These are my Instructions: "The program is supposed to use a loop to let the user enter positive integers. Your program should keep track of the biggest positive number it has found. Use a loop to continue asking the user to enter a positive number until the user enters the sentinel value (which is -1). Once the user enters -1, that should trigger the loop to stop, and then display the maximum number. The variable userNumber should be used for storing the input the user gives you. The variable maxVal keeps track of the largest integer found so far. In the loop, prompt and store the user's number. Then check, if userNumber is positive and userNumber is greater than maxVal. If it is, then update maxVal to equal userNumber. Repeat this process until userNumber is equal to the SENTINEL variable. You will be creating three different versions of this logic. For the first TODO, try to implement the above logic using a while loop. Once you have that working, comment out your while loop. The second TODO is to try creating a do-while loop that replicates the same logic. Repeat this again for the third TODO (remembering to comment out the previous attempts), but now using a for loop." This is what I have thus far: #include using namespace std; int main() { // variables int maxVal = 0, userNumber = 0; const int SENTINEL = -1; // TODO: create a `while` loop version of finding the maximum number cout << "Enter a number: "; cin >> userNumber; while (userNumber != SENTINEL) { userNumber = ++maxVal; cout << userNumber << endl; } // TODO: create a `do-while` loop version of finding the maximum number do { cout << "Enter a number: "; cin >> userNumber; if(userNumber < -1) cout << "Error, invalid input! Try again!\n"; userNumber++; } while (userNumber < -1); // TODO: create a `for` loop version of finding the maximum number for (userNumber= 0; maxVal <= 200;) { cout << "Enter a number: "; cin >> userNumber; } // display results cout << "\nMaximum number entered: " << maxVal << endl; // terminate return 0; }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
These are my Instructions:
"The program is supposed to use a loop to let the user enter positive integers. Your program should keep track of the biggest positive number it has found. Use a loop to continue asking the user to enter a positive number until the user enters the sentinel value (which is -1). Once the user enters -1, that should trigger the loop to stop, and then display the maximum number.
The variable userNumber should be used for storing the input the user gives you. The variable maxVal keeps track of the largest integer found so far.
In the loop, prompt and store the user's number. Then check, if userNumber is positive and userNumber is greater than maxVal. If it is, then update maxVal to equal userNumber. Repeat this process until userNumber is equal to the SENTINEL variable.
You will be creating three different versions of this logic. For the first TODO, try to implement the above logic using a while loop. Once you have that working, comment out your while loop. The second TODO is to try creating a do-while loop that replicates the same logic. Repeat this again for the third TODO (remembering to comment out the previous attempts), but now using a for loop."
This is what I have thus far:
#include <iostream>
using namespace std;
int main()
{
// variables
int maxVal = 0, userNumber = 0;
const int SENTINEL = -1;
// TODO: create a `while` loop version of finding the maximum number
cout << "Enter a number: ";
cin >> userNumber;
while (userNumber != SENTINEL)
{
userNumber = ++maxVal;
cout << userNumber << endl;
}
// TODO: create a `do-while` loop version of finding the maximum number
do
{
cout << "Enter a number: ";
cin >> userNumber;
if(userNumber < -1)
cout << "Error, invalid input! Try again!\n";
userNumber++;
} while (userNumber < -1);
// TODO: create a `for` loop version of finding the maximum number
for (userNumber= 0; maxVal <= 200;)
{
cout << "Enter a number: ";
cin >> userNumber;
}
// display results
cout << "\nMaximum number entered: " << maxVal << endl;
// terminate
return 0;
}

Code:
#include <iostream>
using namespace std;
int main()
{
//declating required variables
int userNumber,maxVal=0;
int SENTINEL = -1;
//TODO while loop for getting the maximum value
while(true) {
cout << "Enter Any Positive Number : " << std::endl;
cin >> userNumber;
if(userNumber > maxVal) {
maxVal = userNumber;
}
if (userNumber==SENTINEL) {
break;
}
}
//TODO do while loop for getting the maximum value
/* do {
std::cout << "Enter Any Positive Number : " << std::endl;
std::cin >> userNumber;
if(userNumber > maxVal) {
maxVal = userNumber;
}
} while (userNumber != SENTINEL); */
//TODO for loop for getting the maximum value
/* for(;;) {
std::cout << "Enter Any Positive Number : " << std::endl;
std::cin >> userNumber;
if(userNumber > maxVal) {
maxVal = userNumber;
}
if (userNumber==SENTINEL) {
break;
}
} */
//printing the result
std::cout << "maxVal : " << maxVal << std::endl;
return 0;
}
Code Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

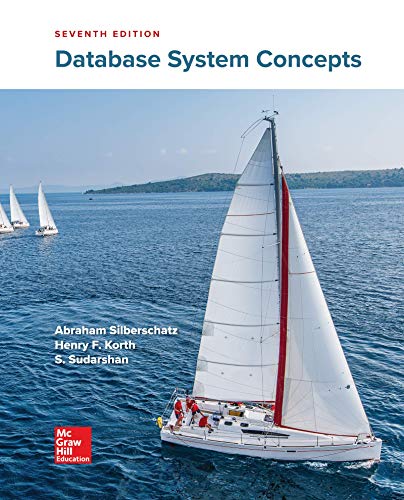
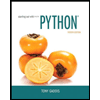
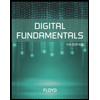
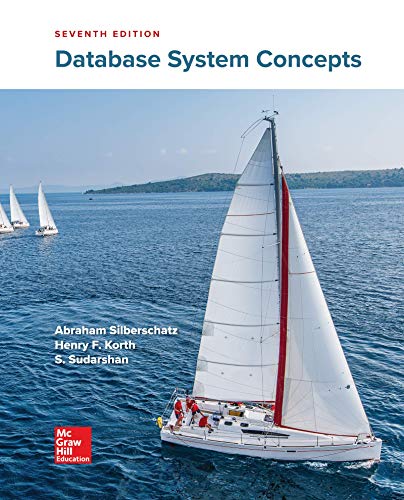
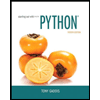
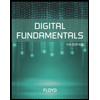
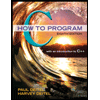
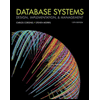
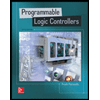