The purpose of this program is to compute the time required to double an investment, and every year after the first year you also include a contribution. I can't seem to figure out how to make my program get the correct output. For example in my code if I set the variable contribution to 100 he output comes out as: Annual contribution: 100 Year: 13 Balance: 20448.20 But the output that I'm supposed to get is: Annual contribution: 100 Year: 13 Balance: 20527.79
The purpose of this program is to compute the time required to double an investment, and every year after the first year you also include a contribution. I can't seem to figure out how to make my program get the correct output. For example in my code if I set the variable contribution to 100 he output comes out as: Annual contribution: 100 Year: 13 Balance: 20448.20 But the output that I'm supposed to get is: Annual contribution: 100 Year: 13 Balance: 20527.79
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
The purpose of this program is to compute the time required to double an investment, and every year after the first year you also include a contribution. I can't seem to figure out how to make my program get the correct output. For example in my code if I set the variable contribution to 100 he output comes out as:
Annual contribution: 100
Year: 13
Balance: 20448.20
But the output that I'm supposed to get is:
Annual contribution: 100
Year: 13
Balance: 20527.79
I think the error in my program is coming from trying to find a way to skip the annual contribution in the first year. Any help will be greatly appreciated.
![### Java Code: Doubling an Investment with Annual Contribution
This Java program calculates the time required to double an initial investment by factoring in an annual contribution. It's an excellent example for understanding loops, conditionals, and basic input in Java programming.
#### Source Code
```java
import java.util.Scanner;
/***
* This program computes the time required to double an investment
* with an annual contribution.
*/
public class DoubleInvestment2 {
public static void main(String[] args) {
final double RATE = 5;
final double INITIAL_BALANCE = 10000;
final double TARGET = 2 * INITIAL_BALANCE;
Scanner in = new Scanner(System.in);
System.out.print("Annual contribution: ");
double contribution = in.nextDouble();
double balance = INITIAL_BALANCE;
int year = 0;
// TODO: Add annual contribution, but not in year 0
while (balance < TARGET) {
balance = balance + (balance * (RATE / 100));
if (year > 0) {
balance = balance + contribution;
}
year++;
}
System.out.println("Year: " + year);
System.out.printf("Balance: %.2f\n", balance);
}
}
```
#### Key Elements
- **Variables:**
- `RATE`: The annual interest rate.
- `INITIAL_BALANCE`: The initial amount of the investment.
- `TARGET`: The goal amount to double the initial investment.
- **User Input:**
- The program prompts the user to enter an "Annual contribution."
- **Logic:**
- The program uses a `while` loop to continuously calculate the new balance each year until it reaches or exceeds the target balance.
- Contributions are added only after the first year (`if (year > 0)`).
- **Output:**
- The program outputs the year when the investment doubles and the exact balance at that time.
This script also includes a `TODO` comment suggesting potential improvements, such as refining how contributions are added.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6e87594-a0d7-48c0-af39-cad58e8108aa%2F40919008-3897-4b3e-a84d-3a26208c8a05%2F72zfrda_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Java Code: Doubling an Investment with Annual Contribution
This Java program calculates the time required to double an initial investment by factoring in an annual contribution. It's an excellent example for understanding loops, conditionals, and basic input in Java programming.
#### Source Code
```java
import java.util.Scanner;
/***
* This program computes the time required to double an investment
* with an annual contribution.
*/
public class DoubleInvestment2 {
public static void main(String[] args) {
final double RATE = 5;
final double INITIAL_BALANCE = 10000;
final double TARGET = 2 * INITIAL_BALANCE;
Scanner in = new Scanner(System.in);
System.out.print("Annual contribution: ");
double contribution = in.nextDouble();
double balance = INITIAL_BALANCE;
int year = 0;
// TODO: Add annual contribution, but not in year 0
while (balance < TARGET) {
balance = balance + (balance * (RATE / 100));
if (year > 0) {
balance = balance + contribution;
}
year++;
}
System.out.println("Year: " + year);
System.out.printf("Balance: %.2f\n", balance);
}
}
```
#### Key Elements
- **Variables:**
- `RATE`: The annual interest rate.
- `INITIAL_BALANCE`: The initial amount of the investment.
- `TARGET`: The goal amount to double the initial investment.
- **User Input:**
- The program prompts the user to enter an "Annual contribution."
- **Logic:**
- The program uses a `while` loop to continuously calculate the new balance each year until it reaches or exceeds the target balance.
- Contributions are added only after the first year (`if (year > 0)`).
- **Output:**
- The program outputs the year when the investment doubles and the exact balance at that time.
This script also includes a `TODO` comment suggesting potential improvements, such as refining how contributions are added.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
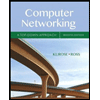
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
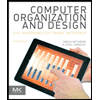
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
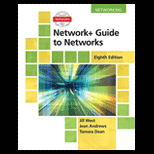
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
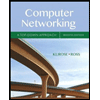
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
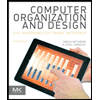
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
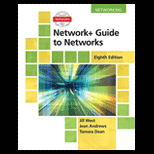
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
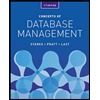
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
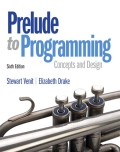
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
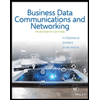
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY