The following is a (silly) decision structure: a,b,c = eval( input('Enter three numbers: ')) if a > b: if b > c: print("Spam Please!") else: print("It's a late pa rrot!") elif b > c: print("Cheese Shoppe") if a >= c: print("Cheddar") elif a < b: print("Gouda") elif c == b: print("Swiss") else: print("Trees") if a == b: print("Chestnut")
The following is a (silly) decision structure: a,b,c = eval( input('Enter three numbers: ')) if a > b: if b > c: print("Spam Please!") else: print("It's a late pa rrot!") elif b > c: print("Cheese Shoppe") if a >= c: print("Cheddar") elif a < b: print("Gouda") elif c == b: print("Swiss") else: print("Trees") if a == b: print("Chestnut")
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### A Silly Decision Structure
The following is a (silly) decision structure:
```python
a, b, c = eval(input('Enter three numbers: '))
if a > b:
if b > c:
print("Spam Please!")
else:
print("It's a late parrot!")
elif b > c:
print("Cheese Shoppe")
if a >= c:
print("Cheddar")
elif a < b:
print("Gouda")
elif c == b:
print("Swiss")
else:
print("Trees")
if a == b:
print("Chestnut")
```
This script begins by prompting the user to enter three numbers, which are then evaluated and stored in variables `a`, `b`, and `c`.
1. **First Condition (`if a > b`):**
- If `a` is greater than `b`, it checks whether `b` is also greater than `c`.
- If `b` is greater than `c`, it prints "Spam Please!".
- Otherwise, it prints "It's a late parrot!"
2. **Second Condition (`elif b > c`):**
- If the first condition is not met and `b` is greater than `c`, it prints "Cheese Shoppe" and proceeds with additional nested conditions:
- If `a` is greater than or equal to `c`, it prints "Cheddar".
- If `a` is less than `b`, it prints "Gouda".
- If `c` is equal to `b`, it prints "Swiss".
3. **Else Condition:**
- If none of the above conditions are met, it prints "Trees".
- It contains an additional nested condition:
- If `a` is equal to `b`, it prints "Chestnut".

Transcribed Image Text:Here is the transcription of the provided text for an educational website:
---
**Programming Logic Example**
```python
print("Trees")
if a == b:
print("Chestnut")
else:
print("Larch")
print("Done")
```
### Task:
Show the output that would result from each of these possible inputs:
**Inputs:** 6, 7, 8
---
**Explanation:**
This snippet of code is a simple Python script that demonstrates the use of conditional statements.
1. It prints "Trees".
2. It then evaluates whether the variable `a` is equal to the variable `b`.
- If `a` is equal to `b`, it prints "Chestnut".
- Otherwise, it prints "Larch".
3. Finally, it prints "Done".
To determine the output for different values of `a` and `b`, you would need to run this script with specific values assigned to `a` and `b`. For this exercise, consider the inputs 6, 7, and 8.
---
Note: This example assumes that the values for `a` and `b` can be provided or set within the script. Therefore, the exact output would depend on what values are assigned to `a` and `b` for each run of the script.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
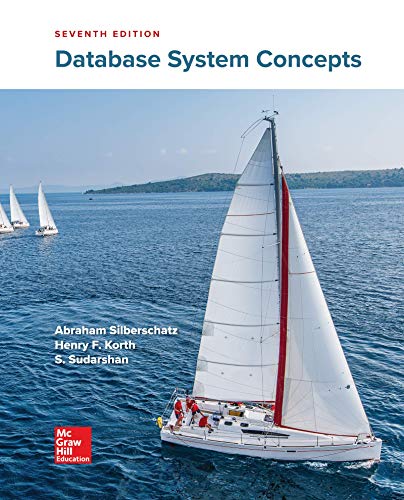
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
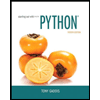
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
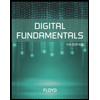
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
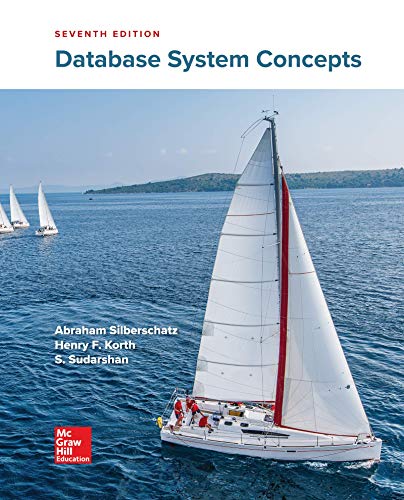
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
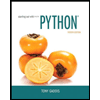
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
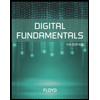
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
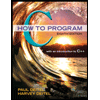
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
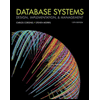
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
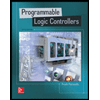
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education