The following program uses Pthreads to create two threads. They do some work for the process and then exit. The process then outputs a result. Assume all supporting libraries and other functions have been included. => Use the answer text field to describe what work (operations) the threads are doing, and what kind of result (what is it?) is output by the process. #include #include #include int res1, res2, a[100], b[100]; void *runner1(void *param); void *runner2(void *param); void readData(int []); int main(int argc, char *argv[]) { pthread_t tid1, tid2; pthread_attr_t attr; readData(a); readData(b); pthread_attr_init(&attr); pthread_create(&tid1, &attr, runner1, argv[1]); pthread_create(&tid2, &attr, runner2, argv[1]); pthread_join(tid1,NULL); pthread_join(tid2,NULL); printf("result = %d\n", res1+res2); } void *runner1(void *param) { int i, upper = atoi(param); res1 = 0; for (i = 0; i < upper; i++) res1 += a[i]; pthread_exit(0); } void *runner2(void *param) { int i, upper = atoi(param); res2 = 0; for (i = 0; i < upper; i++) res2 += b[i]; pthread_exit(0);
The following program uses Pthreads to create two threads. They do some work for the process and then exit. The process then outputs a result.
Assume all supporting libraries and other functions have been included.
=> Use the answer text field to describe what work (operations) the threads are doing, and what kind of result (what is it?) is output by the process.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
int res1, res2, a[100], b[100];
void *runner1(void *param);
void *runner2(void *param);
void readData(int []);
int main(int argc, char *argv[])
{
pthread_t tid1, tid2;
pthread_attr_t attr;
readData(a);
readData(b);
pthread_attr_init(&attr);
pthread_create(&tid1, &attr, runner1, argv[1]);
pthread_create(&tid2, &attr, runner2, argv[1]);
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
printf("result = %d\n", res1+res2);
}
void *runner1(void *param)
{
int i, upper = atoi(param);
res1 = 0;
for (i = 0; i < upper; i++)
res1 += a[i];
pthread_exit(0);
}
void *runner2(void *param)
{
int i, upper = atoi(param);
res2 = 0;
for (i = 0; i < upper; i++)
res2 += b[i];
pthread_exit(0);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

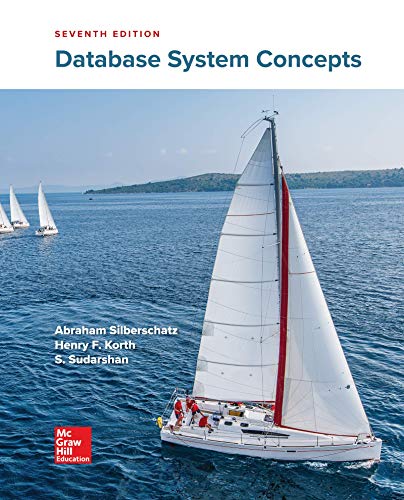
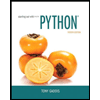
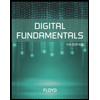
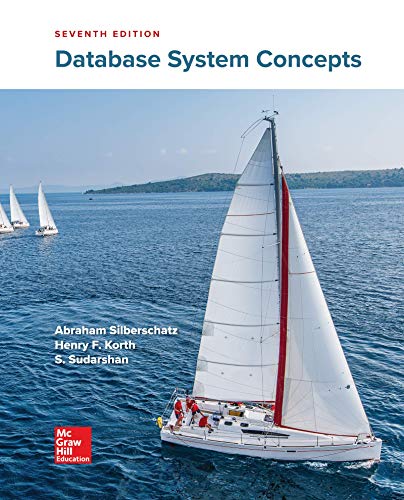
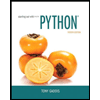
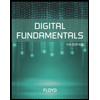
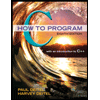
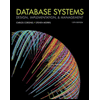
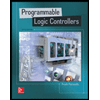