The restoring force of a simple pendulum in the angular (6) direction is F₂ = -mg sin 0, and the corresponding acceleration is a the angular acceleration == -Fe/m = -g sin 0. Dividing ao by the pendulum length L yields g απ = a/L sin L ΦΩ We know from rotational kinematics that angular acceleration is the rate of change of angular velocity, i.e. a = $, and the angular velocity is the rate of change of the angle ǝ, i.e. dt Ω de dt Therefore, our differential equations of motion for the simple pendulum are ΦΩ g sin dt de Ω dt Why do we use a capital greek omega (n) for the angular velocity? Because the lowercase wis defined in the lab manual as the angular frequency of the pendulum, which is a different (constant) quantity: g Iteration is King (again)! The Euler-Cromer method of numerical integration To solve these differential equations, we use (almost) the same iterative Euler method as for the "free fall/air drag" experiment. First, let's define the initial values: We start the pendulum at time to from an angle 00 with zero angular velocity (0 = 0). For a sufficiently short time interval At, the angular acceleration can be considered constant, so we can calculate the new angular velocity at time t₁ = to + At: Ω1 = Ωο + ΦΩ dt At: = No - - sin (00) At Likewise, the angular velocity does not change much during At, so the new angle at time t₁ is 01 = θο + Ω1Δt We repeat these calculations over and over. For the ith step, we have ti = ti−1 + At N₁ = n;-1 - 1-sin(04-1) At L θ; = 0-1 + Ω;ΔΙ 21 Write the code for calculating tį, Si and Oi, based on the equations above. As before, we use a for loop for the repetitive (iterative) calculations. Remember that only indented lines become part of the loop, and all those lines must have the same indentation. • The sin function comes from the numpy library, so call it as np.sin(). • For the time step, use the variable dt defined earlier. • The i-th elements of time, angle and angular velocity can be accessed as t[i], theta[i] and Omega [i], respectively. For element number i - 1, replace i with i-1. [ ] : # Initial conditions. Vector numbering in Python starts at 0. time [0] = = 0 theta [0] = Omega [0] = 0 # initial time np.deg2rad(5.0) # initial angle, converted to radians. # initial angular velocity (pendulum starts at rest) # Main loop: Euler algorithm and evaluation of exact solutions for v and y # # Your code should look like this (without the # signs, of course): # for i in range(1, t_steps): # time[i] Omega[i] = .... = .... # theta[i] = # YOUR CODE HERE raise NotImplementedError()
The restoring force of a simple pendulum in the angular (6) direction is F₂ = -mg sin 0, and the corresponding acceleration is a the angular acceleration == -Fe/m = -g sin 0. Dividing ao by the pendulum length L yields g απ = a/L sin L ΦΩ We know from rotational kinematics that angular acceleration is the rate of change of angular velocity, i.e. a = $, and the angular velocity is the rate of change of the angle ǝ, i.e. dt Ω de dt Therefore, our differential equations of motion for the simple pendulum are ΦΩ g sin dt de Ω dt Why do we use a capital greek omega (n) for the angular velocity? Because the lowercase wis defined in the lab manual as the angular frequency of the pendulum, which is a different (constant) quantity: g Iteration is King (again)! The Euler-Cromer method of numerical integration To solve these differential equations, we use (almost) the same iterative Euler method as for the "free fall/air drag" experiment. First, let's define the initial values: We start the pendulum at time to from an angle 00 with zero angular velocity (0 = 0). For a sufficiently short time interval At, the angular acceleration can be considered constant, so we can calculate the new angular velocity at time t₁ = to + At: Ω1 = Ωο + ΦΩ dt At: = No - - sin (00) At Likewise, the angular velocity does not change much during At, so the new angle at time t₁ is 01 = θο + Ω1Δt We repeat these calculations over and over. For the ith step, we have ti = ti−1 + At N₁ = n;-1 - 1-sin(04-1) At L θ; = 0-1 + Ω;ΔΙ 21 Write the code for calculating tį, Si and Oi, based on the equations above. As before, we use a for loop for the repetitive (iterative) calculations. Remember that only indented lines become part of the loop, and all those lines must have the same indentation. • The sin function comes from the numpy library, so call it as np.sin(). • For the time step, use the variable dt defined earlier. • The i-th elements of time, angle and angular velocity can be accessed as t[i], theta[i] and Omega [i], respectively. For element number i - 1, replace i with i-1. [ ] : # Initial conditions. Vector numbering in Python starts at 0. time [0] = = 0 theta [0] = Omega [0] = 0 # initial time np.deg2rad(5.0) # initial angle, converted to radians. # initial angular velocity (pendulum starts at rest) # Main loop: Euler algorithm and evaluation of exact solutions for v and y # # Your code should look like this (without the # signs, of course): # for i in range(1, t_steps): # time[i] Omega[i] = .... = .... # theta[i] = # YOUR CODE HERE raise NotImplementedError()
Physics for Scientists and Engineers: Foundations and Connections
1st Edition
ISBN:9781133939146
Author:Katz, Debora M.
Publisher:Katz, Debora M.
Chapter16: Oscillations
Section: Chapter Questions
Problem 28PQ: We do not need the analogy in Equation 16.30 to write expressions for the translational displacement...
Question

Transcribed Image Text:The restoring force of a simple pendulum in the angular (6) direction is F₂ = -mg sin 0, and the corresponding acceleration is a
the angular acceleration
==
-Fe/m = -g sin 0. Dividing ao by the pendulum length L yields
g
απ
= a/L
sin
L
ΦΩ
We know from rotational kinematics that angular acceleration is the rate of change of angular velocity, i.e. a
= $, and the angular velocity is the rate of change of the angle ǝ, i.e.
dt
Ω
de
dt
Therefore, our differential equations of motion for the simple pendulum are
ΦΩ
g
sin
dt
de
Ω
dt
Why do we use a capital greek omega (n) for the angular velocity? Because the lowercase wis defined in the lab manual as the angular frequency of the pendulum, which is a different (constant)
quantity:
g
Iteration is King (again)! The Euler-Cromer method of numerical integration
To solve these differential equations, we use (almost) the same iterative Euler method as for the "free fall/air drag" experiment. First, let's define the initial values: We start the pendulum at time to
from an angle 00 with zero angular velocity (0 = 0).
For a sufficiently short time interval At, the angular acceleration can be considered constant, so we can calculate the new angular velocity at time t₁ = to + At:
Ω1 = Ωο +
ΦΩ
dt
At: =
No - - sin (00) At
Likewise, the angular velocity does not change much during At, so the new angle at time t₁ is
01 = θο + Ω1Δt
We repeat these calculations over and over. For the ith step, we have
ti = ti−1 + At
N₁ = n;-1 - 1-sin(04-1) At
L
θ; = 0-1 + Ω;ΔΙ
![21
Write the code for calculating tį, Si and Oi, based on the equations above. As before, we use a for loop for the repetitive (iterative) calculations. Remember that only
indented lines become part of the loop, and all those lines must have the same indentation.
• The sin function comes from the numpy library, so call it as np.sin().
• For the time step, use the variable dt defined earlier.
• The i-th elements of time, angle and angular velocity can be accessed as t[i], theta[i] and Omega [i], respectively. For element number i - 1, replace i
with i-1.
[ ] : # Initial conditions. Vector numbering in Python starts at 0.
time [0] =
= 0
theta [0] =
Omega [0] = 0
# initial time
np.deg2rad(5.0) # initial angle, converted to radians.
# initial angular velocity (pendulum starts at rest)
# Main loop: Euler algorithm and evaluation of exact solutions for v and y
#
# Your code should look like this (without the # signs, of course):
# for i in range(1, t_steps):
#
time[i]
Omega[i]
= ....
=
....
#
theta[i]
=
# YOUR CODE HERE
raise NotImplementedError()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc303f822-5e20-4d30-8920-178170554c96%2F1fe1f649-b0dd-409b-809c-af7d823f5e84%2F7kbijxd_processed.png&w=3840&q=75)
Transcribed Image Text:21
Write the code for calculating tį, Si and Oi, based on the equations above. As before, we use a for loop for the repetitive (iterative) calculations. Remember that only
indented lines become part of the loop, and all those lines must have the same indentation.
• The sin function comes from the numpy library, so call it as np.sin().
• For the time step, use the variable dt defined earlier.
• The i-th elements of time, angle and angular velocity can be accessed as t[i], theta[i] and Omega [i], respectively. For element number i - 1, replace i
with i-1.
[ ] : # Initial conditions. Vector numbering in Python starts at 0.
time [0] =
= 0
theta [0] =
Omega [0] = 0
# initial time
np.deg2rad(5.0) # initial angle, converted to radians.
# initial angular velocity (pendulum starts at rest)
# Main loop: Euler algorithm and evaluation of exact solutions for v and y
#
# Your code should look like this (without the # signs, of course):
# for i in range(1, t_steps):
#
time[i]
Omega[i]
= ....
=
....
#
theta[i]
=
# YOUR CODE HERE
raise NotImplementedError()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
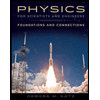
Physics for Scientists and Engineers: Foundations…
Physics
ISBN:
9781133939146
Author:
Katz, Debora M.
Publisher:
Cengage Learning
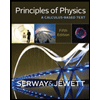
Principles of Physics: A Calculus-Based Text
Physics
ISBN:
9781133104261
Author:
Raymond A. Serway, John W. Jewett
Publisher:
Cengage Learning

University Physics Volume 1
Physics
ISBN:
9781938168277
Author:
William Moebs, Samuel J. Ling, Jeff Sanny
Publisher:
OpenStax - Rice University
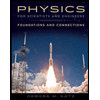
Physics for Scientists and Engineers: Foundations…
Physics
ISBN:
9781133939146
Author:
Katz, Debora M.
Publisher:
Cengage Learning
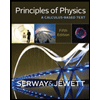
Principles of Physics: A Calculus-Based Text
Physics
ISBN:
9781133104261
Author:
Raymond A. Serway, John W. Jewett
Publisher:
Cengage Learning

University Physics Volume 1
Physics
ISBN:
9781938168277
Author:
William Moebs, Samuel J. Ling, Jeff Sanny
Publisher:
OpenStax - Rice University
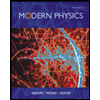
Modern Physics
Physics
ISBN:
9781111794378
Author:
Raymond A. Serway, Clement J. Moses, Curt A. Moyer
Publisher:
Cengage Learning
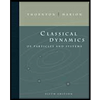
Classical Dynamics of Particles and Systems
Physics
ISBN:
9780534408961
Author:
Stephen T. Thornton, Jerry B. Marion
Publisher:
Cengage Learning
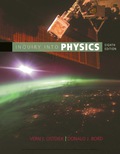