and run without any error or warnings and should work correctly as per
the specifications
Code uses exception handling to handle incorrect input from user
Text to speech works correctly if user wins or loses
![In this assignment, you will create a WinForm-based guessing game. The user will be asked for their name and how many chances they want (up to a maximum of 10). The program will then randomly generate a number between 1 and 30, prompting the user to guess the number within the specified number of tries. If the user guesses correctly, they will be notified and awarded points based on the speed of their guess.
### User Interface Preview
The User Interface (UI) for your program should appear similar to the sample shown:
- **Title**: UHCL Number Guessing Game
- **Fields**:
- **Your Name**: An input field for the user's name.
- **Number of tries**: An input field for the number of tries the user desires.
- **Buttons**:
- **Start Game**: Initiates the guessing game.
- **Game Display**:
- **Enter your guess**: Displays the guessed number.
- **Submit**: Button to submit the user's guess.
- **Feedback and remaining chances**:
- Displays a message indicating if the guessed number is lower or higher than the actual number.
- Shows the number of remaining chances.
### Scoring System
Use the following formula to calculate the points:
\[ \text{Points} = (10 - \text{NumberOfTries})^2 \]
For example, if the user guesses the number on their first try:
\[ \text{Points} = (10 - 1)^2 = 81 \, \text{points} \]
This simple game helps to enhance logical thinking and problem-solving skills by requiring quick and accurate guesses.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F870515b7-c85c-46fe-9e37-44ea51c9af79%2F916067d6-aa82-4c07-b4ec-bf212df0562f%2F6saw3td_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

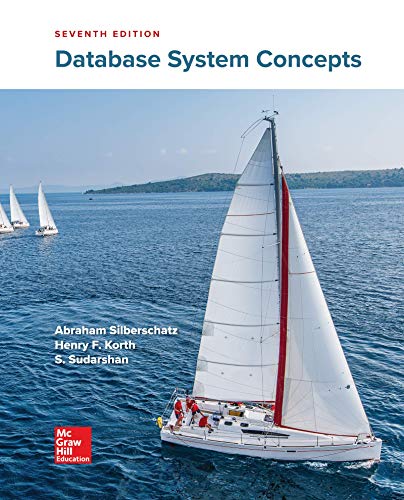
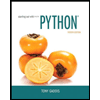
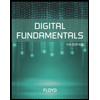
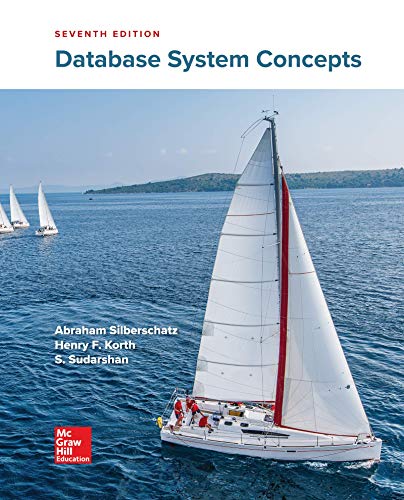
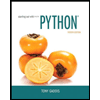
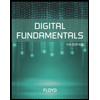
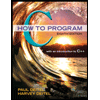
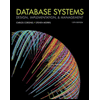
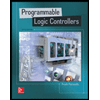