The following program has no syntax problem, but has logical problems. Please correct the member function add(), and make a correct program. The output should be: --------------- From: 10:20:30 After: 15:45:40 Now is: 2:6:10 ------------------ Submit the correct code and the running result. #include using namespace std; class Time { int hour; int minute; int second; public: Time(int x=0, int y=0, int z=0) { hour = x; minute = y; second = z; } int getHour() { return hour; } int getMinute() { return minute; } int getSecond() { return second; } Time add(Time &t); void input() { cin >> hour >> minute >> second; } void output() { cout << hour << ":" << minute << ":" << second << endl; } }; Time Time::add(Time& t) { int newhour, newminute, newsecond; newhour = hour + t.hour; newminute = minute + t.minute; newsecond = second + t.second; Time time(newhour,newminute,newsecond); return time; } int main() { Time myTime(10,20,30), newTime(15,45,40); cout << "From: "; myTime.output(); cout << "After: "; newTime.output(); cout << "Now is: "; Time now = myTime.add(newTime); now.output(); return 0; }
The following
Please correct the member function add(), and make a correct program.
The output should be:
---------------
From: 10:20:30
After: 15:45:40
Now is: 2:6:10
------------------
Submit the correct code and the running result.
#include <iostream>
using namespace std;
class Time {
int hour;
int minute;
int second;
public:
Time(int x=0, int y=0, int z=0)
{
hour = x;
minute = y;
second = z;
}
int getHour() {
return hour;
}
int getMinute() {
return minute;
}
int getSecond() {
return second;
}
Time add(Time &t);
void input()
{
cin >> hour >> minute >> second;
}
void output()
{
cout << hour << ":" << minute << ":" << second << endl;
}
};
Time Time::add(Time& t) {
int newhour, newminute, newsecond;
newhour = hour + t.hour;
newminute = minute + t.minute;
newsecond = second + t.second;
Time time(newhour,newminute,newsecond);
return time;
}
int main() {
Time myTime(10,20,30), newTime(15,45,40);
cout << "From: ";
myTime.output();
cout << "After: ";
newTime.output();
cout << "Now is: ";
Time now = myTime.add(newTime);
now.output();
return 0;
}

Step by step
Solved in 2 steps with 1 images

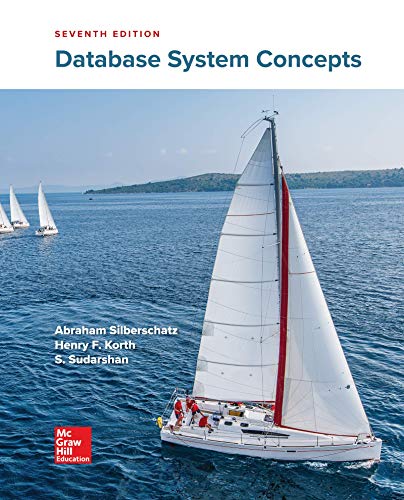
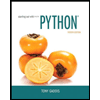
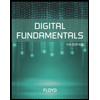
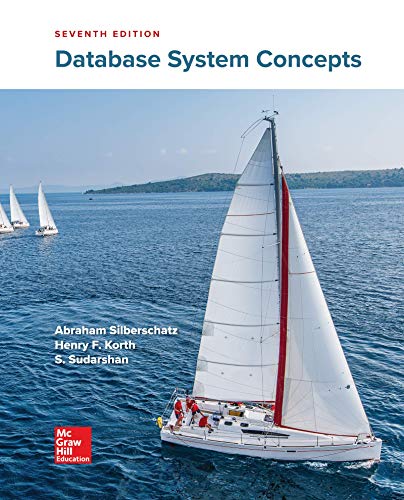
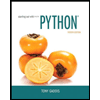
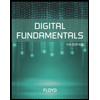
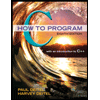
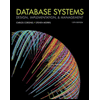
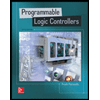