The perimeter P of the rectangle bounded by the two sides L and Wis P=2(L+W) Write a piece of code that implements this equation. In other words, translate the above equation into code. This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and W. Python performs calculations on arrays element-by-element. In [] # YOUR CODE HERE raise Not ImplementedError ()
![The perimeter P of the rectangle bounded by the two sides L and Wis
P = 2(L + W)
Write a piece of code that implements this equation. In other words, translate the above equation into code.
This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and w. Python
performs calculations on arrays element-by-element.
In [ ] # YOUR CODE HERE
raise NotImplementedError()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7f470a0-93f2-43bf-9ed3-2f125d19f075%2F4263acfd-6d2c-4811-81da-bdf7d4d862a2%2Fes8oqat_processed.png&w=3840&q=75)

1. Initialize two empty arrays: lengths and widths to store the dimensions of each rectangle.
2. Prompt the user to enter the number of rectangles (num_rectangles).
3. For each rectangle (i from 1 to num_rectangles):
a. Prompt the user to enter the length of the rectangle and store it in the 'length' variable.
b. Prompt the user to enter the width of the rectangle and store it in the 'width' variable.
c. Append 'length' to the 'lengths' array.
d. Append 'width' to the 'widths' array.
4. Initialize an empty array 'perimeters' to store the calculated perimeters for each rectangle.
5. For each pair of corresponding elements (L and W) from the 'lengths' and 'widths' arrays:
a. Calculate the perimeter P using the formula P = 2 * (L + W).
b. Append the calculated perimeter 'P' to the 'perimeters' array.
6. For each rectangle (i from 1 to num_rectangles):
a. Print the perimeter of rectangle i along with its corresponding value from the 'perimeters' array.
Step by step
Solved in 4 steps with 2 images

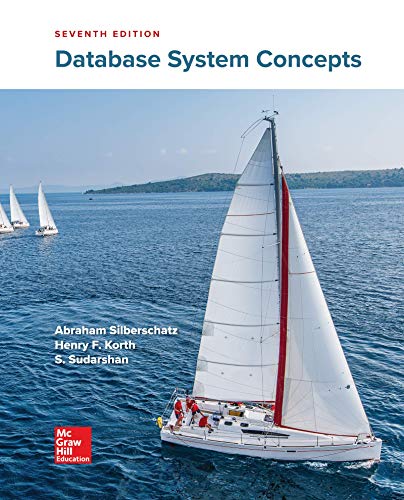
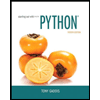
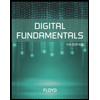
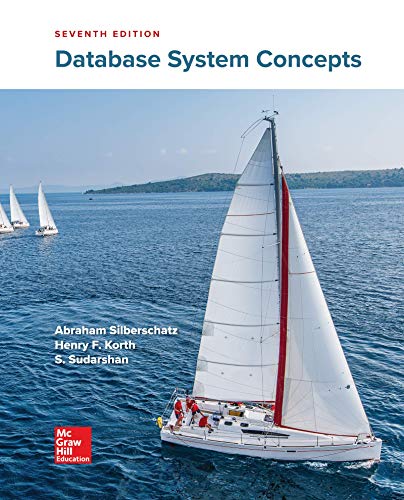
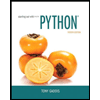
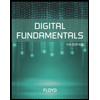
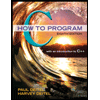
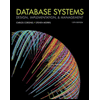
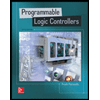