The series below, (four to be precise) of pseudo-code segments that represent typical code and data structures that one might implement with a language such as Java or Python. Devise a strategy to implement these code and data structures using only the Hack assembler. You will be able to use the following assembler codes or symbols to complete this assignment. The following 4 problems are the pseudocode routines that you must implement in the Hack assembler. You should develop the code for your assembler program, compile and test it using the CPU Simulator (Please suggest a link for the CPU Simulator). Kindly show the assembler code by pasting it directly. You should document your code using the // symbol as a comment to describe what part of the pseudo code is being implemented by your assembler code in the assignment so that I can understand how you implemented the code in the assembler. The four problems that you must code include. Comments should not be included in your code when you compile and test it. A couple of tips that might be useful: Comparisons: Your program will need to compare two numbers to determine if one is larger than the other. In assembler, we do not have an ‘if’ expression that allows us to test the equality or inequality of two variables. The way that this capability can be implemented is by subtracting the second number from the first number and then jumping based upon the result. For example, if A and B are our two numbers then the rules for A-B are as follows: If A-B = 0, then A=B. If A-B > 0, then A > B. If A-B < 0, then A < B. If the result of the operation is 0 then we can execute a JEQ which means jump if the output of the operation is equal to zero (see the jump table above). In the algorithm, we need to be able to test if a variable is less than, greater than, or equal to either another variable or a constant value. Each of these tests can be accomplished by subtracting the two numbers and then using the appropriate jump instructions such as: JLT – jump less than 0 JGT – jump greater than 0 JEQ – jump equal to 0 Here, you should develop your solution to the exercise and test it using the CPU emulator.
The series below, (four to be precise) of pseudo-code segments that represent typical code and data structures that one might implement with a language such as Java or Python. Devise a strategy to implement these code and data structures using only the Hack assembler. You will be able to use the following assembler codes or symbols to complete this assignment.
The following 4 problems are the pseudocode routines that you must implement in the Hack assembler. You should develop the code for your assembler program, compile and test it using the CPU Simulator (Please suggest a link for the CPU Simulator). Kindly show the assembler code by pasting it directly. You should document your code using the // symbol as a comment to describe what part of the pseudo code is being implemented by your assembler code in the assignment so that I can understand how you implemented the code in the assembler. The four problems that you must code include. Comments should not be included in your code when you compile and test it.
A couple of tips that might be useful:
Comparisons: Your program will need to compare two numbers to determine if one is larger than the other. In assembler, we do not have an ‘if’ expression that allows us to test the equality or inequality of two variables. The way that this capability can be implemented is by subtracting the second number from the first number and then jumping based upon the result. For example, if A and B are our two numbers then the rules for A-B are as follows:
If A-B = 0, then A=B.
If A-B > 0, then A > B.
If A-B < 0, then A < B.
If the result of the operation is 0 then we can execute a JEQ which means jump if the output of the operation is equal to zero (see the jump table above).
In the
JLT – jump less than 0
JGT – jump greater than 0
JEQ – jump equal to 0
Here, you should develop your solution to the exercise and test it using the CPU emulator.
![(uhen -o)
(when a1)
el
c2
03 c4 e5 06
Cmp emnic
10 1 0
11 1 1
1 1 1 0 10
0 0 1 1 0 0
1 !0 0 . 0
O 0 1 1 1
1 10 0 o 1
0 0 11 1 1
1 10 0 1 1
0 1 1 11 1
1 101 1 1
0 011 10
1 10 0 10
0 0 0 0 10
010 o11
0 0 0 1 1 1
0 00 0 DO
010 LDI
-1
A
ID
IA
IM
-A
D+1
D-1
A-1
N-1
D-A
D-
A-D
M-D
DAA
DlA
In addition you can take advantage of the jump and dest functionality that is represented in the fallowing two tables
j2
(out < 0) (oat =0) (ut >
Mnenie
Elfecet
No jump
If or>0 jump
If at jump
null
If or 20 jump
If ar <0 jump
If at0 jump
If at S0 jump
Jump
1.
JLT
JHE
JLE
INP
Figure 45 The ny fiedd of dhe Citstnxtien. Oat sekes e Ihe ALU output jeesulting from
the inaruction's coxp parn, and ep inples "cotinas evaution uah the instnuction
adressod by the A register."
di d2 43
Macnic
Destiwaniow ( where te store the compwted ralve)
The value is not stored anywhere
MemoryjA] (memory register addressed by A)
D register
Memory(A) and D register
A register
A register and MemoryA)
A register and D register
A register, MemorylAL and D register
null
MD
100
10 1
AM
AD
AMD
Figure 44 The dat field of the C-istruction](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2Fe048415e-2fab-4c27-9025-c7be93f2bf51%2Fvqkuydn_processed.jpeg&w=3840&q=75)
![/ Problem 1
for loap
J=5
forfi=1; i«5: ir+)
/ Problem 2
/- then else
i=4
if i < 5) then
j= 3
else
j= 2
/ Problem 3
/while loap
i = 0
i=0
whileli--0)
j++
if j= 5 then
i=j
/ Problem 4
/ load and traverse an array
A0 =5
A11-4
A2]=3
A31=2
A4=1
ASI-0
for (i=0, ie=5; i++)
if A == 0 then
Alil = 5:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2Fe048415e-2fab-4c27-9025-c7be93f2bf51%2Fzi3ujad_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

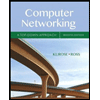
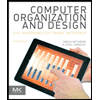
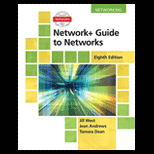
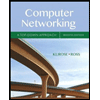
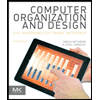
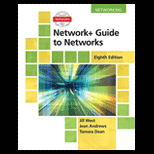
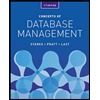
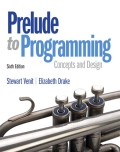
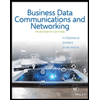