The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an integer. At FIXME in the code, add try and except blocks to catch the ValueError exception and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33 -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34
The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an integer. At FIXME in the code, add try and except blocks to catch the ValueError exception and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33 -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34
Chapter12: Exception Handling
Section: Chapter Questions
Problem 8RQ
Related questions
Question
Need help solving this in Python

Transcribed Image Text:The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an integer. At FIXME in the code, add try and except blocks to catch the ValueError exception and output 0 for the age.
**Example:**
If the input is:
```
Lee 18
Lua 21
Mary Beth 19
Stu 33
-1
```
Then the output is:
```
Lee 19
Lua 22
Mary 0
Stu 34
```
![```python
# Split input into 2 parts: name and age
parts = input().split()
name = parts[0]
while name != '-1':
# FIXME: The following line will throw ValueError exception.
# Insert try/except blocks to catch the exception.
age = int(parts[1]) + 1
print('{} {}'.format(name, age))
# Get next line
parts = input().split()
name = parts[0]
```
### Explanation
This Python script reads input in a loop, expecting each line to contain a name followed by an age. The code attempts to convert the second part of the input (the age) from a string to an integer, increments the age by one, and prints the name and incremented age. The loop continues until the input name is `'-1'`.
#### Important Notes:
- **Input Splitting**: The `input().split()` method is used to split the input line into a list, based on spaces.
- **Potential Error**: The comment indicates that a `ValueError` might occur if the input is not properly formatted (e.g., if the age is not a valid integer). A suggestion is made to use try/except blocks to handle this exception.
- **Looping Mechanism**: The loop will terminate when the input name is `'-1'`.
### Suggested Improvement
To make the code robust, it should include error handling. Here’s an example of how you might add a try/except block:
```python
while name != '-1':
try:
age = int(parts[1]) + 1
print('{} {}'.format(name, age))
except ValueError:
print("Invalid age input. Please enter a valid integer.")
# Get the next line
parts = input().split()
name = parts[0]
```
This ensures that if the conversion of the age fails, a message is printed, and the program continues without termination.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F722c0934-aff7-4600-989e-1d198e6697ab%2F9987aad5-d64d-4040-a24c-748fd3f32e63%2Fdpsts9r_processed.png&w=3840&q=75)
Transcribed Image Text:```python
# Split input into 2 parts: name and age
parts = input().split()
name = parts[0]
while name != '-1':
# FIXME: The following line will throw ValueError exception.
# Insert try/except blocks to catch the exception.
age = int(parts[1]) + 1
print('{} {}'.format(name, age))
# Get next line
parts = input().split()
name = parts[0]
```
### Explanation
This Python script reads input in a loop, expecting each line to contain a name followed by an age. The code attempts to convert the second part of the input (the age) from a string to an integer, increments the age by one, and prints the name and incremented age. The loop continues until the input name is `'-1'`.
#### Important Notes:
- **Input Splitting**: The `input().split()` method is used to split the input line into a list, based on spaces.
- **Potential Error**: The comment indicates that a `ValueError` might occur if the input is not properly formatted (e.g., if the age is not a valid integer). A suggestion is made to use try/except blocks to handle this exception.
- **Looping Mechanism**: The loop will terminate when the input name is `'-1'`.
### Suggested Improvement
To make the code robust, it should include error handling. Here’s an example of how you might add a try/except block:
```python
while name != '-1':
try:
age = int(parts[1]) + 1
print('{} {}'.format(name, age))
except ValueError:
print("Invalid age input. Please enter a valid integer.")
# Get the next line
parts = input().split()
name = parts[0]
```
This ensures that if the conversion of the age fails, a message is printed, and the program continues without termination.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
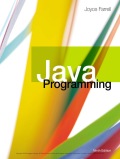
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
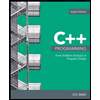
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
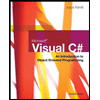
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
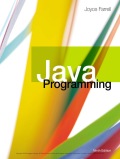
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
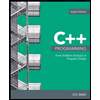
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
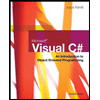
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
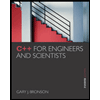
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
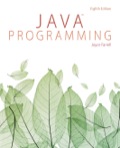
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT